How do I use Java's collections framework effectively?
How to Use Java's Collections Framework Effectively
Effectively using Java's Collections Framework involves understanding its core components and applying best practices for choosing, using, and optimizing collections. The framework provides a rich set of interfaces and classes for storing and manipulating groups of objects. Mastering it requires knowledge of several key aspects:
-
Understanding Interfaces: The framework is built around interfaces like
List
,Set
,Queue
, andMap
. Understanding the characteristics of each is crucial.List
allows duplicates and maintains insertion order;Set
doesn't allow duplicates;Queue
is designed for FIFO (First-In, First-Out) operations; andMap
stores key-value pairs. Choosing the right interface dictates the appropriate implementation. -
Choosing Implementations: Each interface has multiple concrete implementations with varying performance characteristics. For example,
ArrayList
(aList
implementation) provides fast random access but slower insertions and deletions in the middle, whileLinkedList
offers fast insertions and deletions but slower random access.HashSet
is a fast implementation ofSet
using a hash table, whileTreeSet
provides sorted elements but slower operations. Understanding these trade-offs is essential for optimal performance. -
Generics: Using generics
<t></t>
is crucial for type safety and preventing runtimeClassCastException
errors. Declaring the type of objects a collection will hold prevents accidental mixing of different data types. - Iterators and Streams: Iterators provide a standard way to traverse collections. Java 8 introduced streams, which offer a functional approach to processing collections, enabling parallel processing and concise code. Understanding both approaches is important for efficient data manipulation.
- Immutability: When possible, use immutable collections. They prevent accidental modification and enhance thread safety. While not all collections are immutable by default, libraries offer immutable wrappers.
Best Practices for Choosing the Right Collection Type in Java
Choosing the correct collection type depends heavily on the specific requirements of your application. Consider these factors:
-
Data Structure: What kind of data needs to be stored? Do you need to maintain order? Are duplicates allowed? Do you need fast random access, or are insertions/deletions more frequent? These questions help determine whether a
List
,Set
,Queue
, orMap
is appropriate. -
Performance Requirements: Different implementations have different performance characteristics. If random access is crucial,
ArrayList
is a good choice. If frequent insertions and deletions are needed,LinkedList
might be better. For large datasets, consider the memory footprint and performance trade-offs between different implementations. -
Thread Safety: If multiple threads will access the collection concurrently, you need thread-safe implementations like
ConcurrentHashMap
or use synchronization mechanisms to protect mutable collections. -
Null Values: Consider whether your collection will allow null values. Some implementations handle nulls better than others.
HashSet
, for example, allows only one null value. -
Sorting: If you need sorted data,
TreeSet
orTreeMap
are suitable choices. Otherwise,HashSet
orHashMap
are generally faster.
Improving Performance of Java Code by Optimizing Collection Usage
Optimizing collection usage significantly impacts performance. Consider these techniques:
- Choose the Right Implementation: As discussed earlier, selecting the appropriate collection type based on performance requirements is paramount.
- Avoid Unnecessary Iterations: Minimize looping through collections. Use streams for efficient parallel processing when possible.
-
Use Appropriate Data Structures: If you frequently need to check for the presence of an element, a
HashSet
(forSet
operations) orHashMap
(forMap
operations) provides faster lookups (O(1) on average) compared toArrayList
(O(n)). -
Batch Operations: Instead of performing many individual operations, consider batching them together whenever possible. For example, use
addAll()
instead of multipleadd()
calls. -
Efficient Algorithms: Use efficient algorithms for tasks involving collections. For instance, using a binary search on a sorted
List
is much faster than a linear search. - Memory Management: Be mindful of memory usage, especially with large collections. Consider using iterators to avoid loading the entire collection into memory at once. Also, avoid creating unnecessary intermediate collections.
Common Pitfalls to Avoid When Working with Java Collections
Several common mistakes can lead to performance issues or bugs:
- Using the Wrong Collection Type: Choosing an inappropriate collection type based on a misunderstanding of its properties is a major pitfall.
- Ignoring Thread Safety: Concurrent access to mutable collections without proper synchronization can lead to unpredictable results and data corruption.
- Inefficient Iterations: Nested loops and inefficient traversal methods can significantly slow down your code.
- Memory Leaks: Not properly managing collections, especially large ones, can lead to memory leaks if objects are not garbage-collected. Ensure that collections are cleared or set to null when no longer needed.
-
Ignoring Exception Handling: Failing to handle potential exceptions (like
NoSuchElementException
) during collection iteration can lead to program crashes. - Misunderstanding Generics: Improper use of generics can result in runtime type errors. Always specify the generic type explicitly.
By understanding these aspects of Java's Collections Framework and adhering to best practices, you can write efficient, robust, and maintainable Java code.
The above is the detailed content of How do I use Java's collections framework effectively?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


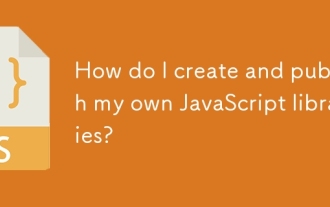
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
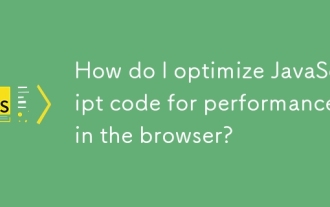
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
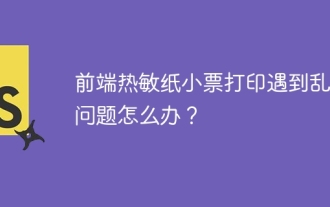
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
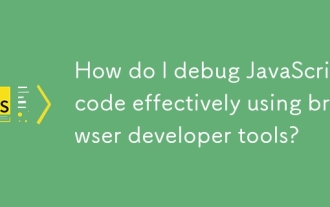
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
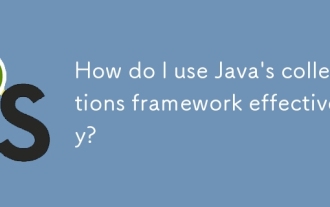
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
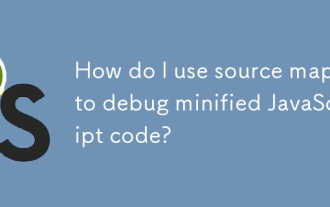
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
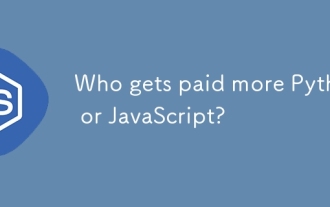
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
