How do I use transactions in MongoDB?
How to Use Transactions in MongoDB?
MongoDB transactions, introduced with version 4.0, provide atomicity, consistency, isolation, and durability (ACID) properties for operations within a single session. They ensure that a set of operations either all succeed or all fail together, preventing partial updates and maintaining data integrity. Transactions are primarily managed using the session
object. Here's a breakdown of how to use them:
1. Initiate a Transaction: You begin a transaction by creating a client session and starting a transaction within that session. This is typically done using the MongoDB driver's capabilities. For example, in the Python driver:
from pymongo import MongoClient, ReadPreference client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase session = client.start_session() with session.start_transaction(): # Perform operations within the transaction result1 = db.collection1.insert_one({"name": "Example"}, session=session) result2 = db.collection2.update_one({"key": "value"}, {"$set": {"field": "updated"}}, session=session) # ... more operations ... session.commit_transaction() # Or session.abort_transaction() if an error occurs client.close()
2. Perform Operations: All operations intended to be part of the transaction must be executed within the with session.start_transaction():
block and explicitly pass the session
object to each operation. This ensures they're all part of the same atomic unit.
3. Commit or Abort: After all operations are completed, you either commit the transaction using session.commit_transaction()
to make the changes permanent or abort the transaction using session.abort_transaction()
to roll back any changes. Error handling is crucial; if any operation within the block fails, the transaction will automatically abort unless explicitly handled otherwise.
What Are the Best Practices for Using MongoDB Transactions?
To maximize the effectiveness and efficiency of MongoDB transactions, follow these best practices:
- Keep Transactions Short: Long-running transactions can negatively impact performance and concurrency. Aim for concise transactions that perform a limited set of operations.
- Use Appropriate Read Concern and Write Concern: Set appropriate read and write concerns for your operations within the transaction to ensure data consistency and durability. The default settings are usually sufficient but consider adjusting them based on your specific needs.
- Error Handling: Implement robust error handling within your transaction block. Catch exceptions, log errors, and handle potential failures gracefully, possibly including retry mechanisms with exponential backoff.
- Avoid Nested Transactions: MongoDB does not support nested transactions. Attempting to start a transaction within an existing transaction will result in an error.
-
Proper Session Management: Ensure that client sessions are properly managed and closed after use to avoid resource leaks. Use context managers (
with
statements) to guarantee cleanup. - Index Optimization: Ensure that appropriate indexes are in place on the collections involved in your transactions to optimize query performance. Inefficient queries can significantly slow down transactions.
Can MongoDB Transactions Handle Multiple Collections?
Yes, MongoDB transactions can span multiple collections within the same database. As shown in the example above, operations on collection1
and collection2
are both part of the same transaction. The key is that all operations within the transaction block must be within the same database. Transactions cannot span multiple databases.
Are There Any Limitations to Using MongoDB Transactions?
While powerful, MongoDB transactions have some limitations:
- Single Database: Transactions are limited to a single database. You cannot perform operations across multiple databases within a single transaction.
- Limited Operation Types: Not all operations are supported within transactions. Certain commands, especially those that involve network operations or external resources, may not be compatible.
- Performance Overhead: Transactions introduce some performance overhead compared to non-transactional operations. The overhead increases with the complexity and duration of the transaction.
- No Support for All Drivers: While major drivers support transactions, ensure your driver version is compatible with transaction support. Older versions may lack this functionality.
- Maximum Transaction Size: There are limits on the size and complexity of transactions. Excessively large transactions can fail due to resource constraints. The specific limits depend on the MongoDB server configuration.
Remember to consult the official MongoDB documentation for the most up-to-date information and best practices related to transactions.
The above is the detailed content of How do I use transactions in MongoDB?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


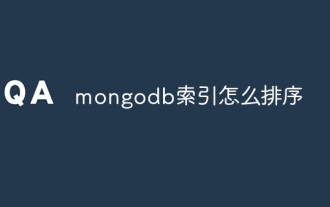
Sorting index is a type of MongoDB index that allows sorting documents in a collection by specific fields. Creating a sort index allows you to quickly sort query results without additional sorting operations. Advantages include quick sorting, override queries, and on-demand sorting. The syntax is db.collection.createIndex({ field: <sort order> }), where <sort order> is 1 (ascending order) or -1 (descending order). You can also create multi-field sorting indexes that sort multiple fields.

MongoDB is more suitable for processing unstructured data and rapid iteration, while Oracle is more suitable for scenarios that require strict data consistency and complex queries. 1.MongoDB's document model is flexible and suitable for handling complex data structures. 2. Oracle's relationship model is strict to ensure data consistency and complex query performance.
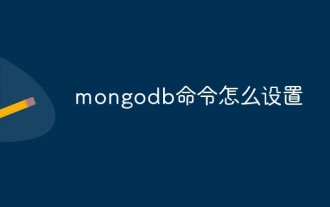
To set up a MongoDB database, you can use the command line (use and db.createCollection()) or the mongo shell (mongo, use and db.createCollection()). Other setting options include viewing database (show dbs), viewing collections (show collections), deleting database (db.dropDatabase()), deleting collections (db.&lt;collection_name&gt;.drop()), inserting documents (db.&lt;collecti

The core strategies of MongoDB performance tuning include: 1) creating and using indexes, 2) optimizing queries, and 3) adjusting hardware configuration. Through these methods, the read and write performance of the database can be significantly improved, response time, and throughput can be improved, thereby optimizing the user experience.

MongoDB is a NoSQL database because of its flexibility and scalability are very important in modern data management. It uses document storage, is suitable for processing large-scale, variable data, and provides powerful query and indexing capabilities.
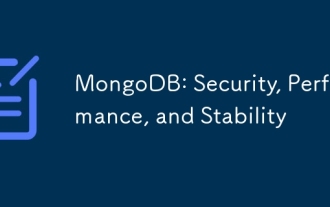
MongoDB excels in security, performance and stability. 1) Security is achieved through authentication, authorization, data encryption and network security. 2) Performance optimization depends on indexing, query optimization and hardware configuration. 3) Stability is guaranteed through data persistence, replication sets and sharding.
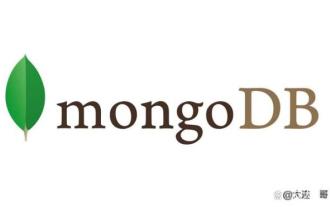
This article explains the advanced MongoDB query skills, the core of which lies in mastering query operators. 1. Use $and, $or, and $not combination conditions; 2. Use $gt, $lt, $gte, and $lte for numerical comparison; 3. $regex is used for regular expression matching; 4. $in and $nin match array elements; 5. $exists determine whether the field exists; 6. $elemMatch query nested documents; 7. Aggregation Pipeline is used for more powerful data processing. Only by proficiently using these operators and techniques and paying attention to index design and performance optimization can you conduct MongoDB data queries efficiently.

The main tools for connecting to MongoDB are: 1. MongoDB Shell, suitable for quickly viewing data and performing simple operations; 2. Programming language drivers (such as PyMongo, MongoDB Java Driver, MongoDB Node.js Driver), suitable for application development, but you need to master the usage methods; 3. GUI tools (such as Robo 3T, Compass) provide a graphical interface for beginners and quick data viewing. When selecting tools, you need to consider application scenarios and technology stacks, and pay attention to connection string configuration, permission management and performance optimization, such as using connection pools and indexes.
