How do you use CSS preprocessors like Sass or Less?
How do you use CSS preprocessors like Sass or Less?
CSS preprocessors like Sass and Less are powerful tools used to enhance the functionality and organization of CSS. They extend the capabilities of vanilla CSS by adding features such as variables, nesting, mixins, and functions, which allow developers to write more maintainable and modular code. Here's how to use them:
-
Installation: To use a CSS preprocessor, you first need to install it on your system. Sass can be installed using Node.js with
npm install -g sass
, while Less can be installed similarly withnpm install -g less
. -
Writing Preprocessor Code: Instead of writing regular CSS, you'll write your styles in Sass or Less syntax. For example, in Sass, you might use:
$primary-color: #333; .button { background-color: $primary-color; }
Copy after loginThis uses a variable
$primary-color
to set the background color of a button. - Compiling to CSS: The preprocessor code you write needs to be compiled into regular CSS that browsers can understand. This is typically done using a command-line tool or a build system. For Sass, you might run
sass input.scss output.css
, and for Less, you could runlessc input.less output.css
. - Integration with Build Tools: Most modern web development projects use build tools like Webpack or Gulp. These tools can be configured to automatically compile your Sass or Less files to CSS whenever you make changes, streamlining your workflow.
- Using in Web Projects: Once your CSS is compiled, you can link the resulting CSS file in your HTML as you would with any regular CSS file.
What are the key benefits of using Sass or Less in web development projects?
Using Sass or Less in web development projects offers several key benefits:
- Variables: Preprocessors allow the use of variables, making it easy to manage repeated values like colors, font sizes, and breakpoints. This makes updating styles across a project much more manageable.
Nesting: You can nest your CSS selectors, which mirrors the structure of HTML and makes the code more readable and maintainable. For example, instead of
.sidebar ul li a
, you can write:.sidebar { ul { li { a { // styles here } } } }
Copy after login- Mixins and Functions: These allow you to define reusable blocks of styles or calculations, reducing code duplication. For instance, you can create a mixin for a button style and use it wherever needed.
- Modularization and Reusability: Preprocessors support the import of other files, allowing you to split your styles into smaller, more manageable files that can be imported where needed, promoting a modular and maintainable codebase.
- Compatibility and Future-proofing: Preprocessors can use features that aren't available in current versions of CSS but may be in future ones, allowing you to adopt modern practices earlier.
How can I set up and start using a CSS preprocessor like Sass or Less in my project?
Setting up a CSS preprocessor in your project involves several steps:
- Install Node.js: Ensure you have Node.js installed, as it's required to run npm commands for installing the preprocessor.
-
Install the Preprocessor:
- For Sass, run
npm install -g sass
in your terminal. - For Less, run
npm install -g less
.
- For Sass, run
-
Create Your Preprocessor Files:
- Create a new file with a
.scss
extension for Sass or.less
extension for Less. This is where you'll write your styles using the preprocessor syntax.
- Create a new file with a
-
Configure Compilation:
- You can compile your files manually from the command line using
sass input.scss output.css
for Sass orlessc input.less output.css
for Less. - For a more automated approach, set up a task runner like Gulp or a module bundler like Webpack to watch and compile your files automatically.
- You can compile your files manually from the command line using
- Link the Compiled CSS: In your HTML, link to the compiled CSS file instead of your preprocessor file.
Which features of Sass or Less can significantly improve my CSS workflow and productivity?
Several features of Sass and Less can dramatically improve your CSS workflow and productivity:
- Variables: By using variables for colors, font sizes, and other common values, you can change a single value to update multiple parts of your stylesheet, greatly reducing the time needed for updates and maintenance.
- Mixins: Mixins allow you to create reusable pieces of code. For example, you can define a mixin for common button styles and use it across your project, reducing repetition and making maintenance easier.
- Nesting: Nesting mirrors the DOM structure in your CSS, which can make your code more intuitive to read and write. It also helps in keeping related styles together.
- Partials and Imports: You can split your styles into multiple files (partials) and import them into a main file. This promotes a modular and organized approach to your stylesheets.
- Functions and Operations: Both Sass and Less allow you to perform operations and use functions, which can help in creating dynamic styles. For instance, you can use functions to adjust color values or perform calculations on sizes and spacing.
-
Extend/Inheritance: Sass's
@extend
and Less's&:extend
allow you to share styles between selectors, reducing redundancy and keeping your CSS DRY (Don't Repeat Yourself).
By leveraging these features, you can significantly enhance your productivity and the maintainability of your CSS codebase.
The above is the detailed content of How do you use CSS preprocessors like Sass or Less?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
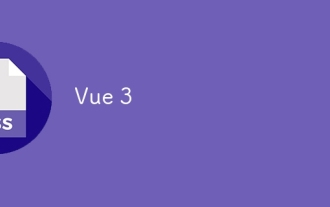
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
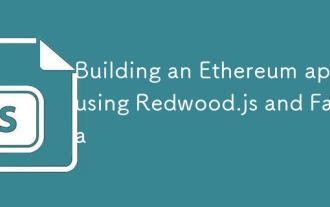
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
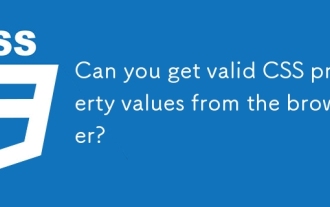
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
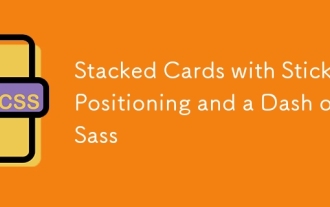
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
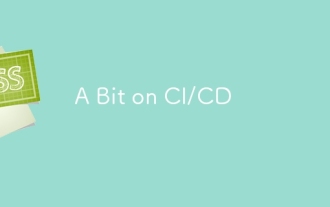
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
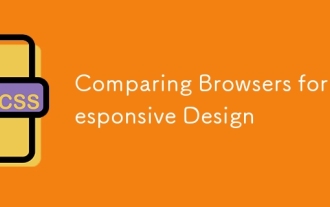
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
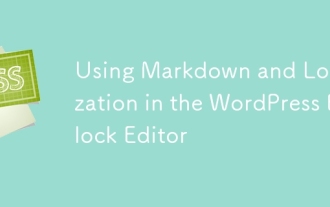
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
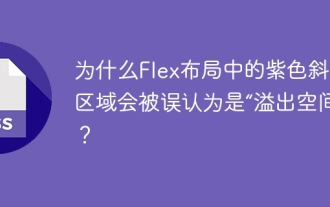
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
