How do I use Web Workers for background processing in HTML5?
How do I use Web Workers for background processing in HTML5?
To use Web Workers for background processing in HTML5, you need to follow these steps:
-
Create a Worker Script: First, create a JavaScript file that will serve as the worker script. This script will contain the code that will run in the background. For instance, save a file named
worker.js
with the following content:self.onmessage = function(event) { // Process the received data let result = processData(event.data); // Send the result back to the main thread self.postMessage(result); }; function processData(data) { // Implement your data processing logic here return data * 2; // Example: doubles the input }
Copy after login Create and Initialize a Worker: In your main JavaScript file (often in an HTML file or a separate .js file), create a new
Worker
object that references your worker script:let worker = new Worker('worker.js');
Copy after loginCommunicate with the Worker: Send messages to the worker using the
postMessage
method, and set up an event listener to receive messages from the worker:// Send a message to the worker worker.postMessage(21); // Receive messages from the worker worker.onmessage = function(event) { console.log('Result: ' event.data); // This should log 42 };
Copy after loginError Handling: Implement error handling to manage any errors that might occur in the worker:
worker.onerror = function(error) { console.error('Worker error: ' error.message); throw error; };
Copy after loginTerminate the Worker: When you're done with the worker, you can terminate it to free up resources:
worker.terminate();
Copy after login
By following these steps, you can leverage Web Workers to offload heavy processing to background threads, enhancing the responsiveness of your web application.
What are the benefits of using Web Workers for improving website performance?
Web Workers provide several benefits for improving website performance:
- Improved Responsiveness: By offloading computationally intensive tasks to Web Workers, the main thread remains free to handle user interactions, keeping your website responsive.
- Parallel Processing: Web Workers allow for parallel execution of scripts, which can significantly speed up operations that can be parallelized. This is particularly beneficial for tasks like data processing, image manipulation, and complex calculations.
- Reduced UI Freezes: Without Web Workers, long-running scripts on the main thread can cause the user interface to freeze. Web Workers prevent this by running such scripts in the background, ensuring the UI remains smooth and interactive.
- Memory Management: Web Workers run in a separate global context, which means they have their own memory space. This helps in better memory management and prevents the main thread from being overloaded.
- Security and Isolation: Since Web Workers run in a separate context, they provide a level of isolation from the main thread. This can be beneficial for security, as it limits the potential impact of malicious scripts.
- Scalability: With Web Workers, you can easily scale up your web application by spawning multiple workers to handle different tasks, enhancing the overall performance and handling capacity of your application.
How can I communicate between the main thread and Web Workers in HTML5?
Communication between the main thread and Web Workers in HTML5 is facilitated through message passing using the postMessage
method and event listeners. Here's how it works:
Sending Messages from the Main Thread to a Worker:
In the main thread, you use the
postMessage
method on theWorker
object to send data:let worker = new Worker('worker.js'); worker.postMessage({ type: 'calculate', value: 42 });
Copy after loginReceiving Messages in the Worker:
In the worker script, you set up an event listener for messages:
self.onmessage = function(event) { if (event.data.type === 'calculate') { let result = event.data.value * 2; self.postMessage({ type: 'result', value: result }); } };
Copy after loginSending Messages from the Worker to the Main Thread:
Within the worker, use
self.postMessage
to send data back to the main thread:self.postMessage({ type: 'result', value: result });
Copy after loginReceiving Messages in the Main Thread:
In the main thread, set up an event listener to receive messages from the worker:
worker.onmessage = function(event) { if (event.data.type === 'result') { console.log('Calculation result: ' event.data.value); } };
Copy after loginError Handling:
Both the main thread and the worker can handle errors:
Main thread:
worker.onerror = function(error) { console.error('Worker error: ' error.message); };
Copy after loginWorker script:
self.onerror = function(error) { self.postMessage({ type: 'error', message: error.message }); };
Copy after login
This bidirectional communication enables efficient data exchange and task management between the main thread and Web Workers.
What are some common pitfalls to avoid when implementing Web Workers in my web application?
When implementing Web Workers, it's important to be aware of and avoid these common pitfalls:
-
Accessing DOM from Workers: Web Workers do not have access to the DOM, the
window
object, or most of the JavaScript APIs that are available to the main thread. Attempting to access these from within a worker will result in errors. - Overusing Web Workers: While Web Workers can improve performance, spawning too many can lead to increased overhead and memory usage, potentially slowing down your application. Use them judiciously and only for tasks that truly benefit from background processing.
- Inefficient Communication: Excessive message passing between the main thread and workers can lead to performance issues. Try to minimize the frequency of messages and use efficient data structures for communication.
- Not Handling Worker Errors: Failing to implement proper error handling can lead to silent failures, making debugging more difficult. Always implement error handling both in the main thread and within the worker script.
- Ignoring Worker Lifecycle: Not properly managing the lifecycle of Web Workers (e.g., forgetting to terminate unused workers) can lead to resource leaks. Always terminate workers when they are no longer needed.
- Synchronous vs. Asynchronous Confusion: Remember that communication with Web Workers is asynchronous. Code that depends on worker results should account for this, possibly using callbacks or promises to handle the asynchronous nature of responses.
- Security Concerns: Since Web Workers run in their own context, ensure that the code loaded into workers is trusted and secure. Malicious scripts in a worker can pose security risks, albeit limited to their own context.
By being mindful of these pitfalls, you can more effectively implement Web Workers and avoid common issues that could undermine your application's performance and reliability.
The above is the detailed content of How do I use Web Workers for background processing in HTML5?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.

AnexampleofastartingtaginHTMLis,whichbeginsaparagraph.StartingtagsareessentialinHTMLastheyinitiateelements,definetheirtypes,andarecrucialforstructuringwebpagesandconstructingtheDOM.
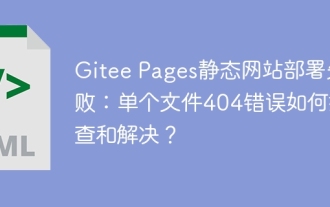
GiteePages static website deployment failed: 404 error troubleshooting and resolution when using Gitee...
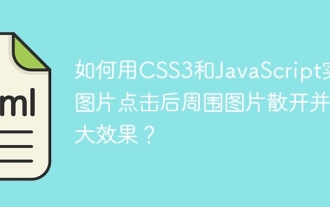
To achieve the effect of scattering and enlarging the surrounding images after clicking on the image, many web designs need to achieve an interactive effect: click on a certain image to make the surrounding...
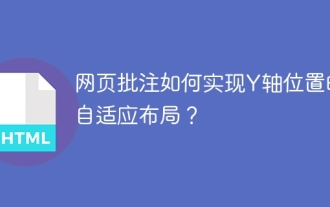
The Y-axis position adaptive algorithm for web annotation function This article will explore how to implement annotation functions similar to Word documents, especially how to deal with the interval between annotations...

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.
