


How do I use Server-Sent Events (SSE) for real-time updates in HTML5?
How do I use Server-Sent Events (SSE) for real-time updates in HTML5?
To use Server-Sent Events (SSE) for real-time updates in HTML5, you need to set up both the client-side and server-side components. Here’s a step-by-step guide:
Client-side Setup:
-
Creating an EventSource:
In your HTML file, you create anEventSource
object that connects to a URL on your server. This URL should be the endpoint that will be sending the events.<script> var source = new EventSource('/events'); </script>
Copy after login Listening for Events:
You can listen for different types of events such asmessage
,open
, anderror
. For example, to handle incoming messages:<script> source.onmessage = function(event) { console.log('New message:', event.data); // Handle the event data }; </script>
Copy after loginCustom Events:
If your server sends custom events, you can listen to them usingaddEventListener
:<script> source.addEventListener('customEvent', function(event) { console.log('Custom event:', event.data); }, false); </script>
Copy after login
Server-side Setup:
Setting up the Server:
Your server needs to respond with the appropriate headers and format the data correctly. For example, in Node.js using Express:app.get('/events', function(req, res) { res.writeHead(200, { 'Content-Type': 'text/event-stream', 'Cache-Control': 'no-cache', 'Connection': 'keep-alive' }); setInterval(function() { res.write('data: ' new Date().toLocaleTimeString() '\n\n'); }, 1000); });
Copy after login- Sending Data:
Data must be sent in the correct format, starting withdata:
, followed by the data, and ending with two newline characters (\n\n
).
Using these steps, you can implement SSE in your web application to push real-time updates to the client.
What are the advantages of using SSE over other real-time technologies like WebSockets?
SSE offers several advantages over other real-time technologies like WebSockets, including:
- Simpler to Implement:
SSE is generally easier to set up and requires less overhead compared to WebSockets. It uses HTTP, which makes it easier to integrate with existing web infrastructure. - Automatic Reconnection:
SSE connections automatically attempt to reconnect if they are interrupted, reducing the need for additional logic to manage connections. - Server-to-Client Only:
SSE is unidirectional, sending data only from server to client. This can be beneficial if your application only requires server-to-client communication, as it simplifies the server logic. - HTTP Friendly:
SSE works over HTTP, making it easier to scale and fit into existing HTTP-based systems like proxies and load balancers. - Event Types:
SSE allows for named events, making it easier to categorize different types of updates on the client-side.
However, SSE may not be suitable for applications that require bidirectional communication, which is a key advantage of WebSockets.
How can I handle errors and disconnections when using SSE in my web application?
Handling errors and disconnections in SSE is crucial for maintaining a robust web application. Here are some strategies:
Listening for Error Events:
You can handle errors by listening to theerror
event:<script> source.onerror = function() { console.log('An error occurred while attempting to connect to the server.'); // Handle error, perhaps by attempting to reconnect }; </script>
Copy after loginReconnection Logic:
TheEventSource
object will automatically try to reconnect if the connection is lost, but you might want to add custom logic:<script> var attempt = 0; source.onerror = function() { if (attempt < 3) { setTimeout(function() { source = new EventSource('/events'); attempt ; }, 1000); } else { console.log('Failed to reconnect after several attempts.'); } }; </script>
Copy after login-
Server-side Handling:
On the server-side, ensure that you handle long-lived connections properly and manage resources efficiently to prevent server overload. -
User Feedback:
Provide user feedback when connections are lost and being re-established to keep the user informed about the application's status.
Implementing these strategies will help you handle errors and disconnections more gracefully in your SSE-based applications.
What steps should I take to ensure browser compatibility when implementing SSE?
Ensuring browser compatibility when implementing SSE involves several steps:
-
Check Browser Support:
SSE is supported by most modern browsers, including Chrome, Firefox, Safari, and Edge. However, you should check the specific version of each browser to ensure support. -
Polyfills and Fallbacks:
For older browsers that do not support SSE natively, consider using polyfills or fallbacks. Libraries likeEventSource polyfill
can help extend SSE functionality to non-supporting browsers. -
Fallback Mechanism:
Implement a fallback mechanism for browsers without SSE support. You could use polling or another real-time technology like WebSockets as a fallback. -
Testing Across Browsers:
Thoroughly test your implementation across different browsers and versions to ensure consistent behavior. Use tools like BrowserStack or Sauce Labs for cross-browser testing. -
Graceful Degradation:
Design your application to degrade gracefully if SSE is not available. Provide alternative ways for users to receive updates if real-time updates via SSE fail. -
Server-Side Compatibility:
Ensure your server-side code is compatible with the SSE protocol, and test it against different client implementations.
By following these steps, you can maximize the compatibility of your SSE implementation across various browsers and user environments.
The above is the detailed content of How do I use Server-Sent Events (SSE) for real-time updates in HTML5?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.

AnexampleofastartingtaginHTMLis,whichbeginsaparagraph.StartingtagsareessentialinHTMLastheyinitiateelements,definetheirtypes,andarecrucialforstructuringwebpagesandconstructingtheDOM.
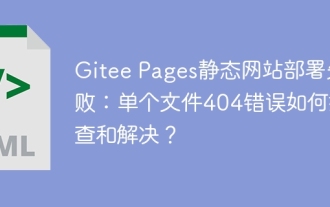
GiteePages static website deployment failed: 404 error troubleshooting and resolution when using Gitee...
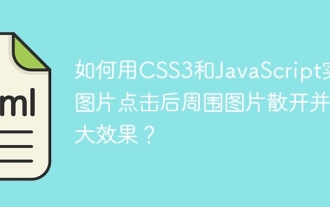
To achieve the effect of scattering and enlarging the surrounding images after clicking on the image, many web designs need to achieve an interactive effect: click on a certain image to make the surrounding...
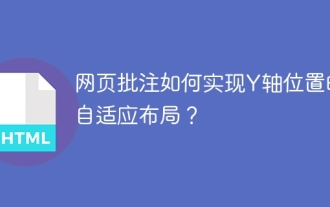
The Y-axis position adaptive algorithm for web annotation function This article will explore how to implement annotation functions similar to Word documents, especially how to deal with the interval between annotations...

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.
