


What are the different ways to store data in the browser using JavaScript (cookies, localStorage, sessionStorage, IndexedDB)?
What are the different ways to store data in the browser using JavaScript (cookies, localStorage, sessionStorage, IndexedDB)?
When developing web applications, it's crucial to know how to manage data efficiently on the client side. JavaScript offers several methods for storing data in the browser, each with its unique features and use cases. Let's explore each of these methods in detail:
-
Cookies:
- Cookies are small pieces of data that a website stores on a user's computer. They are sent with every HTTP request to the originating server, making them useful for session management and tracking user preferences.
- Cookies are typically limited to about 4KB in size and can be set to expire at a specific date or after a session ends.
- They are accessible via JavaScript using the
document.cookie
API.
-
localStorage:
-
localStorage
is part of the Web Storage API and allows storing key-value pairs in a user's browser. The data stored here persists even after the browser is closed. - It has a larger storage capacity than cookies, typically up to 10MB, though this varies between browsers.
-
localStorage
data is accessible via JavaScript usinglocalStorage.setItem()
andlocalStorage.getItem()
methods, and it's scoped to the domain.
-
-
sessionStorage:
- Similar to
localStorage
,sessionStorage
is part of the Web Storage API but stores data for a single session. Once the browser tab is closed, the data is lost. - It also has a capacity of up to 10MB and is accessed via JavaScript using
sessionStorage.setItem()
andsessionStorage.getItem()
methods. -
sessionStorage
is also domain-specific but session-specific.
- Similar to
-
IndexedDB:
- IndexedDB is a low-level API for client-side storage of structured data, including files/blobs. It provides more complex storage capabilities than the Web Storage API.
- It's capable of handling large amounts of data and supports indexing and querying, making it suitable for more advanced applications.
- IndexedDB is asynchronous and accessed via JavaScript using various methods like
open()
,createObjectStore()
,transaction()
, etc.
How does each method of data storage in JavaScript affect website performance?
The performance impact of data storage methods in JavaScript depends on several factors such as the size and frequency of data access, as well as the nature of the data being stored. Here’s how each method affects performance:
-
Cookies:
- Cookies can negatively impact performance because they are sent with every HTTP request, adding to the payload. This can slow down page load times, especially for websites with many cookies.
- Frequent setting and retrieving of cookies using JavaScript can also add to the computational overhead.
-
localStorage:
-
localStorage
is synchronous and can cause performance issues if used to store and retrieve large amounts of data frequently. For example, if a large dataset is being manipulated repeatedly, it can block the main thread. - However, for small amounts of data, it’s efficient and doesn’t affect performance as much as cookies.
-
-
sessionStorage:
- Similar to
localStorage
,sessionStorage
is synchronous and can have performance issues with large data operations, though the impact is limited to the duration of the session. - It's efficient for small to medium-sized data that needs to be accessed only within a single session.
- Similar to
-
IndexedDB:
- IndexedDB is designed to handle large datasets efficiently and is asynchronous, which means it doesn’t block the main thread. This makes it more suitable for applications that require managing large amounts of data without impacting performance.
- The initial setup of IndexedDB can be complex and might have a slight performance overhead, but its efficiency in handling large data makes it a preferable choice for heavy data applications.
What are the security implications of using different JavaScript data storage methods in the browser?
Security is a critical aspect to consider when choosing a method for data storage in browsers. Here are the security implications of each method:
-
Cookies:
- Cookies are vulnerable to cross-site scripting (XSS) and cross-site request forgery (CSRF) attacks. Sensitive data in cookies can be stolen or manipulated if not secured properly.
- Cookies can be set with the
Secure
andHttpOnly
flags to enhance security, limiting their exposure to client-side scripts and ensuring they are sent over HTTPS.
-
localStorage:
- Similar to cookies,
localStorage
is vulnerable to XSS attacks, as any script running on the same domain can access and manipulate the stored data. - It does not have built-in security features like
Secure
orHttpOnly
, so extra caution is needed when storing sensitive information.
- Similar to cookies,
-
sessionStorage:
-
sessionStorage
has the same security vulnerabilities aslocalStorage
, but the data is lost when the tab is closed, which can limit the exposure of sensitive data. - It's less vulnerable to attacks that persist across sessions, but still requires careful handling of sensitive information.
-
-
IndexedDB:
- IndexedDB is also susceptible to XSS attacks. Any script on the same domain can access the database, which necessitates careful management of data permissions.
- It allows for more granular control over data access through the use of transactions and keys, which can be used to implement more secure data handling practices.
Which JavaScript data storage method is best suited for storing large amounts of data in the browser?
For storing large amounts of data in the browser, IndexedDB is the most suitable method. Here's why:
-
Capacity: IndexedDB can handle much larger amounts of data compared to cookies,
localStorage
, andsessionStorage
. It's designed to store significant volumes of structured data, making it ideal for applications that need to manage large datasets. - Performance: IndexedDB is asynchronous, meaning it doesn't block the main thread of the browser. This is crucial for applications dealing with large datasets, as it allows other tasks to continue running while data operations are being processed.
- Functionality: It offers advanced features such as indexing and querying, which are essential for efficiently managing and retrieving large datasets. This makes it suitable for applications that require complex data management.
-
Persistence: Data stored in IndexedDB persists even after the browser is closed, similar to
localStorage
, but with the added benefit of handling more structured and larger data.
In conclusion, while cookies, localStorage
, and sessionStorage
have their place for specific use cases, IndexedDB is the best choice for applications that need to store and manage large amounts of data in the browser.
The above is the detailed content of What are the different ways to store data in the browser using JavaScript (cookies, localStorage, sessionStorage, IndexedDB)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
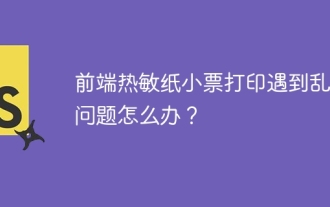
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
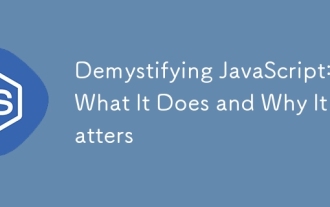
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
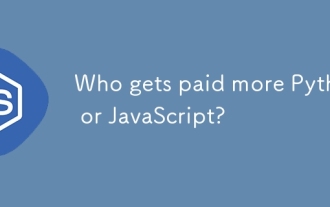
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
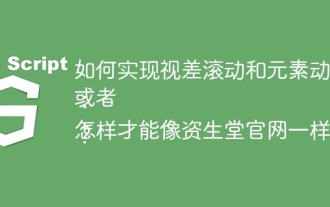
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
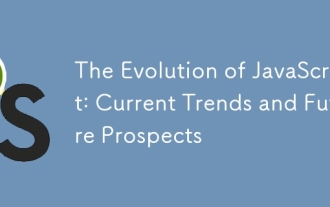
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
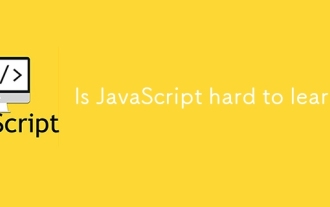
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
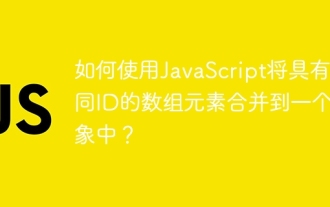
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
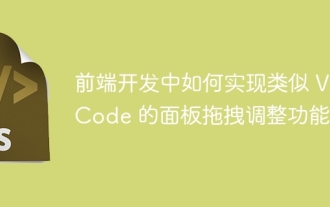
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
