How do I create custom exception classes in C ?
How do I create custom exception classes in C ?
To create custom exception classes in C that can be used to provide detailed and specific error information, follow these steps:
-
Inherit from
std::exception
: The standard C library provides a base class calledstd::exception
. By inheriting from this class, your custom exception class will have a standard interface. - Define the Custom Exception Class: You can define your custom exception class with additional information relevant to your application. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
- Add Additional Members and Methods: You can add any additional members and methods that are needed to store and retrieve information about the error. For instance:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
This FileException
class can store the filename and error code associated with a file operation failure, allowing more detailed error reporting and handling.
What are the benefits of using custom exception classes in C ?
Using custom exception classes in C can provide several significant benefits:
- Improved Error Handling: Custom exception classes allow you to encapsulate specific error information related to your application's domain. This enables more precise error handling, which can lead to better error recovery and debugging.
- Enhanced Code Readability and Maintainability: By creating custom exception classes, you make your code more readable and easier to maintain. Developers can quickly understand the types of errors that may occur and how to handle them without wading through generic error messages.
- Consistent Error Reporting: Custom exceptions can standardize error reporting across your application. By using a set of custom exception classes, you ensure that error handling is consistent, which simplifies the debugging process and makes your application more reliable.
- Hierarchical Exception Handling: You can create a hierarchy of custom exceptions, which allows you to catch and handle exceptions at different levels of specificity. This can be particularly useful for large applications where certain errors might need to be handled differently based on the context.
- Integration with Standard Library: Since custom exceptions inherit from
std::exception
, they can be seamlessly integrated with the standard library’s exception handling mechanisms, making them compatible with existing code and libraries.
How can I handle custom exceptions effectively in C ?
Effective handling of custom exceptions in C involves several best practices and techniques:
- Use Try-Catch Blocks: Enclose code that may throw custom exceptions within
try
blocks, and usecatch
blocks to handle those exceptions appropriately. For example:
1 2 3 4 5 6 7 8 9 10 |
|
- Catch Specific Exceptions First: It is good practice to catch more specific exceptions before more general ones. This ensures that you handle the most relevant exception without accidentally catching a less specific one.
- Use Exception Hierarchies: If you have a hierarchy of custom exceptions, catch the more derived types before the more base types. This allows you to handle specific cases before falling back to more general error handling.
- Proper Cleanup: Ensure that resources are properly released in case an exception is thrown. Use RAII (Resource Acquisition Is Initialization) techniques, such as smart pointers, to manage resources and prevent memory leaks.
- Logging and Diagnostics: When catching exceptions, log detailed information about the error, including the custom exception's data members. This can help in debugging and maintaining your application.
- User-Friendly Error Messages: Translate exception information into user-friendly error messages when appropriate. Custom exceptions can contain detailed internal error information while the user interface can display a more understandable error message.
-
Avoid Catch-All: Be cautious with catch-all blocks (
catch (...)
). While they can be useful in some scenarios, they can also mask errors. Always prefer catching specific exceptions when possible.
By following these practices, you can handle custom exceptions effectively, leading to more robust and reliable C applications.
The above is the detailed content of How do I create custom exception classes in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
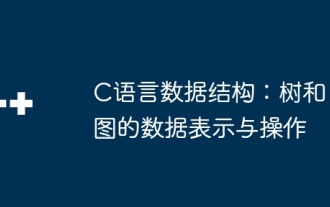
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
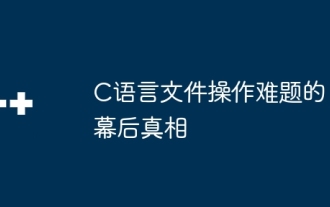
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
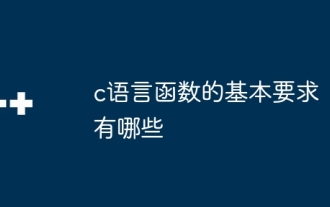
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
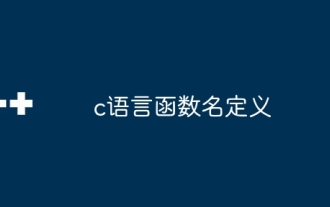
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
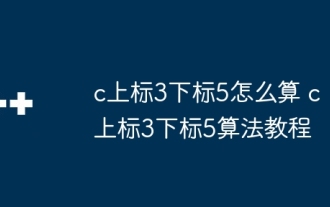
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
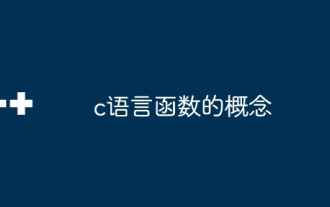
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
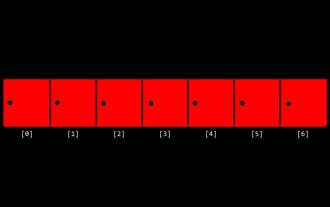
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
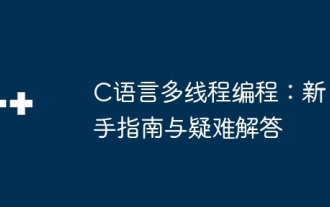
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
