


How can I protect my Yii application against cross-site scripting (XSS) attacks?
How can I protect my Yii application against cross-site scripting (XSS) attacks?
Protecting your Yii application against cross-site scripting (XSS) attacks involves implementing several layers of security measures. Here are some key strategies to safeguard your application:
-
Input Validation: Validate all user inputs to ensure they conform to expected formats. Use Yii's built-in validation rules or custom rules to filter out malicious data. For example, you can use
safe
andfilter
validators to sanitize inputs. -
Output Encoding: Always encode output data that is sent to the browser. Yii provides helpers like
Html::encode()
to escape special characters, preventing them from being interpreted as HTML or JavaScript. - Use of CSRF Protection: Yii automatically includes CSRF (Cross-Site Request Forgery) protection in forms. Ensure that this feature is enabled and correctly implemented in your application.
- Content Security Policy (CSP): Implement a Content Security Policy to reduce the risk of XSS attacks. You can set CSP headers using Yii's response object to define which sources of content are allowed.
- Regular Security Updates: Keep your Yii framework and all related libraries up to date to benefit from the latest security patches and enhancements.
-
Security Headers: Utilize security headers like
X-Content-Type-Options
,X-Frame-Options
, andX-XSS-Protection
to enhance browser security settings.
By combining these practices, you can significantly reduce the vulnerability of your Yii application to XSS attacks.
What are the best practices for input validation in Yii to prevent XSS vulnerabilities?
Implementing robust input validation in Yii is critical to preventing XSS vulnerabilities. Here are some best practices:
-
Use Yii's Validation Rules: Leverage Yii's built-in validation rules in your models to enforce data integrity. Common rules include
required
,string
,number
,email
, andurl
. For example:1
2
3
4
5
6
7
8
public
function
rules()
{
return
[
[[
'username'
],
'required'
],
[[
'username'
],
'string'
,
'max'
=> 255],
[[
'email'
],
'email'
],
];
}
Copy after login - Custom Validation: For more complex validations, use custom validator functions. You can create custom rules to check for specific conditions or patterns in the input data.
- Sanitization: Use filters to sanitize user input. Yii provides the
filter
validator, which can be used to apply various filters liketrim
,strip_tags
, or custom filters. - Whitelist Approach: Adopt a whitelist approach to validate inputs. Only allow inputs that meet your predefined criteria and reject all others.
- Validate All Inputs: Ensure every piece of user input is validated, including form data, URL parameters, and cookies.
Regular Expressions: Utilize regular expressions for more granular control over input validation. For example, to validate a username:
1
2
3
4
5
6
public
function
rules()
{
return
[
[[
'username'
],
'match'
,
'pattern'
=>
'/^[a-zA-Z0-9_] $/'
],
];
}
Copy after login
By adhering to these practices, you can effectively validate input in Yii and reduce the risk of XSS vulnerabilities.
How can I implement output encoding in Yii to safeguard against XSS attacks?
Implementing output encoding in Yii is crucial for safeguarding against XSS attacks. Here's how you can do it:
Using Html::encode(): Use the
Html::encode()
method to encode any output that is rendered as HTML. This method converts special characters to their HTML entities, preventing the browser from interpreting them as code.1
echo
Html::encode(
$userInput
);
Copy after loginHtmlPurifier Extension: For more robust HTML output sanitization, you can use the HtmlPurifier extension. This extension can remove malicious HTML while keeping the content safe.
1
2
3
4
use
yii\htmlpurifier\HtmlPurifier;
$purifier
=
new
HtmlPurifier();
echo
$purifier
->process(
$userInput
);
Copy after loginJson Encoding: When outputting JSON data, use
Json::encode()
with theJSON_HEX_TAG
andJSON_HEX_AMP
options to prevent XSS in JSON responses.1
2
3
use
yii\helpers\Json;
echo
Json::encode(
$data
, JSON_HEX_TAG | JSON_HEX_AMP);
Copy after loginAttribute Encoding: For HTML attributes, use
Html::encode()
or specific attribute encoders likeHtml::attributeEncode()
to ensure safe attribute values.1
echo
'<input type="text" value="'
. Html::encode(
$userInput
) .
'">'
;
Copy after loginCSP Headers: In addition to encoding, implementing Content Security Policy headers can further protect against XSS by restricting the sources of executable scripts.
1
Yii::
$app
->response->headers->add(
'Content-Security-Policy'
,
"default-src 'self'; script-src 'self' 'unsafe-inline';"
);
Copy after login
By consistently applying these output encoding techniques, you can significantly enhance the security of your Yii application against XSS attacks.
Are there any Yii extensions that can help enhance security against XSS?
Yes, several Yii extensions can help enhance security against XSS attacks. Here are some notable ones:
yii2-htmlpurifier: This extension integrates HTML Purifier into your Yii application. HTML Purifier is a powerful library that can sanitize HTML input to remove malicious code while preserving safe content.
1
composer
require
--prefer-dist yiidoc/yii2-htmlpurifier
Copy after loginyii2-esecurity: This extension provides additional security features, including XSS filtering, CSRF protection, and more advanced security headers.
1
composer
require
--prefer-dist mihaildev/yii2-elasticsearch
Copy after loginyii2-csrf: This extension enhances Yii's built-in CSRF protection, making it more robust and configurable.
1
composer
require
--prefer-dist 2amigos/yii2-csrf
Copy after loginyii2-csp: This extension helps implement and manage Content Security Policy headers in your Yii application, which can further protect against XSS by restricting script sources.
1
composer
require
--prefer-dist linslin/yii2-csp
Copy after loginyii2-secure-headers: This extension adds security headers to your application, including those that can mitigate XSS attacks, like
X-XSS-Protection
andContent-Security-Policy
.1
composer
require
--prefer-dist wbraganca/yii2-secure-headers
Copy after login
By integrating these extensions into your Yii application, you can bolster its defenses against XSS attacks and enhance overall security.
The above is the detailed content of How can I protect my Yii application against cross-site scripting (XSS) attacks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










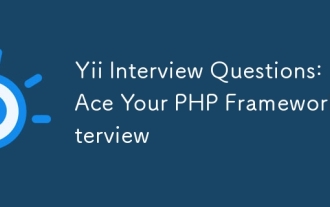
When preparing for an interview with Yii framework, you need to know the following key knowledge points: 1. MVC architecture: Understand the collaborative work of models, views and controllers. 2. ActiveRecord: Master the use of ORM tools and simplify database operations. 3. Widgets and Helpers: Familiar with built-in components and helper functions, and quickly build the user interface. Mastering these core concepts and best practices will help you stand out in the interview.

YiiremainspopularbutislessfavoredthanLaravel,withabout14kGitHubstars.ItexcelsinperformanceandActiveRecord,buthasasteeperlearningcurveandasmallerecosystem.It'sidealfordevelopersprioritizingefficiencyoveravastecosystem.
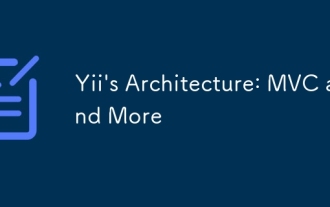
Yii framework adopts an MVC architecture and enhances its flexibility and scalability through components, modules, etc. 1) The MVC mode divides the application logic into model, view and controller. 2) Yii's MVC implementation uses action refinement request processing. 3) Yii supports modular development and improves code organization and management. 4) Use cache and database query optimization to improve performance.

Yii is a high-performance PHP framework designed for fast development and efficient code generation. Its core features include: MVC architecture: Yii adopts MVC architecture to help developers separate application logic and make the code easier to maintain and expand. Componentization and code generation: Through componentization and code generation, Yii reduces the repetitive work of developers and improves development efficiency. Performance Optimization: Yii uses latency loading and caching technologies to ensure efficient operation under high loads and provides powerful ORM capabilities to simplify database operations.
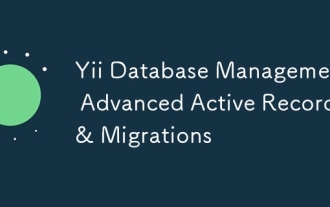
Advanced ActiveRecord and migration tools in the Yii framework are the key to efficiently managing databases. 1) Advanced ActiveRecord supports complex queries and data operations, such as associated queries and batch updates. 2) The migration tool is used to manage database structure changes and ensure secure updates to the schema.
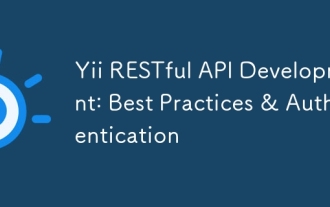
Developing a RESTful API in the Yii framework can be achieved through the following steps: Defining a controller: Use yii\rest\ActiveController to define a resource controller, such as UserController. Configure authentication: Ensure the security of the API by adding HTTPBearer authentication mechanism. Implement paging and sorting: Use yii\data\ActiveDataProvider to handle complex business logic. Error handling: Configure yii\web\ErrorHandler to customize error responses, such as handling when authentication fails. Performance optimization: Use Yii's caching mechanism to optimize frequently accessed resources and improve API performance.
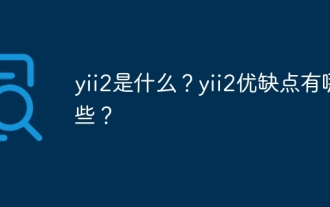
Yii2 is a powerful PHP framework that has been widely praised by developers. With its high performance, scalability and user-friendly interface, it becomes ideal for building large, complex web applications. However, like any framework, Yii2 has some advantages and disadvantages to consider.
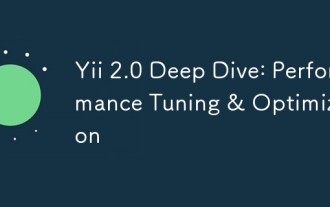
Strategies to improve Yii2.0 application performance include: 1. Database query optimization, using QueryBuilder and ActiveRecord to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page cache; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii2.0 applications can be significantly improved.
