How can I prevent SQL injection vulnerabilities in ThinkPHP?
How can I prevent SQL injection vulnerabilities in ThinkPHP?
Preventing SQL injection vulnerabilities in ThinkPHP involves a multi-layered approach that focuses on using safe query mechanisms and ensuring proper input handling. Here are key strategies to adopt:
-
Use Parameterized Queries: ThinkPHP supports parameterized queries through the
Db
class. These queries separate the SQL logic from the data, which prevents malicious SQL from being injected. For example:$result = Db::table('users') ->where('username', '=', $username) ->select();
Copy after loginIn this example,
$username
is a parameter that is automatically escaped and quoted, reducing the risk of SQL injection. Avoid Raw SQL: Minimize the use of raw SQL statements. If raw SQL is necessary, use placeholders to safely insert values:
$result = Db::query('SELECT * FROM users WHERE username = ?', [$username]);
Copy after loginThe
?
is a placeholder that ThinkPHP will bind to the$username
value.ORM and Query Builder: Leverage ThinkPHP’s Object-Relational Mapping (ORM) and Query Builder capabilities. They offer a higher level of abstraction from raw SQL, inherently providing protections against SQL injection:
$user = User::where('username', $username)->find();
Copy after login- Regular Updates and Patching: Keep your ThinkPHP framework and all related dependencies updated to the latest secure versions. Regular updates often include patches for newly discovered vulnerabilities.
- Proper Error Handling: Configure your application to handle errors gracefully without revealing sensitive information. In ThinkPHP, you can use the
try-catch
block to manage exceptions and prevent error details from being exposed to users.
What are the best practices for securing database queries in ThinkPHP?
Securing database queries in ThinkPHP extends beyond preventing SQL injection and includes several best practices:
- Limit Database Privileges: The database user account used by your application should have the minimum necessary privileges. This reduces the potential damage if an exploit is successful.
- Use Prepared Statements Consistently: Even when dealing with complex queries, always opt for prepared statements or ORM methods that automatically sanitize inputs.
- Avoid Dynamic SQL: Try to avoid constructing SQL queries based on user input dynamically. If you must, ensure all inputs are properly escaped or use parameterized queries.
- Implement Query Logging and Monitoring: Enable query logging in your ThinkPHP application to monitor and review executed queries. This can help in detecting unusual activities or potential security threats.
- Validate Query Results: After executing queries, validate the results to ensure they meet expected criteria, which can help detect anomalies that might arise from injection attempts.
- Secure Configuration Files: Keep database credentials and other sensitive configuration data encrypted or in secure storage, not in plain text in the codebase.
How can I validate and sanitize user inputs to protect against SQL injection in ThinkPHP?
Validating and sanitizing user inputs is crucial in preventing SQL injection attacks. Here’s how you can achieve this in ThinkPHP:
Input Validation: Before processing any data, validate it against expected formats. Use ThinkPHP’s built-in validation features to ensure that inputs match the expected data type and length:
$validate = new \think\Validate([ 'username' => 'require|max:25', 'password' => 'require|min:6', ]); if (!$validate->check($data)) { // Validation failed, handle errors }
Copy after login- Sanitizing Inputs: While ThinkPHP’s query methods handle escaping for SQL, it's still good practice to sanitize inputs at the application level. Use PHP’s built-in functions to strip out potentially harmful characters or use third-party libraries for more advanced sanitization.
Use Filter Functions: PHP’s filter functions can be used within ThinkPHP to sanitize inputs:
$username = filter_input(INPUT_POST, 'username', FILTER_SANITIZE_STRING);
Copy after loginHTML Entities: If the input might be displayed in HTML contexts, convert special characters to their HTML entities to prevent cross-site scripting (XSS) attacks:
$username = htmlspecialchars($username, ENT_QUOTES, 'UTF-8');
Copy after login- Blacklist and Whitelist: Employ a combination of blacklisting known bad patterns and whitelisting acceptable inputs. However, be cautious with blacklisting, as it's less secure than whitelisting.
Which tools or extensions can help detect SQL injection vulnerabilities in ThinkPHP applications?
To detect SQL injection vulnerabilities in ThinkPHP applications, you can use various tools and extensions:
- OWASP ZAP (Zed Attack Proxy): An open-source web application security scanner that can identify SQL injection vulnerabilities. It supports ThinkPHP applications and can be configured for automated scans.
- Burp Suite: A comprehensive platform for web application security testing. It includes tools for intercepting and manipulating HTTP/S traffic, which can be used to test for SQL injection. The Pro version offers more advanced scanning capabilities.
- SQLMap: A dedicated SQL injection and database takeover tool. It automates the process of detecting and exploiting SQL injection flaws and supports databases commonly used with ThinkPHP.
- PHPStan: A PHP static analysis tool that can be configured to look for potential SQL injection vulnerabilities within your ThinkPHP code by analyzing the flow of data into SQL queries.
- SonarQube: A tool that offers code quality and security analysis. It can integrate into your development workflow to scan for SQL injection vulnerabilities in ThinkPHP applications.
- Acunetix: A web vulnerability scanner that can test for SQL injection vulnerabilities. It supports ThinkPHP and can perform both automated and manual testing.
Using these tools regularly in your development and testing processes will help maintain a high level of security in your ThinkPHP applications.
The above is the detailed content of How can I prevent SQL injection vulnerabilities in ThinkPHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


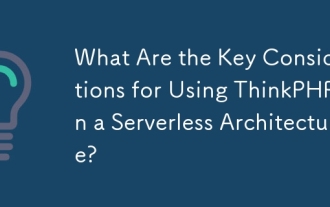
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
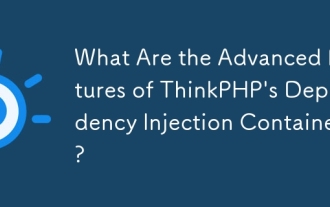
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
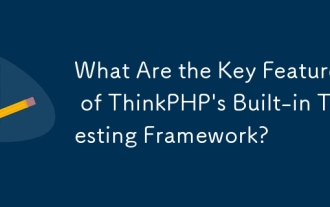
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
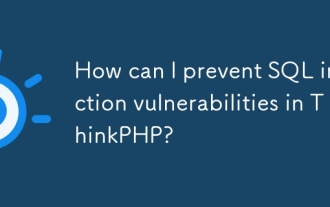
The article discusses preventing SQL injection vulnerabilities in ThinkPHP through parameterized queries, avoiding raw SQL, using ORM, regular updates, and proper error handling. It also covers best practices for securing database queries and validat
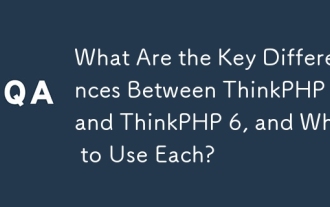
The article discusses key differences between ThinkPHP 5 and 6, focusing on architecture, features, performance, and suitability for legacy upgrades. ThinkPHP 5 is recommended for traditional projects and legacy systems, while ThinkPHP 6 suits new pr
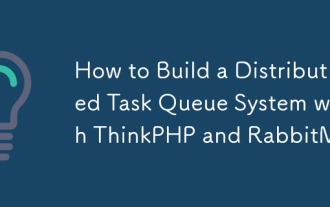
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
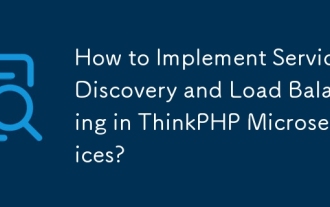
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
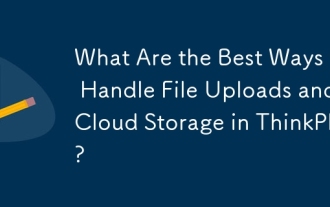
The article discusses best practices for handling file uploads and integrating cloud storage in ThinkPHP, focusing on security, efficiency, and scalability.
