


How do I use multi-stage builds in Docker to create smaller, more secure images?
How do I use multi-stage builds in Docker to create smaller, more secure images?
Multi-stage builds in Docker are a feature that allows you to use multiple FROM
statements in your Dockerfile. Each FROM
statement can start a new stage of the build process, and you can copy artifacts from one stage to another. This method is especially useful for creating smaller, more secure Docker images by separating the build environment from the runtime environment.
Here’s how you can use multi-stage builds to achieve this:
-
Define Build Stage: Start by defining a build stage where you compile your application or prepare your artifacts. For instance, you might use a
golang
image to compile a Go application.FROM golang:1.16 as builder WORKDIR /app COPY . . RUN go build -o myapp
Copy after login Define Runtime Stage: After the build stage, define a runtime stage with a minimal base image. Copy only the necessary artifacts from the build stage into this runtime stage.
FROM alpine:3.14 COPY --from=builder /app/myapp /myapp CMD ["/myapp"]
Copy after login
By using multi-stage builds, you end up with a final image that contains only what is needed to run your application, which is significantly smaller and has fewer potential vulnerabilities compared to the image used for building.
What are the best practices for organizing code in a multi-stage Docker build?
Organizing code effectively in a multi-stage Docker build can greatly enhance the efficiency and clarity of your Dockerfile. Here are some best practices:
Separate Concerns: Use different stages for different purposes (e.g., building, testing, and deploying). This separation of concerns makes your Dockerfile easier to understand and maintain.
# Build stage FROM node:14 as builder WORKDIR /app COPY package*.json ./ RUN npm install COPY . . RUN npm run build # Test stage FROM node:14 as tester WORKDIR /app COPY --from=builder /app . RUN npm run test # Runtime stage FROM node:14-alpine WORKDIR /app COPY --from=builder /app/build /app/build CMD ["node", "app/build/index.js"]
Copy after loginMinimize the Number of Layers: Combine RUN commands where possible to reduce the number of layers in your image. This practice not only speeds up the build process but also makes the resulting image smaller.
RUN apt-get update && \ apt-get install -y some-package && \ rm -rf /var/lib/apt/lists/*
Copy after login- Use
.dockerignore
: Create a.dockerignore
file to exclude unnecessary files from being copied into the Docker build context. This speeds up the build process and reduces the image size. - Optimize Copy Operations: Only copy the files necessary for each stage. For example, in the build stage for a Node.js application, you might copy
package.json
first, runnpm install
, and then copy the rest of the application. - Use Named Stages: Give meaningful names to your stages to make the Dockerfile easier to read and maintain.
How can I optimize caching in multi-stage Docker builds to improve build times?
Optimizing caching in multi-stage Docker builds can significantly reduce build times. Here are several strategies to achieve this:
Order of Operations: Place frequently changing commands towards the end of your Dockerfile. Docker will cache the layers from the beginning of the Dockerfile, speeding up subsequent builds.
FROM node:14 as builder WORKDIR /app COPY package*.json ./ RUN npm install COPY . . RUN npm run build
Copy after loginIn this example,
npm install
is less likely to change than the application code, so it's placed before theCOPY . .
command.- Use Multi-stage Builds: Each stage can be cached independently. This means you can leverage the build cache for each stage, potentially saving time on subsequent builds.
Leverage BuildKit: Docker BuildKit offers improved build caching mechanisms. Enable BuildKit by setting the environment variable
DOCKER_BUILDKIT=1
and use the newRUN --mount
command to mount cache directories.# syntax=docker/dockerfile:experimental FROM golang:1.16 as builder RUN --mount=type=cache,target=/root/.cache/go-build \ go build -o myapp
Copy after login-
Minimize the Docker Build Context: Use a
.dockerignore
file to exclude unnecessary files from the build context. A smaller context means less data to transfer and a quicker build. - Use Specific Base Images: Use lightweight and stable base images to reduce the time it takes to pull the base layers during the build.
What security benefits do multi-stage Docker builds provide compared to single-stage builds?
Multi-stage Docker builds provide several security benefits compared to single-stage builds:
- Smaller Image Size: By copying only the necessary artifacts from the build stage to the runtime stage, multi-stage builds result in much smaller final images. Smaller images have a reduced attack surface because they contain fewer components that could be vulnerable.
- Reduced Vulnerabilities: Since the final image does not include build tools or dependencies required only during the build process, there are fewer opportunities for attackers to exploit vulnerabilities in those tools.
- Isolation of Build and Runtime Environments: Multi-stage builds allow you to use different base images for building and running your application. The build environment can be more permissive and include tools necessary for compiling or packaging, while the runtime environment can be more restricted and optimized for security.
- Easier Compliance: Smaller, more focused images are easier to scan for vulnerabilities and ensure compliance with security policies, making it easier to maintain a secure environment.
- Limiting Secrets Exposure: Since sensitive data (like API keys used during the build) does not need to be included in the final image, multi-stage builds can help in preventing secrets from being exposed in the runtime environment.
By leveraging multi-stage builds, you can significantly enhance the security posture of your Docker images while also optimizing their size and performance.
The above is the detailed content of How do I use multi-stage builds in Docker to create smaller, more secure images?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Docker is a must-have skill for DevOps engineers. 1.Docker is an open source containerized platform that achieves isolation and portability by packaging applications and their dependencies into containers. 2. Docker works with namespaces, control groups and federated file systems. 3. Basic usage includes creating, running and managing containers. 4. Advanced usage includes using DockerCompose to manage multi-container applications. 5. Common errors include container failure, port mapping problems, and data persistence problems. Debugging skills include viewing logs, entering containers, and viewing detailed information. 6. Performance optimization and best practices include image optimization, resource constraints, network optimization and best practices for using Dockerfile.

Docker security enhancement methods include: 1. Use the --cap-drop parameter to limit Linux capabilities, 2. Create read-only containers, 3. Set SELinux tags. These strategies protect containers by reducing vulnerability exposure and limiting attacker capabilities.

DockerVolumes ensures that data remains safe when containers are restarted, deleted, or migrated. 1. Create Volume: dockervolumecreatemydata. 2. Run the container and mount Volume: dockerrun-it-vmydata:/app/dataubuntubash. 3. Advanced usage includes data sharing and backup.

Using Docker on Linux can improve development and deployment efficiency. 1. Install Docker: Use scripts to install Docker on Ubuntu. 2. Verify the installation: Run sudodockerrunhello-world. 3. Basic usage: Create an Nginx container dockerrun-namemy-nginx-p8080:80-dnginx. 4. Advanced usage: Create a custom image, build and run using Dockerfile. 5. Optimization and Best Practices: Follow best practices for writing Dockerfiles using multi-stage builds and DockerCompose.

Docker provides three main network modes: bridge network, host network and overlay network. 1. The bridge network is suitable for inter-container communication on a single host and is implemented through a virtual bridge. 2. The host network is suitable for scenarios where high-performance networks are required, and the container directly uses the host's network stack. 3. Overlay network is suitable for multi-host DockerSwarm clusters, and cross-host communication is realized through the virtual network layer.

DockerSwarm can be used to build scalable and highly available container clusters. 1) Initialize the Swarm cluster using dockerswarminit. 2) Join the Swarm cluster to use dockerswarmjoin--token:. 3) Create a service using dockerservicecreate-namemy-nginx--replicas3nginx. 4) Deploy complex services using dockerstackdeploy-cdocker-compose.ymlmyapp.

The core of Docker monitoring is to collect and analyze the operating data of containers, mainly including indicators such as CPU usage, memory usage, network traffic and disk I/O. By using tools such as Prometheus, Grafana and cAdvisor, comprehensive monitoring and performance optimization of containers can be achieved.
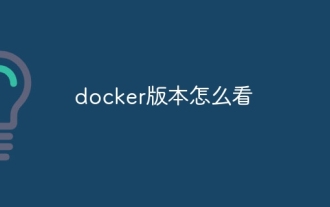
To get the Docker version, you can perform the following steps: Run the Docker command "docker --version" to view the client and server versions. For Mac or Windows, you can also view version information through the Version tab of the Docker Desktop GUI or the About Docker Desktop menu.
