Context-Aware Web Components Are Easier Than You Think
This article explores the often-overlooked lifecycle callbacks of web components, demonstrating how they enable context-aware elements. We'll build a web component that reacts to its environment, showcasing the power of these functions.
Series Overview
This article is part of a series on simplifying web component development:
- Web Components: Easier Than You Think
- Interactive Web Components: Easier Than You Think
- Using Web Components in WordPress: Easier Than You Think
- Supercharging Built-in Elements with Web Components: Easier Than You Think
- Context-Aware Web Components: Easier Than You Think (This Article)
- Web Component Pseudo-Classes and Pseudo-Elements: Easier Than You Think
Web Component Lifecycle Callbacks
Four key callbacks govern a web component's lifecycle:
-
connectedCallback
: Fired when the element is added to the DOM. -
disconnectedCallback
: Fired when the element is removed from the DOM. -
adoptedCallback
: Fired when the element is moved to a new document. -
attributeChangedCallback
: Fired when an observed attribute changes.
Let's illustrate these with a practical example.
Post-Apocalyptic Person Component
We'll create a <postapocalyptic-person></postapocalyptic-person>
component. Each person is either human or zombie, determined by the .human
or .zombie
class on its parent element. The component will display an appropriate image using a shadow DOM.
customElements.define( "postapocalyptic-person", class extends HTMLElement { constructor() { super(); this.shadowRoot = this.attachShadow({ mode: "open" }); } // ... lifecycle callbacks will be added here ... } );
Our initial HTML:
<div class="humans"> <postapocalyptic-person></postapocalyptic-person> </div> <div class="zombies"> <postapocalyptic-person></postapocalyptic-person> </div>
Using connectedCallback
connectedCallback
is called when <postapocalyptic-person></postapocalyptic-person>
is added to the page. We'll use it to add the image:
connectedCallback() { const image = document.createElement("img"); if (this.parentNode.classList.contains("humans")) { image.src = "https://assets.codepen.io/1804713/lady.png"; } else if (this.parentNode.classList.contains("zombies")) { image.src = "https://assets.codepen.io/1804713/ladyz.png"; } this.shadowRoot.appendChild(image); }
This ensures the correct image is displayed based on the parent's class. Note: connectedCallback
can fire multiple times; use this.isConnected
to check connection status.
Counting People with connectedCallback
Let's add buttons to add/remove people and track counts:
<div> <button id="addbtn">Add Person</button> <button id="rmvbtn">Remove Person</button> <br>Humans: <span id="human-count">0</span> Zombies: <span id="zombie-count">0</span> </div>
Button event listeners:
// ... (add/remove person logic) ...
Updated connectedCallback
to update counts:
connectedCallback() { // ... (image logic) ... // Update counts based on image source }
Updating Counts with disconnectedCallback
disconnectedCallback
decrements counts when a person is removed. We'll use the image source as a proxy for type:
disconnectedCallback() { const image = this.shadowRoot.querySelector('img'); // Decrement counts based on image source }
Clown Detection with adoptedCallback
and attributeChangedCallback
We'll introduce the possibility of hidden clowns, moving them to an <iframe></iframe>
using adoptedCallback
and revealing them with attributeChangedCallback
. The details of this advanced example are omitted for brevity, but the core concept involves using adoptedCallback
to detect when a component is moved to a new document and attributeChangedCallback
to react to attribute changes, updating the image accordingly.
This illustrates how lifecycle callbacks provide powerful tools for creating dynamic and context-aware web components, making them more versatile and responsive.
The above is the detailed content of Context-Aware Web Components Are Easier Than You Think. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
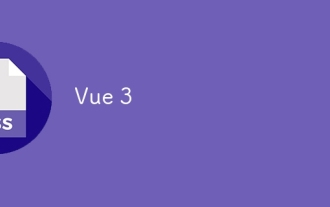
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
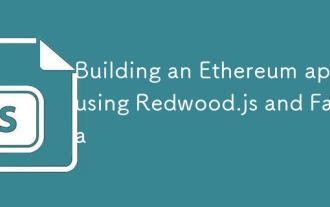
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
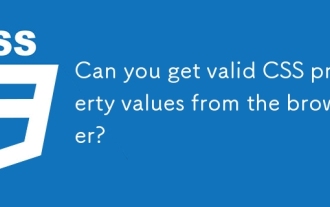
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
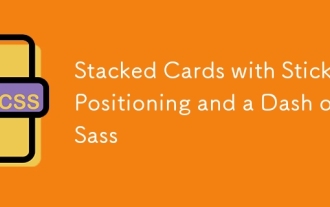
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
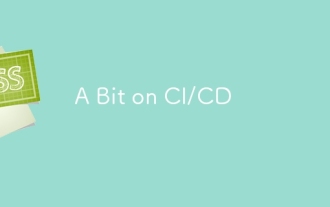
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
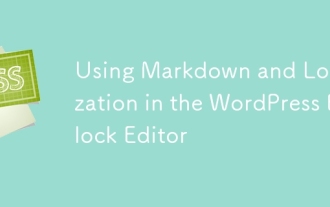
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
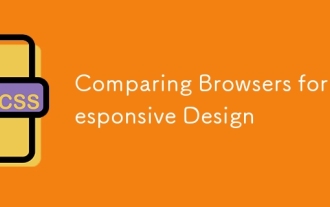
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
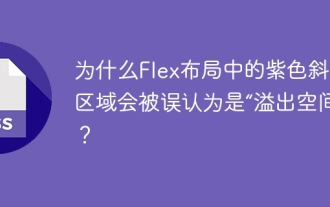
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
