A Handy Little System for Animated Entrances in CSS
A website is not just a static document, some details can make the website more vivid and interesting. What if the web page content is not displayed directly, but appears in a pop-up, slide, fade-in or rotated way? While such animations are not always practical, in some cases they can attract users' attention to specific elements, strengthen distinctions between elements, and even indicate state changes, so they are not completely useless.
So I created a set of CSS tools to add animation effects to an element when it enters the field of view. Yes, this is completely pure CSS implementation. It not only provides multiple animations and variants, but also supports the interleaving effect of animations, which can be almost like creating a scene.
For example, like this:
This is really just a simplified version of this more advanced version:
We first explain the basics of creating animations, then introduce some of the details I added, how to interleave animations, and how to apply them in HTML elements, and finally, we will see how to achieve all of this with respect to the user's reduced movement preferences.
Basic knowledge
The core idea is to add a simple CSS @keyframes
animation, apply it to whatever we want to animate the page when it loads. Let's let an element fade in and change from opacity: 0
to opacity: 1
in half a second:
1 2 3 4 5 6 7 8 9 10 11 |
|
Please note that there is also a 0.5-second animation-delay
here to allow the rest of the website to load first. animation-fill-mode: backwards
is used to ensure that our initial animation state is active when the page is loading. Without this, our animation elements will pop up before we want to.
If we are lazy, we can stop here. However, CSS-Tricks readers certainly won't be lazy, so let's see how to use a system to improve this kind of thing.
More cool animations
It's more fun to have a variety of animations than just using one or two animations. We don't even need to create a bunch of new @keyframes
to make more animations. Creating a new class is very simple, we just need to change the frames used by the animation and keep it the same for all times.
The number of CSS animations is almost unlimited. (See animate.style, which is a huge collection.) CSS filters such as blur()
, brightness()
, and saturate()
, and of course CSS transformations can also be used to create more variants.
But now, let's start with a new animation class that uses CSS transformation to make the element "pop up" into place.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
I added a small cubic-bezier()
time curve from Lea Verou's indispensable cubic-bezier.com for a resilient rebound effect.
Add delay
We can do better! For example, we can make elements animate at different times. This creates an interleaving effect, creating complex-looking motions without the need for a lot of code.
This feels great using CSS filters, CSS conversions and an interleaved animation of about one tenth of a second on three page elements:
All we do is create a new class for each element that separates the time the element starts to animation, using animation-delay
value that is one tenth of a second apart.
1 2 3 |
|
Everything else is exactly the same. Remember that our base latency is 0.5s, so these helper classes start counting from there.
Respect the accessibility preferences
Let's be excellent web citizens and remove animations for users who have enabled reduced sports preferences:
1 2 3 |
|
In this way, the animation will not load and the elements will enter the field of view as normal. "Reduce" movement does not always mean "removal" movement, which is worth reminding.
Apply animation to HTML elements
So far we've looked at the basic animation as well as a slightly more advanced one, which we can make it more advanced with interleaved animation delays (included in the new class). We also see how to respect users’ sports preferences at the same time.
Although there are real-time demonstrations that show these concepts, we don't actually cover how to apply our work to HTML. The cool thing is that we can use it on almost any element, whether it's div, span, article, header, section, table, form...you know.
What we are going to do is: we want to use our animation system on three HTML elements, each of which gets three classes. We can hardcode all the animation code to the element itself, but splitting it allows us to get a reusable animation system.
- .animate: This is the base class that contains our core animation declarations and time.
- Animation type: We will use the previous "pop-up" animation, but we can also use fade-in animation. This class is technically optional, but it is a great way to apply different movements.
- .delay-
: As mentioned earlier, we can create different classes to interleave the time the animation starts on each element, resulting in a neat effect. This class is also optional.
So our animation elements may now look like this:
1 2 3 |
|
Let's count them!
in conclusion
Check it out: We start with a seemingly basic set @keyframes
and turn it into a complete system for applying interesting animations for elements entering the field of view.
This is of course very interesting. But the biggest takeaway for me is that the examples we see form a complete system that can be used to create baselines, different types of animations, interleaving delays and respecting user motion preferences. For me, these are all the elements of a flexible and easy-to-use system. It gave us a lot with very little stuff without a bunch of extra scraps.
What we cover really can be a complete animation library. But of course I didn't stop there. I've provided all my CSS animation files to you. There are several animation types in it, including 15 classes with different delays, which can be used to interleave things. I've been using these in my own projects, but this is still an early version and I'd love to receive feedback about it. Please enjoy and let me know what you think in the comments!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 |
|
The above is the detailed content of A Handy Little System for Animated Entrances in CSS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










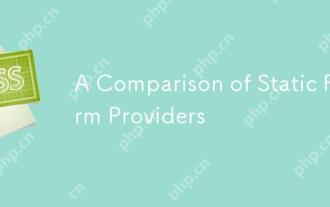
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
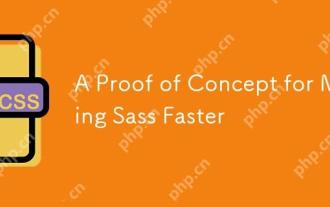
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
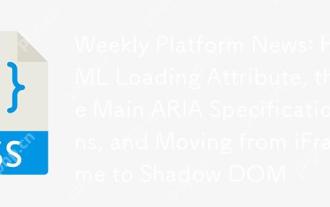
In this week's roundup of platform news, Chrome introduces a new attribute for loading, accessibility specifications for web developers, and the BBC moves
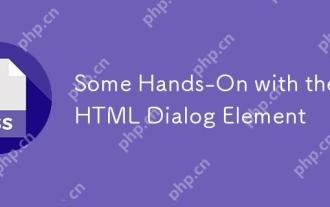
This is me looking at the HTML element for the first time. I've been aware of it for a while, but haven't taken it for a spin yet. It has some pretty cool and
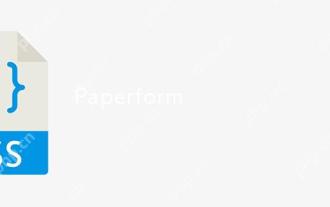
Buy or build is a classic debate in technology. Building things yourself might feel less expensive because there is no line item on your credit card bill, but
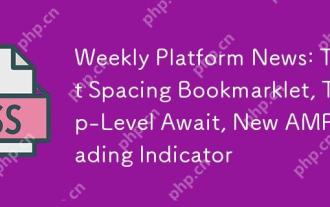
In this week's roundup, a handy bookmarklet for inspecting typography, using await to tinker with how JavaScript modules import one another, plus Facebook's
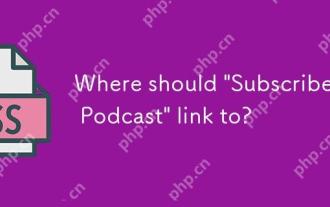
For a while, iTunes was the big dog in podcasting, so if you linked "Subscribe to Podcast" to like:
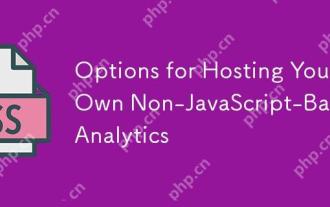
There are loads of analytics platforms to help you track visitor and usage data on your sites. Perhaps most notably Google Analytics, which is widely used
