What are the best practices for writing efficient JavaScript code?
What are the best practices for writing efficient JavaScript code?
Writing efficient JavaScript code is crucial for ensuring that web applications run smoothly and respond quickly to user interactions. Here are some best practices to help achieve this:
-
Minimize DOM Manipulation:
The Document Object Model (DOM) is slow to manipulate due to its need to reflow and repaint the page. To optimize, batch DOM updates and use document fragments or virtual DOM techniques (as used in frameworks like React) to reduce the number of direct DOM manipulations. -
Use Efficient Loops:
Instead of using traditionalfor
loops, consider usingforEach
,map
,filter
, orreduce
when working with arrays. These methods are often optimized and can lead to cleaner and more readable code. However, in performance-critical sections, traditionalfor
loops might still be faster. -
Avoid Unnecessary Function Calls:
Function calls can be expensive, especially when they involve closures or heavy computations. Try to minimize the number of function calls by storing the results of expensive operations if they are to be reused. -
Leverage Asynchronous Programming:
Useasync/await
, Promises, or callbacks to handle asynchronous operations efficiently. This helps in keeping the main thread free for rendering and user interactions. -
Optimize Variable Scope:
Variables declared inside a function (local variables) have faster access times compared to global variables. Use local scope where possible to improve performance. -
Use Efficient Data Structures:
Choose the right data structure for the task at hand. For example, useSet
orMap
when you need to store unique values or key-value pairs, respectively, as these are often more efficient than traditional arrays and objects. -
Avoid Using
with
Statement:
Thewith
statement can make code harder to read and can lead to performance issues because it alters the scope chain, which can slow down JavaScript engines. -
Lazy Loading:
Implement lazy loading for images, scripts, and other resources to reduce initial load time and save bandwidth.
By adhering to these best practices, developers can significantly improve the efficiency of their JavaScript code.
What tools can help optimize JavaScript performance?
Several tools are available to help developers optimize JavaScript performance:
-
Google Chrome DevTools:
Chrome DevTools offer a Performance tab that helps analyze runtime performance, including JavaScript execution time, memory usage, and rendering performance. It provides detailed insights into what's slowing down your application. -
Webpack:
Webpack is a module bundler that helps optimize JavaScript by minifying code, reducing the number of HTTP requests, and enabling features like code splitting and lazy loading. -
Babel:
Babel is used to transpile modern JavaScript code to older versions for better browser compatibility. It can also be configured to remove unused code, which helps in reducing the bundle size. -
ESLint:
ESLint is a static code analysis tool that helps maintain code quality and adherence to best practices. It can be configured to flag potential performance issues. -
Lighthouse:
Lighthouse is an open-source, automated tool for improving the quality of web pages. It has audits for performance, accessibility, progressive web apps, SEO, and more. It provides detailed reports on areas where performance can be improved. -
JSPerf:
JSPerf is a benchmarking tool that helps compare the performance of different JavaScript snippets. It is useful for testing different approaches to a problem and choosing the most efficient one. -
Profiling Tools like Node.js Inspector:
For server-side JavaScript, tools like the Node.js Inspector can help profile and optimize code running in a Node.js environment.
Using these tools, developers can measure, analyze, and improve the performance of their JavaScript applications.
How can code refactoring improve JavaScript efficiency?
Code refactoring involves restructuring existing code without changing its external behavior, and it can significantly improve JavaScript efficiency in several ways:
-
Improving Readability and Maintainability:
Refactoring can simplify complex code, making it easier to read and maintain. This indirectly improves efficiency because well-maintained code is less likely to harbor hidden performance issues. -
Eliminating Redundancies:
Refactoring helps identify and remove redundant code, which directly impacts performance by reducing the amount of code that needs to be executed. -
Optimizing Algorithms and Data Structures:
By refactoring, developers can replace inefficient algorithms or data structures with more efficient alternatives, such as usingSet
instead of an array for storing unique values. -
Reducing Function Calls:
Through refactoring, functions that are called frequently can be inlined or optimized to reduce the overhead of function calls. -
Improving Memory Usage:
Refactoring can help manage memory more effectively by cleaning up unused variables and optimizing data structures for better memory utilization. -
Enabling Use of Modern JavaScript Features:
Refactoring can update code to use newer JavaScript features that are more performant, such as usingasync/await
instead of nested callbacks. -
Enhancing Code Reusability:
By extracting common patterns into reusable functions or modules, refactoring can reduce code duplication and improve overall efficiency.
By regularly refactoring their code, developers can ensure that their JavaScript applications remain efficient and scalable.
What common mistakes should be avoided when writing JavaScript?
When writing JavaScript, it's important to avoid common mistakes that can lead to inefficient code or bugs. Here are some pitfalls to watch out for:
-
Overusing Global Variables:
Using too many global variables can lead to namespace pollution and unintended side effects. Always encapsulate variables within functions or modules when possible. -
Ignoring Browser Compatibility:
Not considering browser compatibility can lead to code that works in one environment but fails in others. Always test your code across different browsers and versions. -
Misusing
this
Keyword:
Thethis
keyword can be tricky in JavaScript, especially within callbacks or nested functions. Use arrow functions or bind methods to ensurethis
refers to the expected context. -
Neglecting Error Handling:
Failing to handle errors can lead to unexpected application crashes. Use try/catch blocks and error events to manage and recover from errors gracefully. -
Using Blocking Synchronous Operations:
Synchronous operations can block the main thread, causing the application to become unresponsive. Use asynchronous programming patterns likeasync/await
or callbacks to avoid this. -
Ignoring Memory Leaks:
Not cleaning up event listeners, timers, or other resources can lead to memory leaks. Always remove unnecessary references and clean up after objects are no longer needed. -
Excessive DOM Manipulation:
Directly manipulating the DOM too frequently can degrade performance. Use efficient techniques like virtual DOM or document fragments to reduce the number of DOM operations. -
Not Using Strict Mode:
Strict mode helps catch common coding errors and prevents the use of certain error-prone features. Always enable strict mode to improve code quality and performance. -
Ignoring Performance Bottlenecks:
Failing to profile and optimize performance can lead to slow applications. Regularly use profiling tools to identify and fix bottlenecks.
By being aware of these common mistakes, developers can write more efficient, robust, and maintainable JavaScript code.
The above is the detailed content of What are the best practices for writing efficient JavaScript code?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
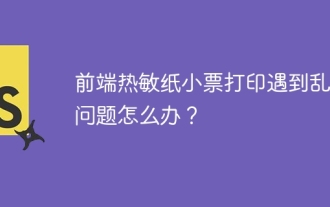
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
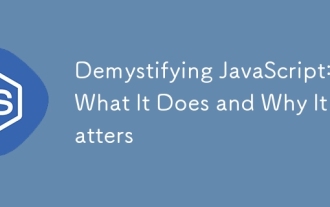
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
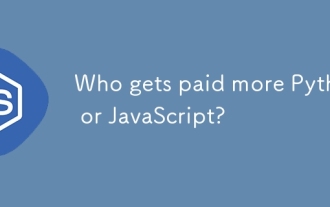
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
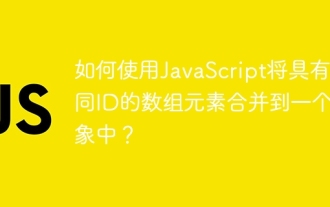
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
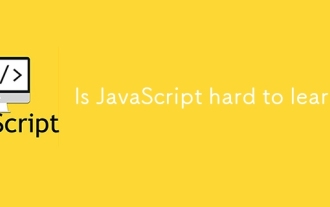
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
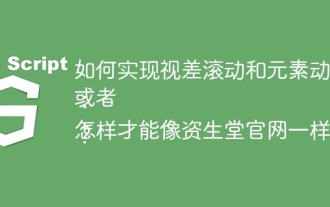
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
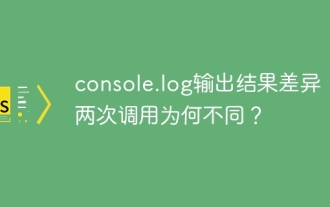
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
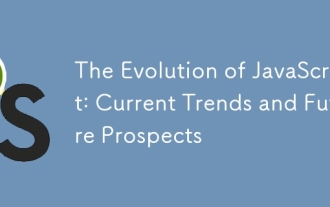
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
