How do I use the Pimpl idiom in C to reduce compilation dependencies?
How do I use the Pimpl idiom in C to reduce compilation dependencies?
The Pimpl idiom, or Pointer to Implementation idiom, is used in C to reduce compilation dependencies by hiding the private implementation details of a class from its public interface. Here's a step-by-step guide on how to use the Pimpl idiom:
-
Declare the Public Interface:
First, define your class's public interface in the header file. The private members are replaced with a pointer to the implementation.// myclass.h #include <memory> class MyClass { public: MyClass(); ~MyClass(); void doSomething(); private: struct Impl; // Forward declaration of the implementation std::unique_ptr<Impl> pimpl; // Pointer to the implementation };
Copy after login Define the Private Implementation:
Create a separate source file where you define the private implementation details.// myclass.cpp #include "myclass.h" struct MyClass::Impl { // Private members go here int someData; void someHelperFunction(); }; MyClass::MyClass() : pimpl(std::make_unique<Impl>()) { // Initialize implementation } MyClass::~MyClass() = default; void MyClass::doSomething() { pimpl->someHelperFunction(); }
Copy after login- Use Forward Declarations:
In the header file, use forward declarations for any classes that are used only in the implementation file. This reduces the need to include additional headers in the public interface, which can speed up compilation. - Manage the Implementation Pointer:
Use a smart pointer likestd::unique_ptr
to manage the lifetime of the implementation. This ensures proper memory management without requiring users of the class to know about the implementation details.
By following these steps, you can effectively use the Pimpl idiom to reduce compilation dependencies, as the public interface is no longer dependent on the implementation details.
What are the key benefits of using the Pimpl idiom in C for managing dependencies?
Using the Pimpl idiom in C offers several key benefits for managing dependencies:
- Reduced Compilation Dependencies:
The Pimpl idiom separates the interface from the implementation, allowing changes to the implementation without requiring recompilation of all files that include the class's header. This reduces the build time, especially in large projects. - Improved Binary Compatibility:
By hiding the implementation details, the Pimpl idiom can help maintain binary compatibility when making changes to the implementation. This means that you can update the implementation without breaking existing binaries that use the class. - Encapsulation and Abstraction:
The Pimpl idiom enhances encapsulation by completely hiding the implementation details from the public interface. This enforces the principle of least knowledge and improves the overall design of the code. - Reduced Header Bloat:
Since the implementation details are moved to the source file, the header file remains smaller and simpler. This reduces the amount of code that needs to be recompiled when the header changes. - Easier Testing and Maintenance:
With a clear separation between interface and implementation, testing and maintenance become easier. You can modify the implementation without affecting the interface, which is particularly useful for unit testing.
How can I implement the Pimpl idiom correctly to minimize recompilation in my C projects?
To implement the Pimpl idiom correctly and minimize recompilation, follow these best practices:
Use Forward Declarations:
In the header file, use forward declarations for any types that are only used in the implementation. This prevents unnecessary#include
directives in the header, which can trigger recompilation of other files.// myclass.h class SomeOtherClass; // Forward declaration class MyClass { // ... private: struct Impl; std::unique_ptr<Impl> pimpl; };
Copy after loginMove Implementation to Source File:
Ensure that all implementation details, including member variables and private methods, are defined in the source file. This keeps the header file clean and minimizes the need for recompilation.// myclass.cpp #include "myclass.h" #include "someotherclass.h" // Include here, not in the header struct MyClass::Impl { SomeOtherClass* someOtherClass; }; // Rest of the implementation
Copy after loginUse Smart Pointers:
Utilizestd::unique_ptr
orstd::shared_ptr
to manage the implementation pointer. This ensures proper memory management and simplifies the class's destructor.MyClass::MyClass() : pimpl(std::make_unique<Impl>()) {} MyClass::~MyClass() = default; // Let unique_ptr handle deletion
Copy after login- Minimize Inline Functions:
Avoid inline functions in the header file. If you need inline functions, consider moving them to the source file or using a separate inline header that clients can choose to include. - Use Pimpl Idiom Judiciously:
Apply the Pimpl idiom to classes that are frequently modified or have complex implementations. Overuse can lead to unnecessary complexity and performance overhead due to indirection.
By following these practices, you can effectively use the Pimpl idiom to minimize recompilation in your C projects.
What common pitfalls should I avoid when using the Pimpl idiom in C ?
When using the Pimpl idiom, it's important to be aware of the following common pitfalls and avoid them:
- Overuse:
Using the Pimpl idiom for every class can lead to unnecessary complexity and indirection. Apply it selectively to classes that benefit from reduced compilation dependencies or improved binary compatibility. - Performance Overhead:
The Pimpl idiom introduces an extra level of indirection, which can have a slight performance impact. Be mindful of this when using the idiom in performance-critical parts of your code. - Debugging Challenges:
The separation of interface and implementation can make debugging more difficult. Use appropriate debugging tools and techniques, such as runtime type information (RTTI) or custom logging, to help diagnose issues. - Increased Memory Usage:
The Pimpl idiom requires additional memory for the pointer to the implementation. In memory-constrained environments, this could be a concern. Consider the trade-offs carefully. Copy and Move Semantics:
Implementing copy and move semantics can be more complex with the Pimpl idiom. Ensure that you correctly implement these operations to avoid unexpected behavior.MyClass::MyClass(const MyClass& other) : pimpl(std::make_unique<Impl>(*other.pimpl)) {} MyClass& MyClass::operator=(const MyClass& other) { if (this != &other) { pimpl = std::make_unique<Impl>(*other.pimpl); } return *this; }
Copy after login-
Lack of Compile-Time Checks:
With the Pimpl idiom, some compile-time checks on the implementation are lost. This can lead to runtime errors if the implementation is incorrect. Use unit tests and runtime checks to mitigate this risk. -
Complex Destructors:
If the destructor needs to perform complex cleanup, managing it correctly with the Pimpl idiom can be challenging. Ensure that the destructor is properly implemented to handle all necessary cleanup tasks.
By being aware of these pitfalls and taking appropriate measures, you can effectively use the Pimpl idiom in your C projects while minimizing potential issues.
The above is the detailed content of How do I use the Pimpl idiom in C to reduce compilation dependencies?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
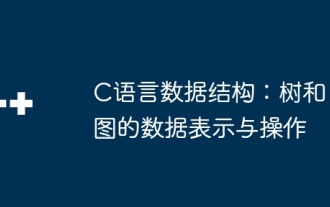
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
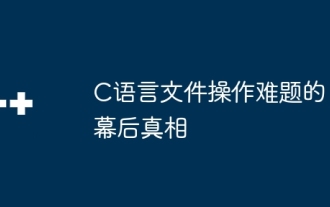
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
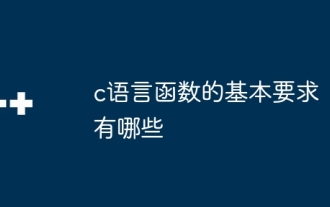
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
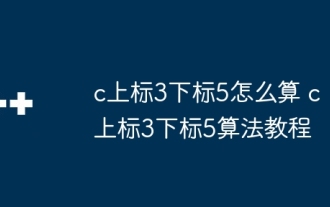
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
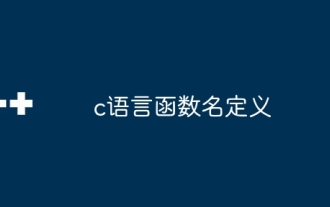
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
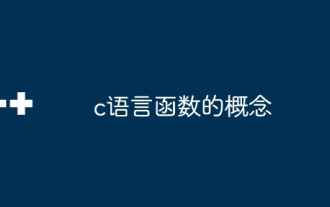
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
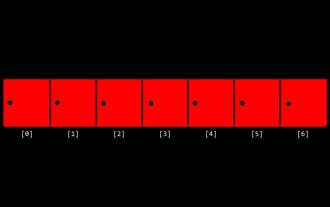
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
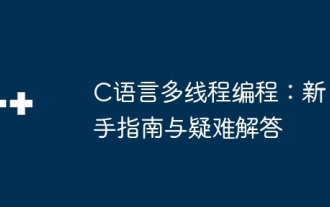
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
