


How does dynamic dispatch work in C and how does it affect performance?
How does dynamic dispatch work in C and how does it affect performance?
Dynamic dispatch in C is a mechanism that allows a program to determine at runtime which function to call based on the actual type of the object, rather than the type of the pointer or reference used to call the function. This is typically achieved through the use of virtual functions and polymorphism.
When a class declares a virtual function, the compiler sets up a virtual table (vtable) for that class. The vtable contains pointers to the implementations of the virtual functions. Each object of a class with virtual functions contains a pointer to the class's vtable. When a virtual function is called through a pointer or reference to a base class, the actual function called is determined at runtime by following the vtable pointer in the object.
This mechanism, while powerful and crucial for implementing polymorphism, comes with a performance cost:
- Indirect Function Call: The use of a vtable results in an indirect function call, which is typically slower than a direct function call used in static dispatch. The CPU must load the vtable pointer and then the function pointer before jumping to the function.
- Cache Misses: The indirect nature of the call can lead to more cache misses, as the processor might not predict the next function call correctly.
- Increased Memory Usage: Every object with virtual functions carries an additional vtable pointer, increasing the memory footprint.
- Compilation and Linking Overhead: The use of virtual functions may increase the complexity of the code, potentially leading to longer compile times and increased binary size.
What are the specific scenarios where dynamic dispatch in C can significantly impact application performance?
Dynamic dispatch can significantly impact application performance in the following scenarios:
- High-Frequency Calls: If virtual functions are called frequently in performance-critical sections of the code, the overhead of indirect calls and potential cache misses can accumulate, leading to noticeable performance degradation.
- Real-Time Systems: In systems where predictable timing is crucial, such as real-time operating systems, the variability introduced by dynamic dispatch can be detrimental.
- Embedded Systems: In resource-constrained environments, the additional memory required for vtables and the potential for slower execution might be critical.
- Games and Graphics Engines: These applications often require high performance and predictable execution paths. Overuse of dynamic dispatch in performance-critical loops can lead to frame rate drops or other performance issues.
- Large-Scale Applications: In applications with a large number of classes and inheritance hierarchies, the overhead of maintaining and traversing vtables can become significant.
How can developers optimize the use of dynamic dispatch in C to minimize performance overhead?
To minimize the performance overhead of dynamic dispatch, developers can employ the following strategies:
- Minimize Virtual Function Calls: Use virtual functions only where polymorphism is necessary. For cases where the exact type is known at compile-time, use non-virtual functions.
-
Use Final and Override: Using
final
andoverride
keywords can help the compiler optimize function calls.final
can prevent further overriding, potentially allowing the compiler to use more efficient dispatch methods. - Inline Functions: When possible, inline virtual functions to reduce the overhead of function calls. However, this is generally more effective with non-virtual functions.
- Virtual Function Tables (VTable) Layout Optimization: Some compilers offer options to optimize the layout of vtables, potentially reducing cache misses.
- Profile and Optimize Hot Paths: Use profiling tools to identify performance bottlenecks and optimize those sections by reducing the use of dynamic dispatch or using alternative approaches like template metaprogramming.
- Use of Design Patterns: Employ design patterns like the "Strategy Pattern" to encapsulate algorithms and provide flexibility without relying heavily on dynamic dispatch.
What are the trade-offs between using dynamic dispatch and static dispatch in C in terms of performance and code flexibility?
The trade-offs between dynamic dispatch and static dispatch in C are as follows:
Performance:
- Dynamic Dispatch: Generally slower due to the need for an indirect function call, potential cache misses, and increased memory usage. However, it allows for runtime polymorphism, which can be critical in many scenarios.
- Static Dispatch: Faster as it results in direct function calls, which are easier for the compiler and CPU to optimize. It eliminates the need for vtables and associated memory overhead.
Code Flexibility:
- Dynamic Dispatch: Offers high flexibility and extensibility. New classes can be added and used polymorphically without modifying existing code. This is particularly valuable in scenarios where the exact type of objects is determined at runtime.
- Static Dispatch: Less flexible as the function to be called must be known at compile-time. This can lead to more rigid code structures and may require code duplication or the use of templates to achieve similar flexibility to dynamic dispatch.
In summary, dynamic dispatch provides more flexibility and ease of maintenance, which can be critical for large and evolving systems, while static dispatch offers superior performance. Developers must weigh these factors based on the specific requirements of their application, often using a mix of both approaches to balance performance and flexibility.
The above is the detailed content of How does dynamic dispatch work in C and how does it affect performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


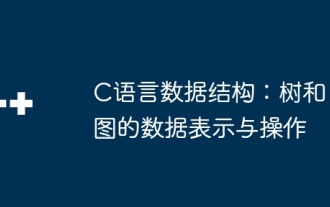
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
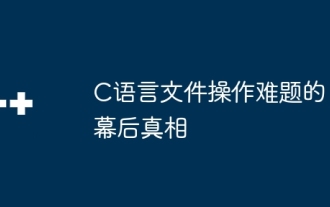
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
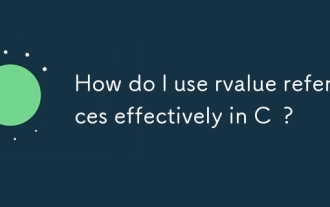
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
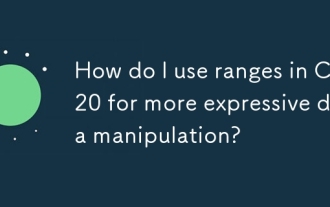
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
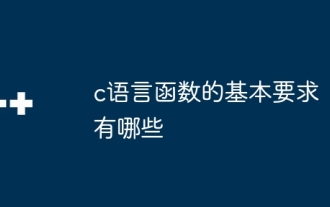
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
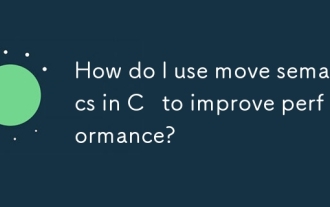
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
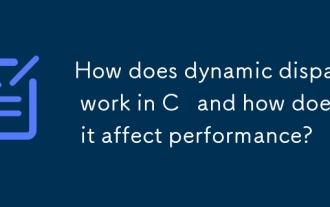
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
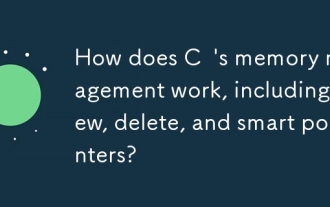
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
