How do I create and use custom Blade directives in Laravel?
How do I create and use custom Blade directives in Laravel?
Creating and using custom Blade directives in Laravel is a powerful feature that allows you to enhance your templating system. Here's how you can do it:
-
Define the Directive:
In Laravel, custom Blade directives are defined in a service provider, typically theAppServiceProvider
. You'll need to add your directive in theboot
method of this provider. Here's a step-by-step guide:- Open
app/Providers/AppServiceProvider.php
. -
In the
boot
method, use theBlade::directive
method to define your custom directive. For example, if you want to create a directive for formatting dates, you could do:use Illuminate\Support\Facades\Blade; public function boot() { Blade::directive('datetime', function ($expression) { return "<?php echo ($expression)->format('M d, Y H:i'); ?>"; }); }
Copy after login - The
$expression
parameter will contain the value passed to your directive when it's used in a Blade template.
- Open
Using the Directive:
Once you've defined your directive, you can use it in your Blade templates. Continuing the example above, you could use thedatetime
directive like this:<p>Published at: @datetime($post->created_at)</p>
Copy after loginWhen rendered, this will output the date in the format
M d, Y H:i
.- Testing the Directive:
After setting up your directive, it's a good idea to test it in a Blade template to ensure it works as expected. You might want to create a simple route and view to test your new directive.
By following these steps, you can create and use custom Blade directives to streamline your templating and add more functionality to your views.
What are the benefits of using custom Blade directives in Laravel applications?
Using custom Blade directives in Laravel applications offers several significant benefits:
- Improved Code Reusability:
Custom directives allow you to encapsulate complex logic that can be reused across multiple views. Instead of repeating the same code in different places, you can define it once as a directive. - Enhanced Readability:
By abstracting complex logic into directives, your Blade templates become cleaner and easier to read. Instead of seeing a block of PHP code, developers see a simple, meaningful directive name. - Consistency in Formatting and Output:
Custom directives can ensure that certain data is always displayed in a consistent format throughout your application. For example, using a directive to format dates or currency ensures all instances are formatted the same way. - Separation of Concerns:
Directives allow you to separate the presentation logic from the HTML structure in your views. This separation makes your code more maintainable and easier to test. - Performance Optimization:
In some cases, custom directives can be used to optimize the performance of your views by pre-processing data or reducing the complexity of the HTML that needs to be rendered.
By leveraging these benefits, custom Blade directives can significantly improve the development and maintenance of your Laravel applications.
Can custom Blade directives in Laravel improve the readability of my views?
Yes, custom Blade directives can significantly improve the readability of your views in Laravel. Here's how:
- Simplification of Complex Logic:
By encapsulating complex PHP logic within a directive, you reduce the clutter in your Blade templates. Instead of having to read through lines of PHP code, other developers (and even you, at a later date) can quickly understand what the directive does based on its name. - Clear Intention:
A well-named directive clearly communicates its purpose. For example,@datetime($post->created_at)
instantly tells you that it's formatting a datetime, whereas a block of PHP code might take more effort to decipher. - Reduced HTML Clutter:
By moving logic out of the HTML, your templates become cleaner and more focused on the structure and layout. This makes it easier to maintain and update your views. - Consistency Across Templates:
When you use the same directive across multiple views, it promotes consistency, which in turn makes your codebase more readable and easier to maintain. - Easier to Follow and Understand:
With custom directives, the flow of your template becomes more straightforward. Instead of navigating through mixed HTML and PHP, you follow a series of directives and HTML tags, making the structure of your page clearer.
By improving the readability of your views, custom Blade directives make your Laravel application more maintainable and easier to work with.
How can I manage and organize custom Blade directives efficiently in a large Laravel project?
In a large Laravel project, managing and organizing custom Blade directives efficiently is crucial to maintaining a clean and scalable codebase. Here are some strategies to achieve this:
Dedicated Service Provider for Directives:
Instead of cluttering theAppServiceProvider
with numerous directives, create a dedicated service provider just for your custom directives. For example,DirectiveServiceProvider
. This keeps your directives organized and makes it easier to manage them as your project grows.// app/Providers/DirectiveServiceProvider.php namespace App\Providers; use Illuminate\Support\Facades\Blade; use Illuminate\Support\ServiceProvider; class DirectiveServiceProvider extends ServiceProvider { public function boot() { // Define your directives here Blade::directive('datetime', function ($expression) { return "<?php echo ($expression)->format('M d, Y H:i'); ?>"; }); // More directives... } public function register() { // } }
Copy after loginThen, register this provider in your
config/app.php
:'providers' => [ // Other Service Providers... App\Providers\DirectiveServiceProvider::class, ],
Copy after login-
Group Related Directives:
Group directives that serve similar purposes. For example, you might have a set of directives for formatting dates, another set for handling user permissions, and so on. This makes it easier to find and update related directives. -
Document Your Directives:
Keep a documentation file or use inline comments to explain what each directive does, its parameters, and any special usage notes. This helps other developers (and yourself) understand and use the directives correctly. -
Version Control and Testing:
Ensure that your custom directives are covered by version control and include unit tests if possible. This helps track changes and ensures that directives continue to work as expected as your project evolves. -
Use a Naming Convention:
Adopt a consistent naming convention for your directives. For example, prefix them with a specific string (e.g.,@app.datetime
) to clearly distinguish them from built-in directives. -
Modular Approach:
If your project is large and complex, consider organizing directives into modules or packages. This allows you to share directives across multiple projects and keeps your main application codebase lean.
By implementing these strategies, you can effectively manage and organize your custom Blade directives, ensuring that your large Laravel project remains maintainable and scalable.
The above is the detailed content of How do I create and use custom Blade directives in Laravel?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


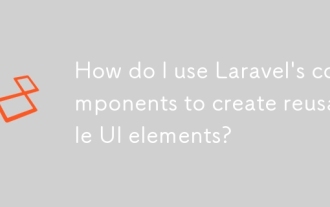
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
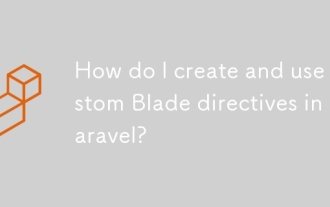
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
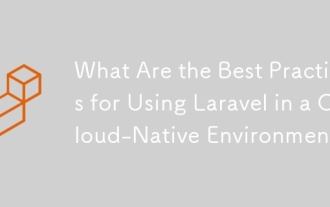
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
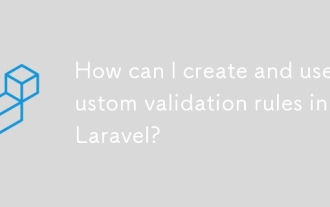
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
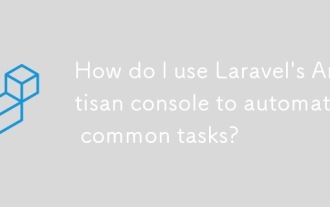
Laravel's Artisan console automates tasks like generating code, running migrations, and scheduling. Key commands include make:controller, migrate, and db:seed. Custom commands can be created for specific needs, enhancing workflow efficiency.Character
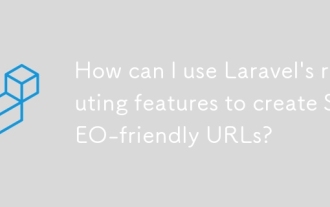
The article discusses using Laravel's routing to create SEO-friendly URLs, covering best practices, canonical URLs, and tools for SEO optimization.Word count: 159

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
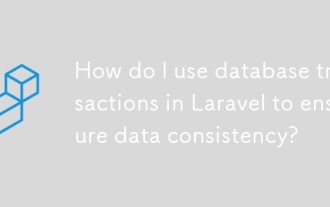
The article discusses using database transactions in Laravel to maintain data consistency, detailing methods with DB facade and Eloquent models, best practices, exception handling, and tools for monitoring and debugging transactions.
