How do I set up a custom session handler in phpStudy?
How do I set up a custom session handler in phpStudy?
Setting up a custom session handler in phpStudy involves a few key steps that enable you to take control over how session data is stored, retrieved, and managed. Here’s how you can do it:
-
Create a Custom Session Handler Class:
- First, you'll need to create a PHP class that extends
SessionHandler
and implements the necessary methods for handling sessions. These methods includeopen
,close
,read
,write
,destroy
, andgc
(garbage collection). -
Example of a basic custom session handler:
class CustomSessionHandler extends SessionHandler { public function open($save_path, $name) { // Initialization code return true; } public function read($id) { // Read session data return ''; } public function write($id, $data) { // Write session data return true; } public function close() { // Cleanup code return true; } public function destroy($id) { // Remove session data return true; } public function gc($maxlifetime) { // Garbage collection return true; } }
Copy after login
- First, you'll need to create a PHP class that extends
Register the Custom Session Handler:
Once the class is created, you need to instantiate it and register it with PHP using
session_set_save_handler()
. This should be done before any session start.$handler = new CustomSessionHandler(); session_set_save_handler($handler, true);
Copy after login
Start the Session:
After setting the handler, you can start the session as usual.
session_start();
Copy after login
Configure phpStudy:
- Ensure that phpStudy is configured to use the script where your custom session handler is defined. This might involve setting the appropriate document root and ensuring PHP can access the necessary files.
What are the steps to configure session handling in phpStudy?
Configuring session handling in phpStudy involves tweaking PHP settings and possibly integrating custom handlers. Here are the steps:
Access php.ini File:
- Locate your
php.ini
file within the phpStudy directory. You can edit this file to change session-related settings.
- Locate your
Modify Session Settings:
Adjust the session settings according to your needs. Key settings include:
session.save_handler
: Change this if you want to use a custom handler.session.save_path
: Set the directory for session storage.session.gc_probability
andsession.gc_divisor
: Adjust these for garbage collection frequency.session.save_handler = user session.save_path = "/path/to/sessions" session.gc_probability = 1 session.gc_divisor = 1000
Copy after login
Restart phpStudy:
- After modifying the
php.ini
file, restart the phpStudy server to ensure the changes take effect.
- After modifying the
Test Configuration:
- Use a test script to ensure that the session handling works as expected. You can start a session and check the session data to verify the setup.
Can I use a custom session handler to improve performance in phpStudy?
Yes, using a custom session handler can improve performance in phpStudy, depending on your specific requirements and implementation. Here’s how:
Optimized Storage:
- If the default file-based session storage is a bottleneck, a custom handler can use more efficient storage solutions like databases or Redis. These can offer better read/write performance compared to disk I/O.
Fine-Tuned Garbage Collection:
- By implementing custom garbage collection, you can better manage session data, preventing unnecessary accumulation and improving system performance.
Load Balancing:
- For large-scale applications, a custom session handler can support load balancing by distributing session data across multiple servers, thereby enhancing scalability and performance.
Session Data Compression:
- You can implement session data compression within your custom handler, reducing the storage footprint and improving transmission times over networks.
However, keep in mind that while custom session handlers can offer performance benefits, they also add complexity to your application. Ensure that the performance gains justify the added development and maintenance efforts.
How do I troubleshoot issues with a custom session handler in phpStudy?
Troubleshooting issues with a custom session handler in phpStudy involves a systematic approach. Here’s how to diagnose and fix common problems:
Enable Error Reporting:
Ensure that PHP error reporting and logging are enabled in your
php.ini
file to capture any errors or warnings from your custom session handler.display_errors = On log_errors = On error_log = /path/to/php_error.log
Copy after login
Log Session Operations:
Add logging within your custom session handler to track the flow and any errors during session operations (open, read, write, etc.). This can help pinpoint where issues occur.
public function read($id) { error_log("Reading session: $id"); // Read logic here }
Copy after login
-
Test Each Method:
- Isolate and test each method of the session handler (
open
,close
,read
,write
,destroy
,gc
) separately to ensure they are functioning as expected.
- Isolate and test each method of the session handler (
-
Check Permissions:
- Ensure that the session storage path has the correct permissions and is writable by the PHP process. This is crucial if you’re using file-based storage.
-
Use Debugging Tools:
- Utilize PHP debugging tools like Xdebug or integrated development environments (IDEs) to step through your code and identify where the issues occur.
-
Verify Configuration:
- Double-check your
php.ini
and custom handler configuration. Ensure thatsession.save_handler
is set touser
and that all required settings are correctly specified.
- Double-check your
-
Consult Logs:
- Review the PHP error logs and any custom logs from your session handler for clues about what might be going wrong.
By following these steps, you can systematically identify and resolve issues with your custom session handler in phpStudy.
The above is the detailed content of How do I set up a custom session handler in phpStudy?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


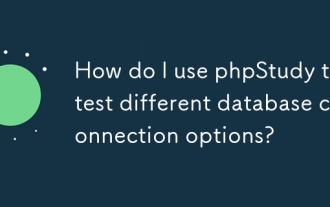
phpStudy enables testing various database connections. Key steps include installing servers, enabling PHP extensions, and configuring scripts. Troubleshooting focuses on common errors like connection failures and extension issues.Character count: 159
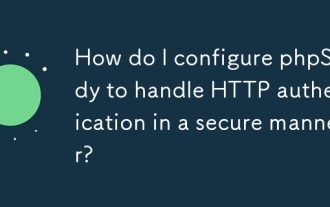
The article discusses configuring phpStudy for secure HTTP authentication, detailing steps like enabling HTTPS, setting up .htaccess and .htpasswd files, and best practices for security.Main issue: Ensuring secure HTTP authentication in phpStudy thro
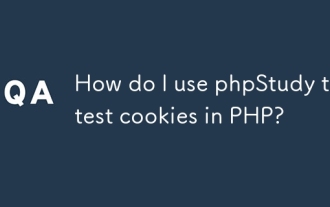
The article details using phpStudy for PHP cookie testing, covering setup, cookie verification, and common issues. It emphasizes practical steps and troubleshooting for effective testing.[159 characters]
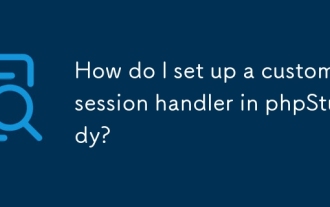
Article discusses setting up custom session handlers in phpStudy, including creation, registration, and configuration for performance improvement and troubleshooting.
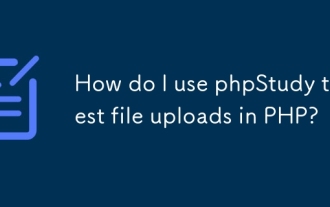
Article discusses using phpStudy for PHP file uploads, addressing setup, common issues, configuration for large files, and security measures.
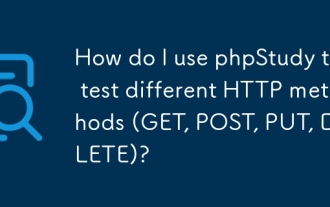
Article discusses using phpStudy to test HTTP methods (GET, POST, PUT, DELETE) through PHP scripts and configuration.
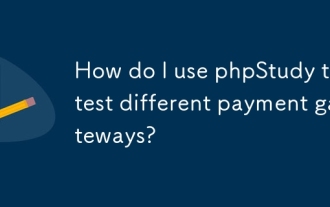
The article explains how to use phpStudy to test different payment gateways by setting up the environment, integrating APIs, and simulating transactions. Main issue: configuring phpStudy effectively for payment gateway testing.
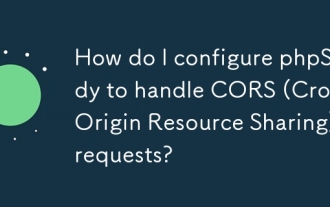
Article discusses configuring phpStudy for CORS, detailing steps for Apache and PHP settings, and troubleshooting methods.
