


How do I use regular expressions effectively in JavaScript for pattern matching?
How do I use regular expressions effectively in JavaScript for pattern matching?
Regular expressions, often abbreviated as regex, are powerful tools for pattern matching and manipulation of text. In JavaScript, you can create and use regular expressions in several ways:
-
Creating a Regular Expression:
-
Literal Notation: The simplest way to create a regex is by using the literal notation, where the pattern is enclosed between slashes:
let regex = /pattern/;
-
Constructor Function: You can also create a regex using the
RegExp
constructor:let regex = new RegExp('pattern');
This method is useful when you need to construct the regex pattern dynamically.
-
Literal Notation: The simplest way to create a regex is by using the literal notation, where the pattern is enclosed between slashes:
-
Basic Usage:
- To test if a string matches a pattern, use the
test()
method:let result = /pattern/.test('string');
- To search for a match within a string, use the
exec()
method:let match = /pattern/.exec('string');
- For replacing parts of a string, use the
replace()
method:let replaced = 'string'.replace(/pattern/, 'replacement');
- To test if a string matches a pattern, use the
-
Flags: Regex flags modify the behavior of the pattern. Common flags in JavaScript include:
-
g
: Global search, finds all matches rather than stopping after the first match. -
i
: Case-insensitive search. -
m
: Multiline search, treats beginning and end characters (^ and $) as working over multiple lines.
-
-
Character Classes and Quantifiers:
- Use character classes like
[abc]
to match any of the enclosed characters, or[^abc]
to match any character except those enclosed. - Quantifiers like
*
,?
, and{n,m}
allow you to specify how many times a character or group should occur.
- Use character classes like
-
Groups and Capturing:
- Use parentheses
()
to create groups, which can be used for capturing parts of the match. You can reference captured groups in replacements or further matches using$1
,$2
, etc.
- Use parentheses
Here's a practical example:
let emailPattern = /^[a-zA-Z0-9._-] @[a-zA-Z0-9.-] \.[a-zA-Z]{2,4}$/; let testEmail = "example@email.com"; if (emailPattern.test(testEmail)) { console.log("Valid email"); } else { console.log("Invalid email"); }
This example uses a regex to validate an email address. The pattern ensures that the string starts with one or more alphanumeric characters, periods, underscores, or hyphens, followed by an @ symbol, and then more alphanumeric characters ending in a domain suffix.
What are some common pitfalls to avoid when using regex in JavaScript?
Using regex effectively requires careful attention to avoid common mistakes:
Greedy vs. Non-Greedy Quantifiers:
- By default, quantifiers like
*
and.*?
instead of.*
to make quantifiers non-greedy and match the least amount of text possible.
- By default, quantifiers like
Escaping Special Characters:
- Characters like
.
,*
,?
,{
,}
,(
,)
,[
,]
,^
,$
,|
, and\
have special meanings in regex. To match them literally, you must escape them with a backslash\
inside the regex pattern.
- Characters like
Performance Issues:
- Complex regex patterns can be computationally expensive. Try to simplify your patterns where possible and avoid unnecessary backtracking by using non-greedy quantifiers and anchors.
Incorrect Use of Anchors:
- The
^
and$
anchors match the start and end of the string (or line if them
flag is used). Misusing these can lead to unexpected matches or failures.
- The
Overlooking Case Sensitivity:
- Without the
i
flag, regex patterns are case-sensitive. Remember to include this flag if you need case-insensitive matching.
- Without the
Not Testing Thoroughly:
- Always test your regex with a variety of inputs, including edge cases, to ensure it behaves as expected.
Can you recommend any tools or libraries that enhance regex functionality in JavaScript?
Several tools and libraries can enhance your regex functionality in JavaScript:
XRegExp:
- XRegExp is a JavaScript library that provides additional regex syntax and flags not available in the built-in
RegExp
object. It supports features like named capture groups, Unicode properties, and more.
- XRegExp is a JavaScript library that provides additional regex syntax and flags not available in the built-in
RegExr:
- RegExr is an online tool that allows you to create, test, and learn regex patterns interactively. It's useful for quick testing and learning, though it's not a library you'd include in your project.
Regex101:
- Similar to RegExr, Regex101 is a comprehensive online regex tester that also provides detailed explanations of matches. It supports JavaScript flavor and can be a valuable learning resource.
Regex-Generator:
- This npm package helps generate regex patterns based on a string or a set of strings. It's handy for creating initial regex patterns that you can later refine.
Debuggex:
- Debuggex is an online tool that visualizes regex patterns as railroad diagrams, helping you understand and debug your patterns visually.
How can I test and debug my regular expressions in a JavaScript environment?
Testing and debugging regex patterns effectively involves using a combination of techniques and tools:
Console Testing:
- You can start by using the browser's console or Node.js REPL to test your regex interactively. Use
console.log()
to see the results oftest()
,exec()
, andreplace()
operations.
let pattern = /hello/; console.log(pattern.test("hello world")); // true console.log(pattern.exec("hello world")); // ["hello", index: 0, input: "hello world", groups: undefined]
Copy after login- You can start by using the browser's console or Node.js REPL to test your regex interactively. Use
Online Regex Testers:
- Use tools like RegExr, Regex101, or Debuggex to test and refine your patterns. These tools often provide explanations and visual aids, which can help identify issues.
Integrated Development Environment (IDE) Support:
- Many modern IDEs and text editors, such as Visual Studio Code, WebStorm, and Sublime Text, offer built-in regex testing and debugging features. Look for regex evaluation tools in your editor's extensions or plugins.
Writing Unit Tests:
- Create unit tests using a testing framework like Jest or Mocha to test your regex patterns against a variety of inputs. This approach ensures that your patterns work correctly across different scenarios.
describe('Email Validation Regex', () => { let emailPattern = /^[a-zA-Z0-9._-] @[a-zA-Z0-9.-] \.[a-zA-Z]{2,4}$/; it('should validate correct email', () => { expect(emailPattern.test('example@email.com')).toBe(true); }); it('should reject incorrect email', () => { expect(emailPattern.test('example')).toBe(false); }); });
Copy after login-
Logging Intermediate Results:
- When debugging, log intermediate results of your regex operations to understand how the pattern is matching your input. Use
console.log()
at different steps to see what parts of the string are being captured.
- When debugging, log intermediate results of your regex operations to understand how the pattern is matching your input. Use
By combining these methods, you can effectively test and debug your regex patterns in a JavaScript environment, ensuring they work as intended and are efficient.
The above is the detailed content of How do I use regular expressions effectively in JavaScript for pattern matching?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










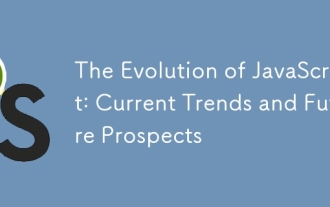
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
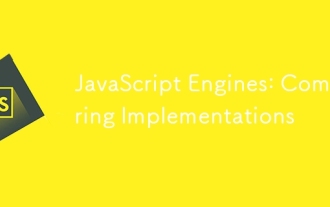
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
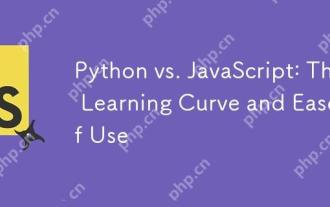
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
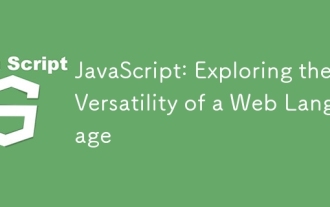
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
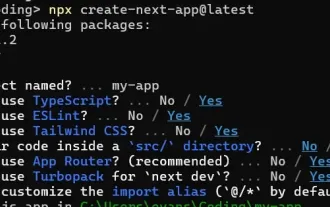
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
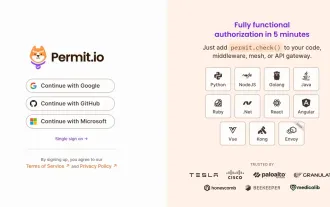
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
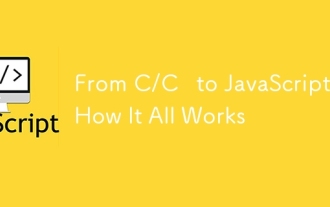
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
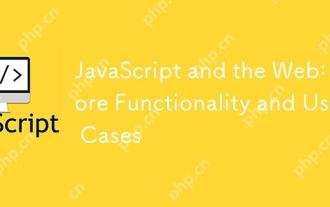
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
