


How to Implement Real-Time Data Synchronization with Workerman and MySQL?
How to Implement Real-Time Data Synchronization with Workerman and MySQL?
Implementing real-time data synchronization with Workerman and MySQL involves several steps, ensuring that data is seamlessly updated across different systems in real time. Here’s a detailed guide on how to achieve this:
-
Setting up Workerman:
-
Start by installing Workerman, a high-performance PHP socket server framework. You can use Composer to install it:
composer require workerman/workerman
Copy after login - Create a PHP file (e.g.,
Server.php
) to initialize and start the Workerman server.
-
Database Configuration:
- Configure a MySQL database to store the data that needs to be synchronized. Ensure that the database is accessible from the server running Workerman.
- Use a library such as
mysqli
orPDO
to interact with MySQL from PHP.
Real-time Data Synchronization Mechanism:
- Use Workerman to establish WebSocket connections with clients. When a data change occurs in the database, trigger an event that notifies the Workerman server.
- To detect changes in the database, you can use MySQL triggers or set up a daemon that polls the database for changes.
- Upon detecting a change, send the updated data through the WebSocket connection to the clients in real-time.
Sample Code for Workerman and MySQL Synchronization:
use Workerman\Worker; require_once __DIR__ . '/vendor/autoload.php'; $worker = new Worker('websocket://0.0.0.0:2346'); $worker->onConnect = function($connection) { echo "New connection\n"; }; $worker->onMessage = function($connection, $data) use ($worker) { // Simulating database check for changes if (/* database change detected */) { $connection->send(json_encode(['event' => 'update', 'data' => /* updated data */])); } }; $worker->onClose = function($connection) { echo "Connection closed\n"; }; Worker::runAll();
Copy after login-
Testing and Deployment:
- Test the setup thoroughly to ensure real-time updates are working as expected.
- Deploy the Workerman server on a production environment and ensure it can handle the expected load.
What are the best practices for setting up Workerman to handle real-time data from MySQL?
To effectively set up Workerman for handling real-time data from MySQL, follow these best practices:
-
Scalability:
- Design your Workerman server to be scalable. Workerman supports multi-process mode, which allows it to handle a larger number of concurrent connections.
- Use load balancing techniques to distribute the workload across multiple Workerman instances.
-
Error Handling and Logging:
- Implement robust error handling to manage connection issues or data processing errors.
- Use logging to monitor the system’s performance and troubleshoot issues quickly.
-
Connection Management:
- Implement a mechanism to handle connection drops gracefully. Use heartbeats to keep connections alive.
- Consider implementing reconnection logic for clients to ensure continuous synchronization.
-
Security:
- Secure WebSocket connections with SSL/TLS to protect data in transit.
- Implement authentication mechanisms to ensure that only authorized clients can connect and receive data.
-
Performance Optimization:
- Optimize database queries to minimize the load on the MySQL server.
- Use caching mechanisms to reduce the frequency of database queries.
-
Testing and Monitoring:
- Perform load testing to ensure the system can handle peak loads.
- Set up monitoring tools to track server performance and client connections in real-time.
How can I ensure data consistency when using Workerman for real-time MySQL synchronization?
Ensuring data consistency in a real-time synchronization system with Workerman and MySQL is crucial. Here are steps to achieve this:
-
Atomic Operations:
- Use transactions to ensure that changes to the database are atomic. This prevents partial updates that could lead to inconsistencies.
-
Optimistic Locking:
- Implement optimistic locking to manage concurrent updates to the same data. This can help prevent conflicts and maintain data integrity.
-
Conflict Resolution:
- Develop a strategy for resolving conflicts when multiple clients attempt to update the same data simultaneously. This may involve timestamp-based conflict resolution or a manual resolution process.
-
Data Validation:
- Implement validation checks on the server-side to ensure that the data being synchronized meets the required standards and constraints.
-
Versioning:
- Use data versioning to track changes over time. This allows you to roll back changes if needed and helps maintain a historical record of data states.
-
Eventual Consistency:
- Design the system to achieve eventual consistency, where data across different nodes or clients will eventually converge to a consistent state even if temporary discrepancies occur.
-
Testing for Consistency:
- Regularly test the system under various scenarios to ensure that data remains consistent across all clients and the database.
What are the common challenges faced when implementing real-time synchronization with Workerman and MySQL, and how to overcome them?
Implementing real-time synchronization with Workerman and MySQL can present several challenges. Here are some common ones and strategies to overcome them:
-
Scalability Issues:
- Challenge: As the number of clients grows, the server may struggle to maintain real-time updates.
- Solution: Implement horizontal scaling by distributing the workload across multiple Workerman instances using load balancers.
-
Connection Management:
- Challenge: Managing a large number of WebSocket connections can be challenging.
- Solution: Use Workerman’s built-in features to manage connections efficiently, and implement a reconnection strategy for clients.
-
Data Consistency:
- Challenge: Ensuring data consistency across multiple clients and the database.
- Solution: Use transactions, implement conflict resolution mechanisms, and test for consistency under various conditions.
-
Latency:
- Challenge: High latency can impact the real-time experience.
- Solution: Optimize the database queries, use caching, and ensure that the network infrastructure supports low latency.
-
Security Concerns:
- Challenge: Securing data during transmission and ensuring that only authorized clients can access the system.
- Solution: Use SSL/TLS to secure WebSocket connections and implement robust authentication and authorization mechanisms.
-
Monitoring and Debugging:
- Challenge: Identifying and resolving issues in a real-time system can be complex.
- Solution: Implement comprehensive logging and monitoring systems. Use tools to track performance metrics and connection status in real-time.
By understanding these challenges and implementing the recommended solutions, you can create a robust and efficient real-time data synchronization system using Workerman and MySQL.
The above is the detailed content of How to Implement Real-Time Data Synchronization with Workerman and MySQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


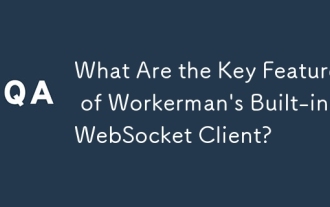
Workerman's WebSocket client enhances real-time communication with features like asynchronous communication, high performance, scalability, and security, easily integrating with existing systems.
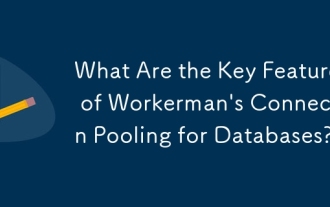
Workerman's connection pooling optimizes database connections, enhancing performance and scalability. Key features include connection reuse, limiting, and idle management. Supports MySQL, PostgreSQL, SQLite, MongoDB, and Redis. Potential drawbacks in
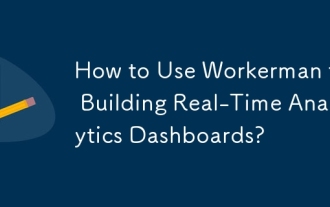
The article discusses using Workerman, a high-performance PHP server, to build real-time analytics dashboards. It covers installation, server setup, data processing, and frontend integration with frameworks like React, Vue.js, and Angular. Key featur

The article discusses implementing real-time data synchronization using Workerman and MySQL, focusing on setup, best practices, ensuring data consistency, and addressing common challenges.
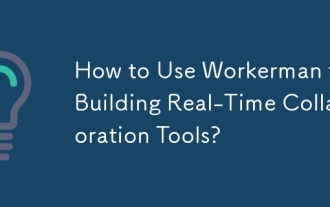
The article discusses using Workerman, a high-performance PHP server, to build real-time collaboration tools. It covers installation, server setup, real-time feature implementation, and integration with existing systems, emphasizing Workerman's key f

The article discusses integrating Workerman into serverless architectures, focusing on scalability, statelessness, cold starts, resource management, and integration complexity. Workerman enhances performance through high concurrency, reduced cold sta
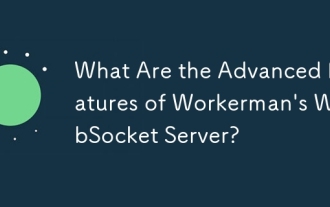
Workerman's WebSocket server enhances real-time communication with features like scalability, low latency, and security measures against common threats.
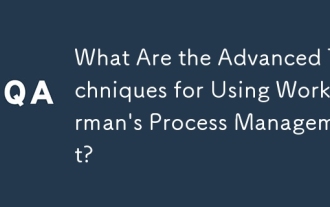
The article discusses advanced techniques for enhancing Workerman's process management, focusing on dynamic adjustments, process isolation, load balancing, and custom scripts to optimize application performance and reliability.
