What are React Hooks? Why were they introduced?
What are React Hooks? Why were they introduced?
React Hooks are functions that allow developers to use state and lifecycle features in functional components. Introduced in React 16.8, hooks represent a shift towards writing more concise and reusable code in React applications. The primary reason for introducing hooks was to solve the problem of code reuse across components, particularly in the context of functional components.
Prior to hooks, developers relied heavily on class components for managing state and side effects. However, class components came with several drawbacks, such as verbose syntax, difficulty in understanding lifecycle methods, and challenges in code reuse. Functional components, on the other hand, were simpler and easier to understand, but they lacked the ability to manage state and side effects.
React Hooks were introduced to:
-
Allow State and Lifecycle in Functional Components: Hooks like
useState
anduseEffect
let functional components manage state and handle side effects, thereby removing the need for class components. - Simplify Code: Hooks simplify the component logic by breaking it down into smaller, manageable functions.
- Enable Code Reuse: Custom hooks allow developers to extract component logic into reusable functions.
- Reduce Confusion: They eliminate complex lifecycle methods, making the code easier to understand and debug.
How do React Hooks enhance functional components?
React Hooks significantly enhance functional components in several ways:
-
State Management: With the
useState
hook, functional components can now manage local state without converting to a class. This makes state management straightforward and keeps components simple and readable. -
Side Effects Handling: The
useEffect
hook allows functional components to handle side effects such as data fetching, subscriptions, or manually changing the DOM. This unifies the handling of side effects in a single place, improving readability and maintainability. -
Context Usage: The
useContext
hook simplifies accessing React context within functional components. This makes it easier to pass data through the component tree without having to pass props down manually at every level. -
Performance Optimization: Hooks like
useMemo
anduseCallback
provide performance optimizations by memoizing expensive computations or callbacks, preventing unnecessary re-renders. - Code Organization and Reusability: By using custom hooks, developers can encapsulate complex logic into reusable functions, leading to cleaner and more maintainable code.
-
Testing: Functional components using hooks are generally easier to test than class components due to their simpler nature and the absence of
this
binding issues.
What problems do React Hooks solve in state management?
React Hooks address several problems in state management:
-
Complexity of Class Components: Before hooks, state management in React required using class components, which introduced complexity due to
this
binding and lifecycle methods. Hooks allow state management in functional components, which are more intuitive and less error-prone. - Code Duplication: Managing state often required duplicating logic across multiple components. Custom hooks enable developers to reuse stateful logic without changing the component hierarchy, thus reducing code duplication.
-
Lack of Composability: With class components, composing reusable stateful logic was difficult. Hooks like
useReducer
anduseState
make it easy to compose and manage state in a more modular way. - Difficulty in Understanding State Flow: Hooks make it easier to understand how state is being used and updated within components, as the logic is more centralized and straightforward compared to the fragmented nature of lifecycle methods in class components.
-
Performance Issues: Hooks like
useMemo
anduseCallback
can help manage state more efficiently by preventing unnecessary re-renders, thus optimizing application performance.
Which React Hook is most commonly used for side effects?
The React Hook most commonly used for side effects is useEffect
. The useEffect
hook allows developers to perform side effects in function components, such as fetching data, setting up subscriptions, or manually changing the DOM.
useEffect
can be used to run code after rendering, and it can be configured to run only when certain values have changed, or just once after the initial render. This flexibility makes it a powerful tool for managing side effects in React applications.
Here is a basic example of how useEffect
is used:
import React, { useState, useEffect } from 'react'; function ExampleComponent() { const [data, setData] = useState(null); useEffect(() => { // This effect runs after every render fetchData().then(result => setData(result)); }, []); // Empty dependency array means this effect runs once on mount return ( <div> {data ? <p>Data: {data}</p> : <p>Loading...</p>} </div> ); } async function fetchData() { // Simulate an API call return new Promise(resolve => setTimeout(() => resolve('Some data'), 1000)); }
In this example, useEffect
is used to fetch data when the component mounts, demonstrating its utility in handling side effects.
The above is the detailed content of What are React Hooks? Why were they introduced?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


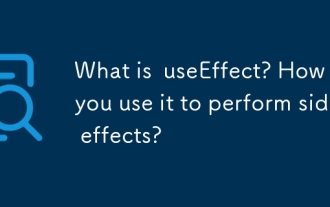
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
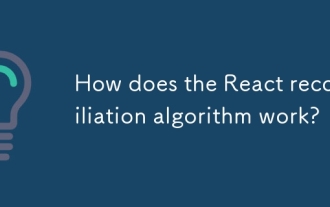
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
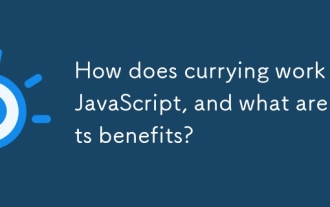
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
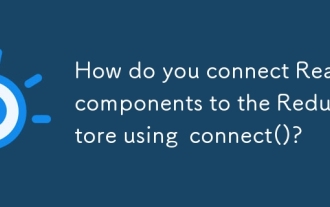
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
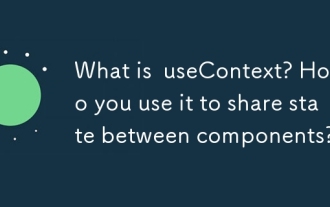
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
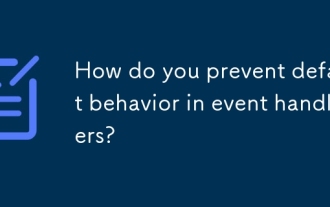
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
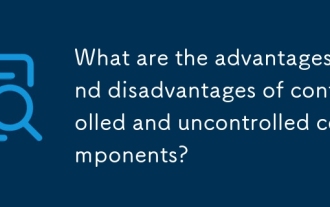
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
