How do you use variables in stored procedures and functions?
How do you use variables in stored procedures and functions?
Variables in stored procedures and functions are used to store temporary data that can be manipulated within the scope of the procedure or function. They enhance the flexibility and reusability of these SQL objects by allowing you to process data dynamically. Here's how you can use them:
-
Declaration: Variables must be declared at the beginning of the procedure or function using the
DECLARE
statement. The syntax isDECLARE @VariableName DataType;
. For example,DECLARE @CustomerName VARCHAR(100);
. -
Assignment: After declaration, you can assign values to variables using the
SET
orSELECT
statements. For example,SET @CustomerName = 'John Doe';
orSELECT @CustomerName = Name FROM Customers WHERE ID = 1;
. -
Usage: Variables can be used in any part of the SQL code within the procedure or function where a value of the same type is expected. For example,
SELECT * FROM Orders WHERE CustomerName = @CustomerName;
. - Scope: Variables declared in a stored procedure or function are local to that specific procedure or function. They cannot be accessed outside their defined scope.
-
Output: In stored procedures, you can use the
OUTPUT
parameter to return the value of a variable back to the caller. For example,CREATE PROCEDURE GetCustomerName @ID INT, @Name VARCHAR(100) OUTPUT AS BEGIN SELECT @Name = Name FROM Customers WHERE ID = @ID; END
.
What are the best practices for declaring variables in SQL stored procedures?
Declaring variables in SQL stored procedures should follow these best practices to ensure code readability, maintainability, and performance:
-
Use Meaningful Names: Choose variable names that clearly represent the data they hold. This improves code readability and maintenance. For example, use
@TotalPrice
instead of@TP
. - Specify Data Types: Always specify the data type of the variable. This prevents data type mismatches and enhances performance by allowing the SQL engine to optimize operations.
-
Initialize Variables: It's good practice to initialize variables at the time of declaration or immediately after to prevent the use of unintended values. For example,
DECLARE @Count INT = 0;
. - Avoid Global Variables: Global variables can lead to unexpected behavior and make the code harder to debug. Use local variables within procedures and functions.
- Document Usage: Use comments to explain the purpose of variables, especially if their use isn't immediately obvious. This helps other developers understand the code.
- Minimize Variable Usage: Only declare variables that are necessary. Excessive use of variables can clutter the code and impact performance.
How can variables enhance the functionality of SQL functions?
Variables can significantly enhance the functionality of SQL functions in several ways:
- Dynamic Data Handling: Variables allow functions to process and return dynamic data based on input parameters. For example, a function can return different results based on a date range passed as variables.
- Complex Calculations: Variables can store intermediate results of complex calculations within functions, making the logic easier to follow and maintain.
- Reusability: By using variables, you can write functions that can be reused in multiple contexts, reducing the need for duplicate code.
- Error Handling: Variables can be used to capture and process error conditions within functions, allowing for more robust error handling and reporting.
- Performance Optimization: Using variables to store frequently accessed data can reduce the number of database queries, thereby improving performance.
For example, consider a function that calculates the average sales for a given period:
CREATE FUNCTION GetAverageSales (@StartDate DATE, @EndDate DATE) RETURNS DECIMAL(10,2) AS BEGIN DECLARE @TotalSales DECIMAL(18,2) = 0; DECLARE @TotalDays INT = DATEDIFF(DAY, @StartDate, @EndDate) 1; SELECT @TotalSales = SUM(SaleAmount) FROM Sales WHERE SaleDate BETWEEN @StartDate AND @EndDate; RETURN @TotalSales / @TotalDays; END;
What are common pitfalls to avoid when using variables in stored procedures?
When using variables in stored procedures, it's important to be aware of and avoid the following common pitfalls:
- Uninitialized Variables: Failing to initialize variables can lead to unexpected results. Always initialize variables to a default value.
- Data Type Mismatches: Ensure that the data type of the variable matches the data type of the column or value it is being assigned. Mismatches can lead to conversion errors or data loss.
- Overuse of Variables: Using too many variables can make the code harder to read and maintain. Only use variables where necessary.
- Scope Confusion: Variables have a local scope within stored procedures. Be careful not to confuse local variables with parameters or global variables, which can lead to logical errors.
- Performance Issues: Overusing variables, especially in large loops, can degrade performance. Minimize variable usage where possible.
- Not Handling NULL Values: Variables can be assigned NULL values, which may cause issues if not handled properly. Always check for NULL values when necessary.
- Ignoring Transactional Behavior: Variables do not participate in transactions. Changes to variables are not rolled back if a transaction is rolled back, which can lead to inconsistencies.
By being mindful of these pitfalls, you can write more robust and efficient stored procedures that effectively leverage variables.
The above is the detailed content of How do you use variables in stored procedures and functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


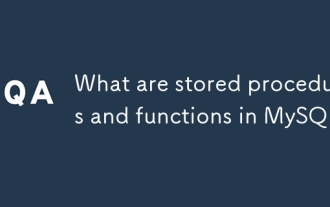
The article discusses stored procedures and functions in MySQL, focusing on their definitions, performance benefits, and usage scenarios. Key differences include return values and invocation methods.
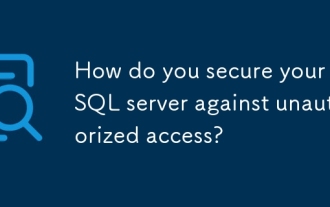
The article discusses securing MySQL servers against unauthorized access through password management, limiting remote access, using encryption, and regular updates. It also covers monitoring and detecting suspicious activities to enhance security.
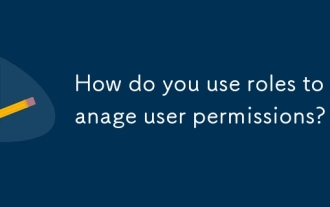
The article discusses using roles to manage user permissions efficiently, detailing role definition, permission assignment, and dynamic adjustments. It emphasizes best practices for role-based access control and how roles simplify user management acr
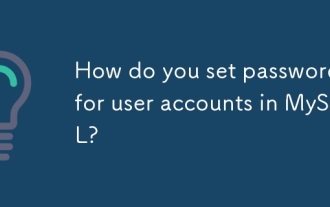
The article discusses methods for setting and securing MySQL user account passwords, best practices for password security, remote password changes, and ensuring compliance with password policies.
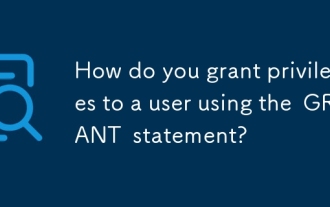
The article explains the use of the GRANT statement in SQL to assign various privileges like SELECT, INSERT, and UPDATE to users or roles on specific database objects. It also covers revoking privileges with the REVOKE statement and granting privileg
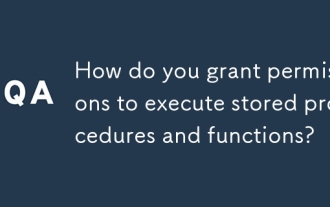
Article discusses granting execute permissions on stored procedures and functions, focusing on SQL commands and best practices for secure, multi-user database management.
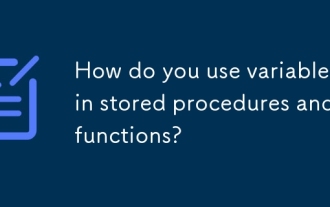
The article discusses using variables in SQL stored procedures and functions to enhance flexibility and reusability, detailing declaration, assignment, usage, scope, and output. It also covers best practices and common pitfalls to avoid when using va
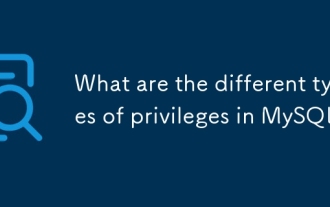
Article discusses MySQL privileges: global, database, table, column, routine, and proxy user types. It explains granting, revoking privileges, and best practices for secure management. Over-privileging risks are highlighted.
