How do you work with XML in Go?
How do you work with XML in Go?
Working with XML in Go involves primarily using the standard library's encoding/xml
package. This package provides the necessary tools for both encoding and decoding XML data. Here's a brief overview of how you can work with XML in Go:
-
Decoding XML:
To decode XML data into Go structs, you first define a struct that matches the structure of the XML. You then use thexml.Unmarshal
function to parse the XML data into the struct. For example:type Person struct { XMLName xml.Name `xml:"person"` Name string `xml:"name"` Age int `xml:"age"` } func main() { xmlData := `<person><name>John Doe</name><age>30</age></person>` var p Person err := xml.Unmarshal([]byte(xmlData), &p) if err != nil { fmt.Println(err) return } fmt.Printf("Name: %s, Age: %d\n", p.Name, p.Age) }
Copy after login Encoding XML:
To encode a Go struct into XML, you define a struct and use thexml.Marshal
function to convert it to XML. For example:type Person struct { XMLName xml.Name `xml:"person"` Name string `xml:"name"` Age int `xml:"age"` } func main() { p := Person{Name: "John Doe", Age: 30} output, err := xml.MarshalIndent(p, "", " ") if err != nil { fmt.Println(err) return } fmt.Println(string(output)) }
Copy after login
These examples illustrate the fundamental ways to handle XML in Go using the encoding/xml
package.
What are the best practices for parsing XML files in Go?
When parsing XML files in Go, following best practices can help you write more robust and maintainable code. Here are some key best practices:
Define Clear Structs:
Ensure that your structs accurately represent the XML structure. Use struct tags to map XML elements and attributes correctly. For example:type Person struct { XMLName xml.Name `xml:"person"` Name string `xml:"name"` Age int `xml:"age"` Email string `xml:"email,attr"` }
Copy after loginError Handling:
Always handle errors returned byxml.Unmarshal
. This helps in diagnosing and handling issues related to malformed XML or incorrect struct definitions.err := xml.Unmarshal([]byte(xmlData), &p) if err != nil { fmt.Println("Error unmarshaling XML:", err) return }
Copy after login- Use XML Schema:
If possible, use XML Schema definitions to validate your XML files before parsing. This can prevent errors and improve the reliability of your application. - Performance Considerations:
For large XML files, consider parsing the file in chunks or using streaming techniques to avoid loading the entire file into memory at once. - Validation:
Validate the parsed data against your expectations to ensure data integrity. For example, check if certain fields are mandatory or if numeric fields fall within expected ranges.
How can you efficiently encode Go structs to XML?
Efficiently encoding Go structs to XML involves using the encoding/xml
package and following certain practices to optimize performance and clarity. Here are some strategies:
Use
xml.MarshalIndent
:
For better readability, usexml.MarshalIndent
instead ofxml.Marshal
. It adds indentation to the output, which can be useful for debugging and human-readable output.output, err := xml.MarshalIndent(p, "", " ")
Copy after login- Avoid Nested Structs When Possible:
Simplifying your struct hierarchy can improve the efficiency of encoding. If you can flatten your structs without losing necessary information, do so. Use Tags Wisely:
Use struct tags to control how fields are encoded. For example, you can usexml:"omitempty"
to skip fields with zero values.type Person struct { XMLName xml.Name `xml:"person"` Name string `xml:"name"` Age int `xml:"age,omitempty"` }
Copy after login-
Batch Processing:
When encoding multiple structs, consider batching them into a single call toxml.Marshal
to avoid repeated function calls and improve performance. -
Use Streaming:
For very large datasets, consider using streaming techniques to encode structs. This can help manage memory usage and improve performance.
What libraries should you use for handling XML in Go applications?
In Go, the standard encoding/xml
package is the primary library for handling XML and is recommended for most use cases due to its simplicity and efficiency. However, there are additional libraries that you might consider for more specialized tasks:
-
encoding/xml:
The standard library'sencoding/xml
package is the go-to choice for general XML handling in Go. It provides robust support for both encoding and decoding XML. -
github.com/beevik/etree:
Theetree
library offers an alternative approach to working with XML, providing an element tree API similar to Python'slxml
. It can be useful for tasks requiring more complex manipulation of XML structures. -
github.com/antchfx/xmlquery:
xmlquery
is a library that allows you to query XML using XPath expressions. This can be very useful for extracting specific pieces of data from large or complex XML documents. -
github.com/clbanning/mxj:
mxj
is another XML library that supports both marshaling and unmarshaling of XML and JSON. It can be helpful if you need to convert between these formats frequently.
When choosing a library, consider the specific requirements of your project. For most use cases, encoding/xml
should suffice, but specialized libraries can provide additional features that might be necessary for more advanced XML handling tasks.
The above is the detailed content of How do you work with XML in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




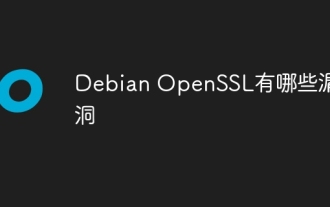
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
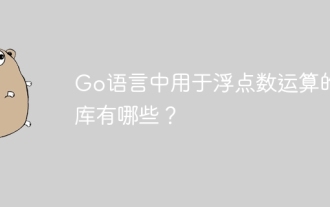
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
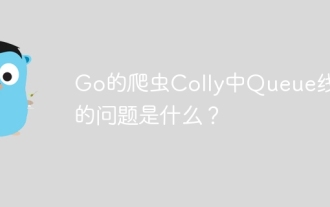
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
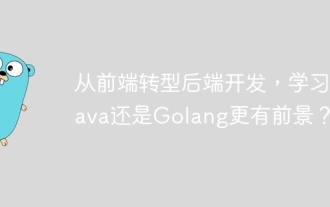
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
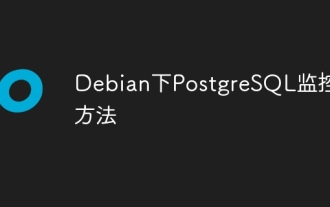
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
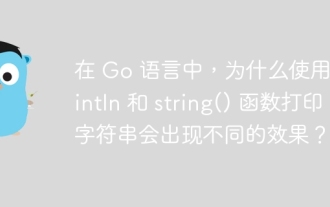
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
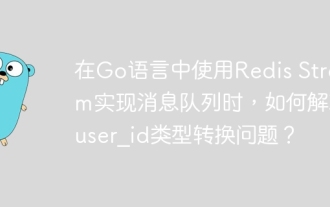
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
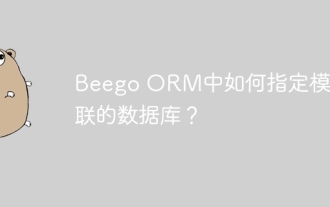
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
