How do you start, manage, and destroy sessions in PHP?
How do you start, manage, and destroy sessions in PHP?
Starting, managing, and destroying sessions in PHP involves several key functions and practices.
Starting a Session:
To start a session in PHP, you use the session_start()
function. This function must be called before any output is sent to the browser. It initializes a new session or resumes an existing one based on a session ID passed via a GET or POST request, or passed via a cookie.
session_start();
Managing a Session:
Once a session is started, you can store data in the session using the $_SESSION
superglobal array. Here's an example:
$_SESSION['username'] = 'john_doe'; $_SESSION['last_activity'] = time();
To access or modify session data, you continue to use the $_SESSION
array:
echo $_SESSION['username']; $_SESSION['last_activity'] = time();
Destroying a Session:
To destroy a session, you can use several methods depending on your needs:
Unset Session Variables:
To clear all session variables, useunset($_SESSION)
.unset($_SESSION);
Copy after loginCopy after loginDestroy the Session:
To destroy the session data on the server, usesession_destroy()
. This does not unset the session variables.session_destroy();
Copy after loginCopy after loginComplete Session Termination:
To completely terminate a session, combine the above methods and unset the session cookie:session_unset(); session_destroy(); setcookie(session_name(), '', time() - 3600, '/');
Copy after login
What are the best practices for securely managing sessions in PHP?
Securing session management in PHP involves several best practices:
- Use HTTPS:
Always use HTTPS to encrypt the data sent between the client and server, including session IDs. This prevents session hijacking through man-in-the-middle attacks. Regenerate Session IDs:
Usesession_regenerate_id(true)
to generate a new session ID when a user logs in or their privilege level changes. This helps to prevent session fixation attacks.session_regenerate_id(true);
Copy after loginCopy after loginSet Proper Session Configuration:
Adjust session configuration settings inphp.ini
or usingini_set()
function:session.cookie_httponly = 1
: Prevents client-side JavaScript from accessing the session cookie.session.cookie_secure = 1
: Ensures the session cookie is only sent over HTTPS.session.use_only_cookies = 1
: Ensures session IDs are only passed via cookies, not via URL parameters.
- Validate and Sanitize Session Data:
Always validate and sanitize session data to prevent injection attacks. Use PHP's built-in functions likefilter_var()
andhtmlspecialchars()
. - Implement Session Timeout:
Set a session timeout usingsession.gc_maxlifetime
to automatically terminate inactive sessions. - Use a Secure Session Handler:
Consider using a custom session handler or a library that provides more security features, such asmemcached
orredis
for storing session data. - Monitor and Log Session Activity:
Implement logging to monitor session activity and detect potential security issues.
How can you ensure session data integrity in PHP applications?
Ensuring session data integrity in PHP applications involves several strategies:
- Validate and Sanitize Data:
Always validate and sanitize data before storing it in the session. Use PHP's built-in functions likefilter_var()
,htmlspecialchars()
, andintval()
to ensure data integrity. - Use Serialized Objects:
Instead of storing raw data, use serialized objects to encapsulate data and methods, ensuring that the data is only modified through the object's methods. Implement Data Encryption:
Encrypt sensitive session data using PHP's OpenSSL functions or a library likelibsodium
. This adds an extra layer of protection if session data is compromised.$data = 'sensitive_data'; $encrypted = openssl_encrypt($data, 'AES-256-CBC', 'secret_key', 0, 'iv'); $_SESSION['encrypted_data'] = $encrypted;
Copy after loginImplement Checksums:
Use a checksum or hash to verify the integrity of session data. Store a hash of the data along with the data itself, and verify it upon retrieval.$data = 'some_data'; $checksum = hash('sha256', $data); $_SESSION['data'] = $data; $_SESSION['checksum'] = $checksum; // Later, verify the checksum if (hash('sha256', $_SESSION['data']) === $_SESSION['checksum']) { // Data is intact }
Copy after login- Use a Secure Session Handler:
Implement a custom session handler that can store session data in a secure manner, such as usingmemcached
orredis
with appropriate security configurations.
What methods are available for destroying a session in PHP to prevent session fixation attacks?
To prevent session fixation attacks, you need to ensure that the session ID is regenerated and the old session is destroyed. Here are the methods available for destroying a session in PHP:
Regenerate Session ID:
When a user logs in or their privilege level changes, usesession_regenerate_id(true)
to generate a new session ID and destroy the old session. This is crucial for preventing session fixation attacks.session_regenerate_id(true);
Copy after loginCopy after loginUnset Session Variables:
Clear all session variables usingunset($_SESSION)
to ensure no data from the old session remains.unset($_SESSION);
Copy after loginCopy after loginDestroy the Session:
Usesession_destroy()
to destroy the session data on the server.session_destroy();
Copy after loginCopy after loginUnset Session Cookie:
Unset the session cookie to prevent any future access to the session:setcookie(session_name(), '', time() - 3600, '/');
Copy after loginComplete Session Termination:
Combine the above methods for a complete session termination:session_unset(); session_destroy(); session_regenerate_id(true); setcookie(session_name(), '', time() - 3600, '/');
Copy after loginBy following these methods, you can effectively destroy a session and prevent session fixation attacks, ensuring the security of your PHP application.
The above is the detailed content of How do you start, manage, and destroy sessions in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
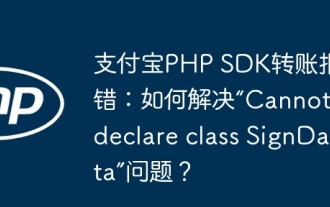
Alipay PHP...
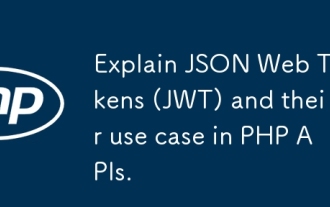
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
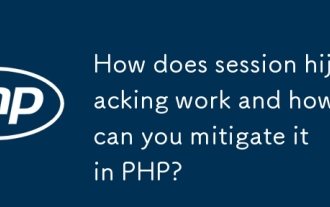
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
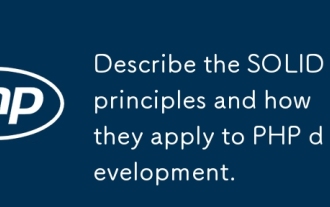
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
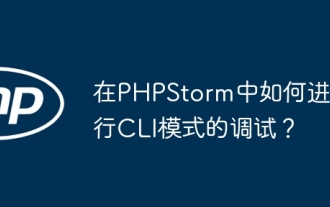
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
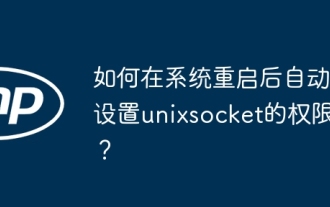
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
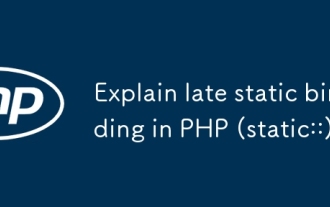
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
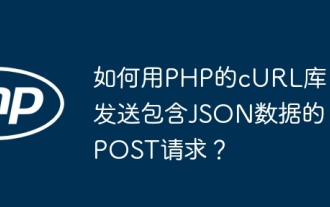
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
