Explain how cookies work in PHP.
Explain how cookies work in PHP
Cookies are small pieces of data stored on a user's computer by the web browser while browsing a website. In PHP, cookies are used to manage session data, store user preferences, and facilitate a more personalized user experience.
When a PHP script wants to set a cookie, it sends a Set-Cookie
header to the user's browser, which includes the cookie's name, value, expiration time, path, domain, and security options. Once the browser receives this header, it saves the cookie according to the specified parameters. On subsequent requests to the same domain, the browser automatically sends the cookie back to the server in the Cookie
header.
PHP can then access the cookie data using the $_COOKIE
superglobal array. This allows PHP scripts to read the values of cookies sent by the browser and use them for various purposes, such as maintaining session state or remembering user settings.
What are the common uses of cookies in PHP web applications?
Cookies serve several common purposes in PHP web applications:
- Session Management: Cookies are often used to store a unique session ID, allowing the server to link a user's actions across multiple page requests. This is crucial for maintaining a user's login state or shopping cart contents.
- User Preferences: Cookies can save user preferences such as language, theme, or other customizable settings, ensuring a more tailored user experience on return visits.
- Tracking: Websites use cookies to track user behavior, such as pages visited or actions taken, to improve the user experience, personalize content, or analyze site performance.
- Authentication: Cookies can store tokens or authentication information, allowing users to remain logged in across different pages or sessions without needing to re-enter their credentials.
- Personalization: E-commerce sites might use cookies to remember items a user has recently viewed or added to a wish list, facilitating easier access to these items on subsequent visits.
How do you set and retrieve cookies in PHP?
Setting a cookie in PHP is done using the setcookie()
function. Here's an example:
// Set a cookie that expires in one hour setcookie('username', 'JohnDoe', time() 3600, '/');
In this example:
'username'
is the cookie name.'JohnDoe'
is the cookie value.time() 3600
sets the expiration time to one hour from now.'/'
specifies the path on the server where the cookie will be available.
To retrieve a cookie, PHP provides the $_COOKIE
superglobal array. You can access the value of a cookie by its name:
// Retrieve the value of the 'username' cookie $username = $_COOKIE['username'] ?? null;
In this example, $_COOKIE['username']
retrieves the value of the 'username' cookie. The null coalescing operator ??
is used to provide a default value (null
) if the cookie doesn't exist.
What are the security considerations when using cookies in PHP?
Using cookies in PHP comes with several security considerations:
- Data Sensitivity: Avoid storing sensitive data in cookies, such as passwords or credit card numbers, as they are vulnerable to interception.
Secure Flag: Use the
secure
flag to ensure cookies are only sent over HTTPS. This helps prevent man-in-the-middle attacks:setcookie('username', 'JohnDoe', time() 3600, '/', '', true); // 'true' sets the secure flag
Copy after loginHttpOnly Flag: Set the
httpOnly
flag to prevent client-side scripts from accessing the cookie, reducing the risk of cross-site scripting (XSS) attacks:setcookie('username', 'JohnDoe', time() 3600, '/', '', true, true); // 'true' sets the httpOnly flag
Copy after login- Cookie Tampering: Validate and sanitize cookie data to prevent tampering. Use cryptographic signing or hashing to ensure the integrity of the data.
- Expiration: Set appropriate expiration times for cookies. Session cookies (without an expiration time) can be more secure than persistent cookies.
- Domain and Path Restrictions: Set appropriate domain and path values to limit the scope of the cookie, reducing the risk of exposure to unintended parts of your site or other sites.
SameSite Attribute: Use the
SameSite
attribute to specify whether and how cookies are sent with cross-origin requests, mitigating cross-site request forgery (CSRF) attacks:setcookie('username', 'JohnDoe', time() 3600, '/', '', true, true, 'Lax'); // 'Lax' sets the SameSite attribute
Copy after loginBy following these security practices, you can help protect your users' data and enhance the security of your PHP applications that use cookies.
The above is the detailed content of Explain how cookies work in PHP.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
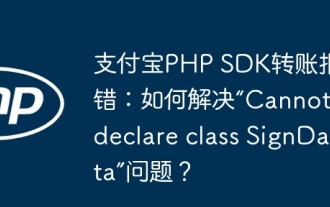
Alipay PHP...
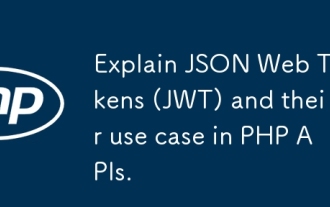
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
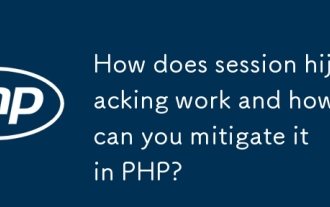
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
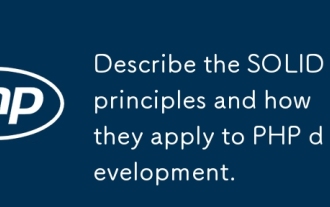
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
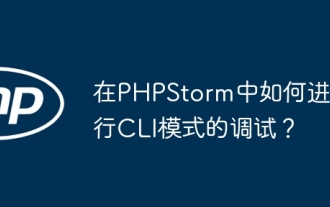
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
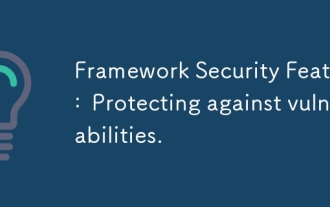
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
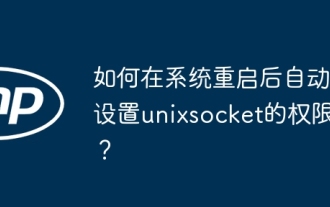
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
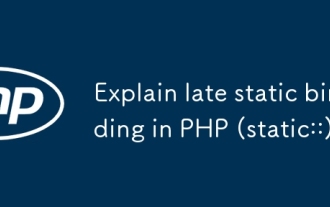
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
