How do you set, retrieve, and delete cookies in PHP?
How do you set, retrieve, and delete cookies in PHP?
Setting Cookies:
In PHP, you can set a cookie using the setcookie()
function. This function must be called before any output is sent to the browser. Here's how you can set a cookie:
setcookie("cookie_name", "cookie_value", time() 3600, "/");
In this example:
"cookie_name"
is the name of the cookie."cookie_value"
is the value of the cookie.time() 3600
sets the expiration time to one hour from now."/"
specifies that the cookie is available across the entire domain.
Retrieving Cookies:
To retrieve a cookie, you can access the $_COOKIE
superglobal array:
$cookie_value = $_COOKIE['cookie_name'];
This will return the value of the cookie named cookie_name
.
Deleting Cookies:
To delete a cookie, you need to set its expiration time in the past:
setcookie("cookie_name", "", time() - 3600, "/");
This sets the expiration time to one hour ago, which effectively deletes the cookie.
How can I ensure the security of cookies when using PHP?
Ensuring the security of cookies is crucial when working with PHP. Here are some practices to enhance cookie security:
Use HTTPS:
Always use HTTPS to encrypt the data transmitted between the client and the server. This prevents man-in-the-middle attacks where someone could intercept and modify the cookie data.
setcookie("cookie_name", "cookie_value", time() 3600, "/", "", true, true);
The last two arguments true, true
set the secure
and httponly
flags respectively.
Secure Flag:
The secure
flag ensures that the cookie is only sent over HTTPS connections.
HttpOnly Flag:
The httponly
flag prevents client-side scripts (like JavaScript) from accessing the cookie, which helps mitigate the risk of cross-site scripting (XSS) attacks.
Validate and Sanitize:
Always validate and sanitize cookie data before using it. This helps prevent injection attacks.
if (isset($_COOKIE['cookie_name'])) { $cookie_value = filter_var($_COOKIE['cookie_name'], FILTER_SANITIZE_STRING); }
Use Short Lifespan:
Set cookies to have a short lifespan to reduce the window of opportunity for an attacker to use a stolen cookie.
Use Cookie Prefixes:
Use the __Secure-
and __Host-
prefixes to ensure cookies are only sent over secure channels and to restrict their path and domain.
What are the best practices for managing session data with cookies in PHP?
Managing session data with cookies in PHP involves several best practices to ensure efficiency and security:
Use PHP Sessions:
PHP provides a built-in session management system that uses cookies to track session IDs. This is the recommended way to manage session data.
session_start(); $_SESSION['user_id'] = $user_id;
Regenerate Session ID:
To prevent session fixation attacks, regenerate the session ID after a successful login.
session_regenerate_id(true);
Use Secure Session Cookies:
Ensure that the session cookie is set with the secure
and httponly
flags.
session_set_cookie_params(0, '/', '', true, true); session_start();
Store Session Data on the Server:
Store sensitive session data on the server rather than in the cookie itself. Only the session ID should be stored in the cookie.
Session Timeout:
Set an appropriate session timeout to balance user convenience with security.
ini_set('session.gc_maxlifetime', 3600); // 1 hour session_start();
Validate Session Data:
Always validate and sanitize session data before using it to prevent injection attacks.
if (isset($_SESSION['user_id'])) { $user_id = filter_var($_SESSION['user_id'], FILTER_SANITIZE_NUMBER_INT); }
What common issues should I be aware of when working with cookies in PHP?
When working with cookies in PHP, you should be aware of several common issues:
Output Before Cookie Setting:
Cookies must be set before any output is sent to the browser. If you try to set a cookie after sending output, you will get a "headers already sent" error.
Cookie Size Limitations:
Browsers have limits on the size of cookies. The total size of all cookies sent to a domain is typically limited to around 4KB. Be mindful of this when storing data in cookies.
Cross-Site Scripting (XSS):
If cookies are accessible to client-side scripts, they can be vulnerable to XSS attacks. Always use the httponly
flag to mitigate this risk.
Session Fixation:
If an attacker can set a user's session ID before they log in, they can hijack the session after login. Always regenerate the session ID after login.
Cookie Tampering:
Users can tamper with cookie data on the client side. Always validate and sanitize cookie data on the server side.
Privacy Concerns:
Users may disable cookies or clear them, which can affect your application's functionality. Provide alternative methods for maintaining state when cookies are unavailable.
Time Zone Differences:
Be aware of time zone differences when setting expiration times for cookies. Use UTC or server time consistently.
By understanding and addressing these common issues, you can effectively work with cookies in PHP and enhance the security and reliability of your applications.
The above is the detailed content of How do you set, retrieve, and delete cookies in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


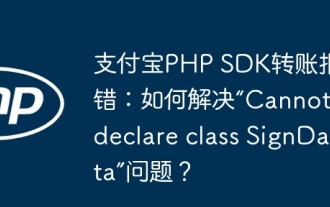
Alipay PHP...
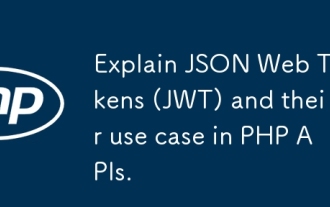
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
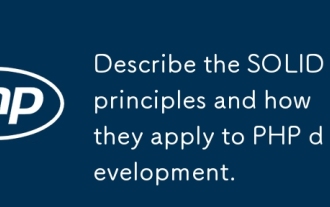
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
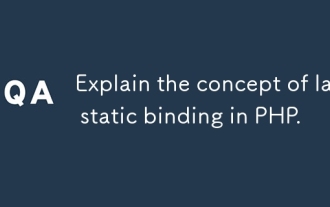
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
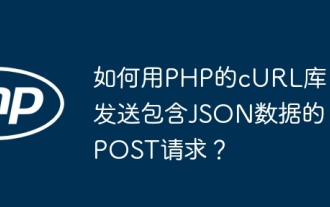
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
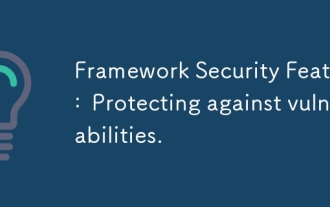
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
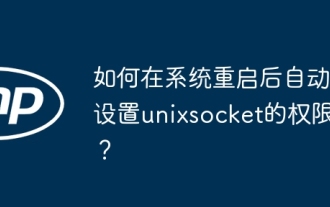
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
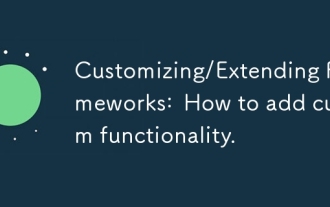
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
