Responsible Markdown in Next.js
Markdown is indeed a great format. It's close enough to plain text that anyone can learn quickly, and it's well structured enough to be parsed and eventually converted to whatever format you want.
However: parsing, processing, enhancing, and converting Markdown requires code. Deploying all this code on the client comes at a price. It's not huge in itself, but it's still a few dozen KB of code that's only used to handle Markdown and no other purpose.
In this article, I'll explain how to keep Markdown out of the client in a Next.js application, using the Unified/Remark ecosystem (I really don't know which name to use, which is too confusing).
Main ideas
The idea is to use only Markdown in the getStaticProps
function in Next.js to do this during the build process (if you use Vercel's incremental build, it is done in the Next serverless function), but it is by no means used on the client side. I guess getServerSideProps
is OK, too, but I think getStaticProps
is more likely to be a common use case.
This returns an AST generated by parsing and processing Markdown content ( abstract syntax tree , that is, a large nested object that describes our content), and the client is only responsible for rendering the AST into a React component.
I guess we can even render Markdown as HTML directly in getStaticProps
and return it to render with dangerouslySetInnerHtml
, but we are not that kind of people. Safety is important. Also, the flexibility to render Markdown the way we want with our own components instead of rendering it as pure HTML. Seriously, friends, don't do that. ?
export const getStaticProps = async () => { // Get Markdown content from somewhere, such as CMS or something. As far as this article is concerned, this is not important. It can also be read from the file. const markdown = await getMarkdownContentFromSomewhere() const ast = parseMarkdown(markdown) return { props: { ast } } } const Page = props => { // This usually includes your layout and so on, but it's omitted here for simplicity. Return<markdownrenderer ast="{props.ast}"></markdownrenderer> } export default Page
Analyze Markdown
We will use the Unified/Remark ecosystem. We need to install unified and remark-parse, that's it. It is relatively simple to parse Markdown itself:
import { unified } from 'unified' import remarkParse from 'remark-parse' const parseMarkdown = content => unified().use(remarkParse).parse(content) export default parseMarkdown
Now, what took me a long time to understand is why my extra plugins like remark-prism or remark-slug don't work like this. This is because the Unified .parse(..)
method does not handle AST using the plugin. As the name implies, it only parses the Markdown string content into a tree.
If we want Unified to apply our plugins, we need Unified to go through what they call the "running" phase. Typically, this is done by using .process(..)
method instead of .parse(..)
method. Unfortunately, .process(..)
not only parses Markdown and applies plugins, it also strings the AST into another format (for example, using HTML via remark-html, or using JSX via remark-react). And that's not what we want because we want to keep the AST, but after it's processed by the plugin.
<code>| ........................ process ........................... | | .......... parse ... | ... run ... | ... stringify ..........| -------- ----------输入->- | 解析器| ->- 语法树->- | 编译器| ->- 输出-------- | ---------- X | -------------- | 变换器| --------------</code>
Therefore, all we need to do is run the parsing and run phases, but not the stringization phase. Unified does not provide a method to execute two of these three stages, but it provides a separate method for each stage so we can do it manually:
import { unified } from 'unified' import remarkParse from 'remark-parse' import remarkPrism from 'remark-prism' const parseMarkdown = content => { const engine = unified().use(remarkParse).use(remarkPrism) const ast = engine.parse(content) // Unified's *process* contains three different stages: parsing, running, and stringification. We don't want to go through the stringization phase because we want to keep AST so we can't call `.process(..)`. However, calling `.parse(..)` is not enough, because the plugin (and therefore Prism) is executed during the run phase. So we need to call the run phase manually (for simplicity, synchronously). // See: https://github.com/unifiedjs/unified#description return engine.runSync(ast) }
Look! We parsed Markdown into a syntax tree. We then run our plugin on that tree (it is done synchronously for simplicity, but you can do it asynchronously using .run(..)
). However, we did not convert our tree to other syntaxes such as HTML or JSX. We can do it ourselves in rendering.
Render Markdown
Now that we have our cool tree ready, we can render it as we intend. Let's create a MarkdownRenderer
component that takes the tree as ast
property and renders it with the React component.
const getComponent = node => { switch (node.type) { case 'root': return ({ children }) => {children}> case 'paragraph': return ({ children }) =><p> {children}</p> case 'emphasis': return ({ children }) => <em>{ children}</em> case 'heading': return ({ children, depth = 2 }) => { const Heading = `h${depth}` Return<heading> {children}</heading> } case 'text': return ({ value }) => {value}> /* Handle all types here... */ default: console.log('Unprocessed node type', node) return ({ children }) => {children}> } } const Node = ({ node }) => { const Component = getComponent(node) const { children } = node return children ? ( <component> {children.map((child, index) => ( <node key="{index}" node="{child}"></node> ))} </component> ) : ( <component></component> ) } const MarkdownRenderer = ({ ast }) =><node node="{ast}"></node> export default React.memo(MarkdownRenderer)
Most of the logic of our renderer is located in Node
component. It finds out what to render based on type
key of the AST node (this is our getComponent
method deals with each type of node) and then renders it. If the node has children, it recursively enters the child node; otherwise, it only renders the component as the final leaf node.
Clean the tree
Depending on the Remark plugin we are using, we may encounter the following issues when trying to render the page:
Error: An error occurred while serializing .content[0].content.children[3].data.hChildren[0].data.hChildren[0].data.hChildren[0].data.hChildren[0].data.hName (from getStaticProps in '/'). Cause: undefined cannot be serialized to JSON. Please use null or omit this value.
This happens because our AST contains keys with undefined values, which is not something that can be safely serialized to JSON. Next gives us a solution: we can omit the value altogether, or replace it with null if we need it more or less.
However, we won't fix each path manually, so we need to recursively traverse that AST and clean it up. I found this happens when using remark-prism (a plugin that enables code block syntax highlighting). The plugin does add a [data]
object to the node.
What we can do is iterate over it to clean up these nodes before returning AST:
const cleanNode = node => { if (node.value === undefined) delete node.value if (node.tagName === undefined) delete node.tagName if (node.data) { delete node.data.hName delete node.data.hChildren delete node.data.hProperties } if (node.children) node.children.forEach(cleanNode) return node } const parseMarkdown = content => { const engine = unified().use(remarkParse).use(remarkPrism) const ast = engine.parse(content) const processedAst = engine.runSync(ast) cleanNode(processedAst) return processedAst }
The last thing we can do is delete the position
object that exists on each node, which holds the original position in the Markdown string. It is not a large object (it has only two keys), but it accumulates quickly when the tree gets bigger.
const cleanNode = node => { delete node.position // ...Other cleaning logic}
Summarize
That's it! We managed to limit Markdown processing to build/server side code, so we don't send unnecessary Markdown runtimes to the browser, which unnecessarily increases costs. We pass the data tree to the client, and we can iterate over it and convert it into any React component we want.
Hope this helps. :)
The above is the detailed content of Responsible Markdown in Next.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
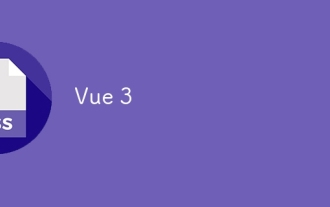
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
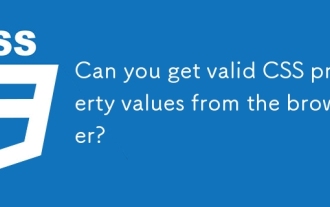
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
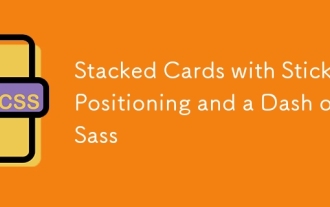
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
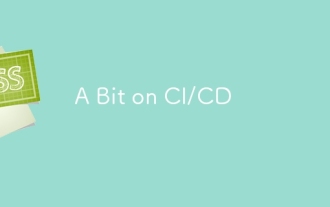
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
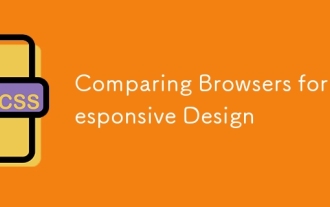
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
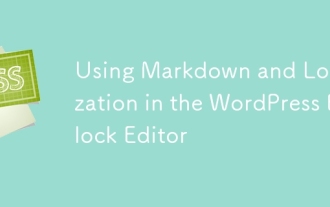
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
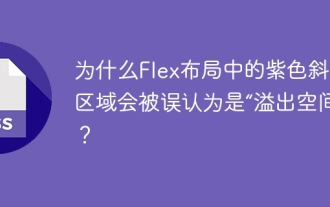
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
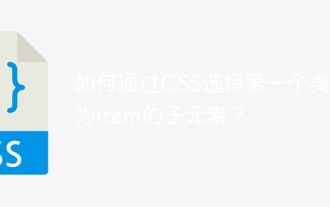
When the number of elements is not fixed, how to select the first child element of the specified class name through CSS. When processing HTML structure, you often encounter different elements...
