What is list comprehension in Python?
What is list comprehension in Python?
List comprehension in Python is a concise and powerful way to create lists based on existing lists or other iterables. It allows you to combine loops and conditional statements into a single, readable line of code. The basic syntax for a list comprehension is:
new_list = [expression for item in iterable if condition]
Here's how it works:
- Expression: This is what you want to do with each
item
in theiterable
. It could be a simple operation, like multiplying the item by 2, or a more complex operation. - Iterable: This is the source of the data you are working with, such as a list, tuple, or any iterable object.
- Condition (optional): This is used to filter the items from the
iterable
. If the condition is true for an item, theexpression
is applied to it.
Here is an example of list comprehension:
# Traditional way numbers = [1, 2, 3, 4, 5] squared_numbers = [] for num in numbers: squared_numbers.append(num ** 2) # Using list comprehension squared_numbers = [num ** 2 for num in numbers]
Both methods achieve the same result, but list comprehension does so more succinctly.
How can list comprehension improve the efficiency of my Python code?
List comprehension can improve the efficiency of your Python code in several ways:
- Concise Code: List comprehension allows you to write fewer lines of code, which can make your code easier to read and maintain. This reduction in code length can also mean fewer opportunities for errors.
- Performance: List comprehensions are often faster than traditional for loops because they are optimized at the C level in CPython, the default Python implementation. They create and return the list in one pass, which can be more efficient than building the list incrementally.
- Memory Usage: List comprehensions are more memory-efficient for smaller lists because they avoid the need to create a separate list object to hold intermediate results. However, for very large lists, traditional loops might be preferable because list comprehensions create the entire list in memory at once.
- Expressiveness: They can clearly express the intent of the code, making it easier for other developers (or yourself in the future) to understand what the code is meant to do.
Here's an example comparing the performance:
import timeit # List comprehension list_comp_time = timeit.timeit('[x**2 for x in range(1000)]', number=10000) print(f"List comprehension time: {list_comp_time}") # Traditional for loop for_loop_time = timeit.timeit(''' numbers = [] for x in range(1000): numbers.append(x**2) ''', number=10000) print(f"For loop time: {for_loop_time}")
Running this code often shows that list comprehension is faster.
What are some common use cases for list comprehension in Python programming?
List comprehensions are versatile and can be used in many scenarios. Some common use cases include:
Transforming Lists: You can use list comprehensions to transform elements of a list. For example, converting a list of strings to uppercase:
original_list = ["apple", "banana", "cherry"] upper_list = [fruit.upper() for fruit in original_list]
Copy after loginFiltering Lists: You can filter elements based on a condition. For example, selecting only even numbers from a list:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [num for num in numbers if num % 2 == 0]
Copy after loginCreating Lists from Other Iterables: List comprehensions can create lists from other iterables like tuples or sets:
tuple_data = (1, 2, 3, 4, 5) new_list = [x * 2 for x in tuple_data]
Copy after loginNested List Comprehensions: You can use nested list comprehensions to flatten a list of lists or perform more complex operations:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] flat_list = [num for row in matrix for num in row]
Copy after loginConditional Logic: You can incorporate conditional logic to apply different transformations based on conditions:
numbers = [1, -2, 3, -4, 5] absolute_values = [abs(num) if num < 0 else num for num in numbers]
Copy after login
Can list comprehension be used with other data structures in Python besides lists?
While the term "list comprehension" specifically refers to creating lists, the concept can be extended to other data structures in Python. Here's how you can use similar syntax with other data structures:
Set Comprehensions: Set comprehensions use curly braces
{}
instead of square brackets[]
and return a set:numbers = [1, 2, 3, 4, 5, 5, 6] unique_squares = {x**2 for x in numbers}
Copy after loginDictionary Comprehensions: Dictionary comprehensions create dictionaries. They use curly braces
{}
and a colon:
to separate keys and values:original_dict = {'a': 1, 'b': 2, 'c': 3} doubled_dict = {key: value * 2 for key, value in original_dict.items()}
Copy after loginGenerator Expressions: Generator expressions are similar to list comprehensions but use parentheses
()
instead of square brackets[]
. They generate values on-the-fly and do not store them in memory:numbers = [1, 2, 3, 4, 5] squares_gen = (x**2 for x in numbers) for square in squares_gen: print(square)
Copy after loginWhile list comprehensions are specifically for lists, these related constructs allow you to use similar syntax for other data structures, improving code readability and efficiency in a similar manner.
The above is the detailed content of What is list comprehension in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
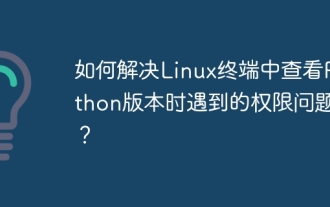
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
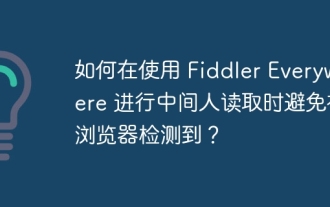
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
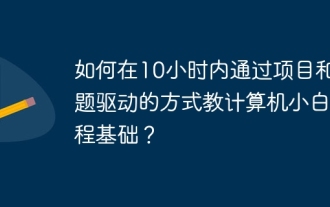
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
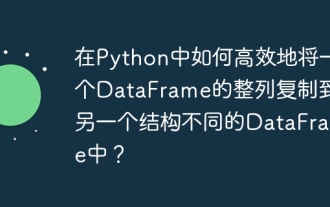
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
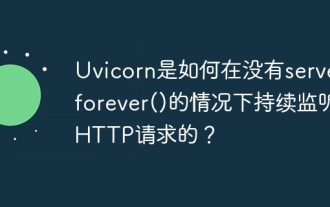
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
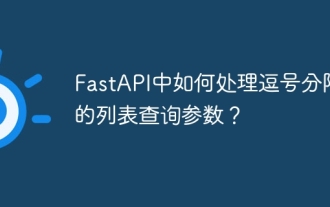
Fastapi ...
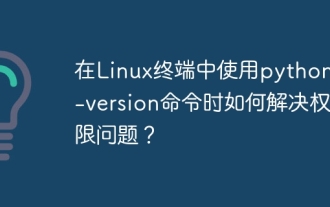
Using python in Linux terminal...
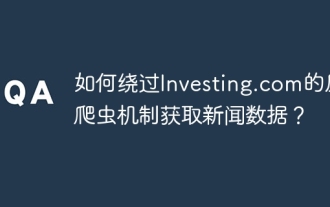
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
