How do you write unit tests in Go?
How do you write unit tests in Go?
Writing unit tests in Go is straightforward due to the built-in testing
package. Here's a step-by-step approach to writing unit tests:
-
Create a Test File: For a source file named
foo.go
, create a test file namedfoo_test.go
in the same package. -
Write Test Functions: Inside
foo_test.go
, write functions that start withTest
followed by the name of the function being tested. These functions take a*testing.T
argument. For example:func TestFoo(t *testing.T) { // Test code here }
Copy after login Assertions: Use
t.Error
ort.Errorf
to log errors and fail the test.t.Fatal
ort.Fatalf
can be used to stop the test immediately if something critical fails.if result != expected { t.Errorf("expected %v, but got %v", expected, result) }
Copy after login- Run Tests: Execute tests using the
go test
command in the terminal from the directory containing the test files. - Test Coverage: To get test coverage, run
go test -cover
. Table-Driven Tests: Use a table-driven approach to reduce code duplication and test multiple scenarios efficiently.
func TestFoo(t *testing.T) { tests := []struct { input int expected int }{ {1, 2}, {2, 4}, {-1, -2}, } for _, tt := range tests { result := foo(tt.input) if result != tt.expected { t.Errorf("foo(%d) = %d, want %d", tt.input, result, tt.expected) } } }
Copy after login
What are the best practices for writing effective unit tests in Go?
Adhering to best practices in writing unit tests in Go can significantly improve the quality and maintainability of your tests. Here are some key practices:
- Keep Tests Simple and Focused: Each test should cover one specific behavior or scenario. This makes tests easier to understand and maintain.
- Use Descriptive Names: Name your test functions and variables clearly to convey their purpose. For example,
TestFooReturnsDoubleOfInput
is more descriptive thanTestFoo
. - Utilize Helper Functions: If you find yourself repeating the same setup or assertion logic across multiple tests, move it to a helper function to reduce duplication.
- Test for Error Conditions: Do not only test happy paths; also test how your function behaves with invalid inputs or in error conditions.
Parallel Testing: Use
t.Parallel()
to run tests in parallel, which can significantly speed up your test suite, especially for larger projects.func TestFoo(t *testing.T) { t.Parallel() // Test code here }
Copy after login- Mock Dependencies: Use mocking to isolate the function being tested from external dependencies, allowing for more controlled and reliable tests.
- Achieve High Test Coverage: Aim for high test coverage, but remember that 100% coverage is not always necessary. Focus on critical paths and edge cases.
- Continuous Integration: Integrate your tests into a CI/CD pipeline to ensure tests run automatically on every code change.
How can you use mocking in Go to improve your unit tests?
Mocking in Go can help isolate the unit under test by replacing its dependencies with controlled, fake objects. Here's how you can utilize mocking to enhance your unit tests:
Choose a Mocking Library: Popular Go mocking libraries include
GoMock
,testify/mock
, andgomock
. For example, withtestify/mock
:import ( "testing" "github.com/stretchr/testify/mock" ) type MockDependency struct { mock.Mock } func (m *MockDependency) SomeMethod(input string) string { args := m.Called(input) return args.String(0) }
Copy after login- Create Mock Objects: Define mock objects that mimic the interface of the dependency you wish to mock. Set up expected behavior for these mocks before running the test.
Setup Expectations: Before executing the function under test, set up the expected behavior of the mock using the library’s API.
mockDependency := new(MockDependency) mockDependency.On("SomeMethod", "input").Return("output")
Copy after login- Run the Test: Execute the function you're testing with the mock dependency. The mock will behave as predefined.
Assert Mock Calls: After the test, verify that the mock was called as expected.
mockDependency.AssertCalled(t, "SomeMethod", "input")
Copy after login- Benefits: Mocking allows you to test the behavior of your code in isolation, making tests faster and more reliable. It also allows testing of error scenarios without affecting real services.
What tools can help you manage and run unit tests efficiently in Go?
Several tools can help you manage and run unit tests efficiently in Go. Here’s a list of some popular ones:
-
Go Test Command: The built-in
go test
command is versatile and can be used with various flags to customize the test execution. For example,go test -v
for verbose output orgo test -coverprofile=coverage.out
to generate a coverage report. -
Ginkgo: An advanced testing framework that offers a more readable DSL and better support for integration and end-to-end testing. It can be used in conjunction with
Gomega
for matchers and assertions. -
Testify: A popular suite of test-related packages that includes
require
for test assertions,assert
for fluent assertions,suite
for test organization, andmock
for mocking dependencies. - GoConvey: An alternative testing framework that provides a more interactive and visual way of writing tests and viewing results, which is particularly useful for TDD.
- Delve: A debugger for Go that can be used to step through tests, helping you diagnose and resolve issues more efficiently.
- Coveralls: A tool that can be integrated into your CI pipeline to track and report on test coverage over time, helping to ensure your test suite remains effective.
- CircleCI, Travis CI, or GitHub Actions: These continuous integration services can automate the running of your tests, providing immediate feedback on the health of your codebase after every commit.
Using these tools in combination can help streamline your testing process, improve test coverage, and make your test suite more maintainable and efficient.
The above is the detailed content of How do you write unit tests in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


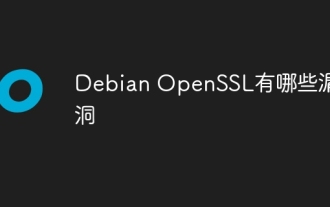
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
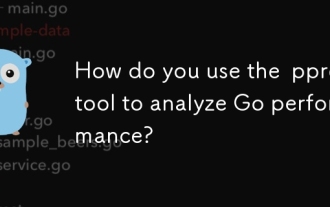
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
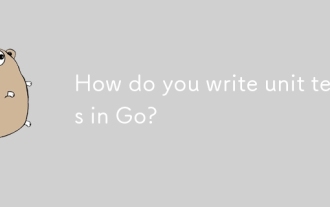
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
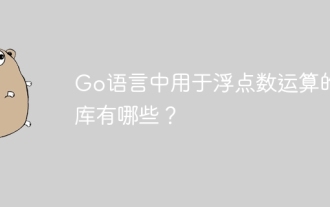
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
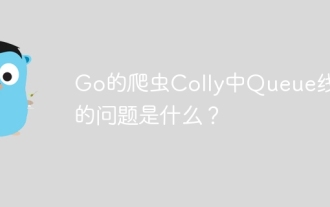
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
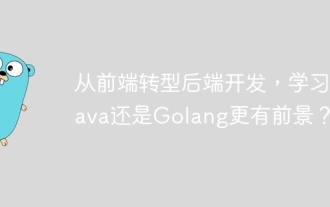
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
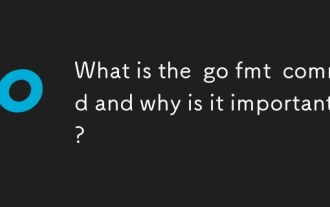
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
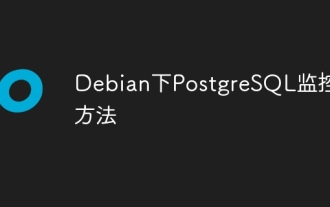
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
