PHP require vs include vs require_once: When to use each?
Mar 25, 2025 am 10:24 AMPHP require vs include vs require_once: When to use each?
In PHP, require
, include
, and require_once
are used to include and evaluate the specified file during the execution of a script. The choice between these statements depends on the specific needs of your application. Here is a detailed explanation of when to use each:
-
include: The
include
statement includes and evaluates the specified file. If the file is not found, a warning will be issued and the script will continue execution. It is generally used when the file is not essential for the script to continue running. This makes it ideal for including optional components or templates.include 'optional_file.php';
Copy after login require: The
require
statement is similar toinclude
, but it treats the file as essential for the script's execution. If the file is not found, a fatal error will be issued, and the script execution will halt. Userequire
for files that are critical to the operation of your script, such as configuration files or core libraries.require 'critical_file.php';
Copy after loginrequire_once: The
require_once
statement is identical torequire
except PHP will check if the file has already been included, and if so, it will not include it again. This is particularly useful for including files that define functions or classes that should not be redefined, helping to prevent function redefinition errors.require_once 'library_file.php';
Copy after login
What are the performance implications of using require_once over require?
The performance implications of using require_once
over require
can be significant, especially in larger applications:
- Additional Overhead:
require_once
has more overhead thanrequire
because it needs to check if the file has already been included before including it. This involves maintaining a list of included files in memory, which consumes more resources. - Execution Time: In scripts where multiple files are included, using
require_once
can increase the execution time because of the additional checks. This can be particularly noticeable in large applications with many includes. - Memory Usage: The internal tracking mechanism of
require_once
requires additional memory, although the impact might be negligible unless your application includes a very large number of files. - Best Practices: If you are certain that a file will not be included more than once, using
require
instead ofrequire_once
can save some processing overhead. However, if you are unsure or it's critical to ensure a file is included only once (to avoid function redefinition errors, for example),require_once
should be used despite the performance cost.
How does the error handling differ between include and require in PHP?
The primary difference in error handling between include
and require
in PHP is the severity of the error and how it impacts the script execution:
include: If
include
cannot find the specified file, PHP will issue a warning (E_WARNING) but will continue executing the script. This allows the rest of the script to run, potentially with altered behavior if the included file was important but not critical.include 'non_existent_file.php'; // Continues execution with a warning echo "This will still be executed.";
Copy after loginrequire: If
require
cannot find the specified file, PHP will issue a fatal error (E_COMPILE_ERROR) and halt the script execution. This ensures that the script does not proceed if a critical file is missing, which can be crucial for maintaining the integrity of your application.require 'non_existent_file.php'; // Stops execution with a fatal error echo "This will not be executed.";
Copy after login
In what scenarios would using include be more appropriate than require or require_once?
Using include
is more appropriate in the following scenarios:
Non-critical Content: When including files that contain non-essential content, such as HTML templates or optional feature modules,
include
is more suitable. If these files are missing, the application can still function with a warning.include 'optional_template.php'; // Non-critical HTML template
Copy after loginDynamic Inclusion: When you need to include files conditionally or based on user input,
include
is preferred because it allows the script to continue even if the file is missing.if ($condition) { include 'conditional_file.php'; }
Copy after login- Testing and Development: During the development phase,
include
can be useful for testing how the script behaves when certain files are missing, allowing developers to see warnings instead of fatal errors. Logging and Monitoring: Including files that contain logging or monitoring functions where the absence of the file should not prevent the main application from running.
include 'logging_functions.php'; // Non-critical logging
Copy after login
In these scenarios, include
helps in maintaining the flexibility and robustness of your application by allowing it to gracefully handle missing files without stopping execution.
The above is the detailed content of PHP require vs include vs require_once: When to use each?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
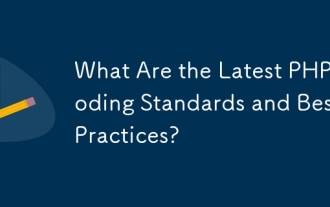
What Are the Latest PHP Coding Standards and Best Practices?
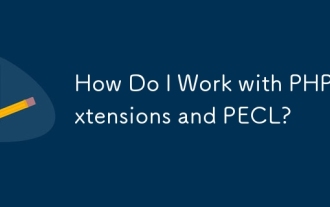
How Do I Work with PHP Extensions and PECL?
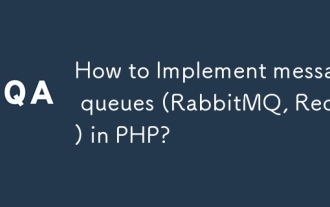
How to Implement message queues (RabbitMQ, Redis) in PHP?
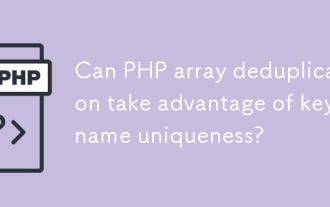
Can PHP array deduplication take advantage of key name uniqueness?
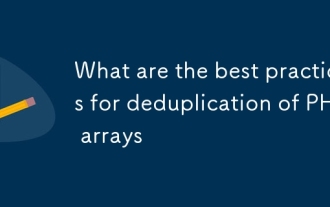
What are the best practices for deduplication of PHP arrays
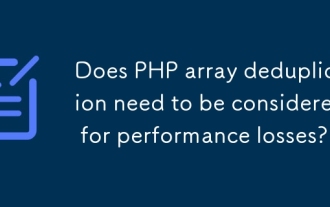
Does PHP array deduplication need to be considered for performance losses?
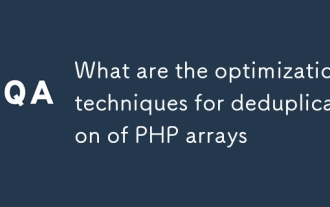
What are the optimization techniques for deduplication of PHP arrays
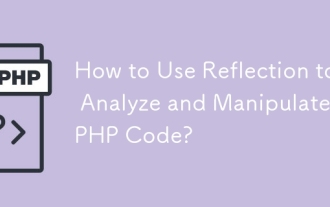
How to Use Reflection to Analyze and Manipulate PHP Code?
