


What is SFINAE (Substitution Failure Is Not An Error)? How is it used in template metaprogramming?
Mar 25, 2025 pm 02:48 PMWhat is SFINAE (Substitution Failure Is Not An Error)? How is it used in template metaprogramming?
SFINAE, which stands for "Substitution Failure Is Not An Error," is a principle in C template metaprogramming that dictates that if substitution of template parameters into a function declaration fails, it does not result in a compilation error, but rather causes that particular specialization to be removed from the overload resolution set. This technique is commonly used to control which function template specializations are considered during overload resolution.
In template metaprogramming, SFINAE is used to selectively enable or disable function overloads based on certain conditions, typically involving the type traits of the template arguments. This is done by using expressions that are valid for some types but not for others within the template's declaration, often in default template arguments or function parameter types.
For example, consider a generic function that is supposed to work with types that have a specific member function. You can use SFINAE to ensure that the function compiles only when the type does indeed have that member function:
template<typename T> auto foo(T t) -> decltype(t.memberFunction(), void(), std::true_type{}) { t.memberFunction(); return std::true_type{}; } template<typename T> std::false_type foo(T t) { return std::false_type{}; }
In this example, the first foo
function will be selected by overload resolution only if T
has a member function called memberFunction
. Otherwise, the second foo
function, which always compiles, will be used.
How can SFINAE improve the flexibility of C template functions?
SFINAE significantly enhances the flexibility of C template functions by allowing developers to write more generic code that can adapt to different types at compile-time. This adaptability is achieved by enabling and disabling different function overloads based on the properties of the types involved, resulting in more robust and reusable code.
One key way SFINAE improves flexibility is by allowing the creation of generic interfaces that can behave differently based on the capabilities of the types involved. For instance, consider a template function that may need to use different algorithms depending on whether a type provides certain member functions or operators. SFINAE allows such a function to seamlessly adapt:
template<typename T> auto sort(T& container) -> decltype(container.sort(), void(), std::true_type{}) { container.sort(); } template<typename T> void sort(T& container) { std::sort(container.begin(), container.end()); }
In this case, if T
has a sort
member function, the first overload will be chosen, leveraging the type's own sorting mechanism. If not, the second overload using the standard library's std::sort
will be used instead.
By using SFINAE, developers can create more expressive and adaptable APIs that are easier to use correctly and harder to misuse.
What are common pitfalls to avoid when implementing SFINAE in C ?
When implementing SFINAE in C , there are several common pitfalls to be aware of and avoid:
- Inadvertent Ambiguity: When creating multiple SFINAE-based overloads, it's possible to end up with overloads that are ambiguous for certain types, leading to compilation errors. Always ensure that the overloads are clearly differentiated based on their enabling conditions.
- Unintended Substitution Failures: Sometimes, the conditions for SFINAE might trigger on cases you didn't anticipate, leading to unexpected behaviors. Thoroughly test your SFINAE conditions with a variety of types to ensure they behave as intended.
- Overuse of SFINAE: While SFINAE is a powerful tool, overusing it can make the code harder to read and maintain. Use it judiciously, and consider alternatives like tag dispatching or explicit template specializations when they might be clearer or more appropriate.
- Not Handling All Cases: Make sure you have a fallback or default case to handle situations where none of your SFINAE-enabled overloads match. This is usually achieved by having a non-templated function that serves as a catch-all.
- Misunderstanding the Substitution Context: Remember that SFINAE applies during template argument substitution, and not during the body of the function. Only expressions in function declarations, return types, and default argument values are considered for SFINAE.
Can SFINAE be used to achieve function overloading in C templates?
Yes, SFINAE can indeed be used to achieve function overloading in C templates. It allows the compiler to selectively discard certain template specializations during overload resolution, effectively enabling or disabling them based on the properties of the types involved.
The classic example of using SFINAE for function overloading is creating generic functions that have different implementations based on whether certain operations are available for the argument types. Consider the example of a toString
function that converts a value to a string in different ways depending on the available operations:
#include <string> #include <sstream> template<typename T> std::string toString(T value, std::enable_if_t<std::is_arithmetic_v<T>, int> = 0) { std::ostringstream oss; oss << value; return oss.str(); } template<typename T> std::string toString(T value, std::enable_if_t<!std::is_arithmetic_v<T>, int> = 0) { return value.toString(); // Assumes T has a toString member function }
In this example, the first toString
function will be used for arithmetic types (like int
and double
), while the second will be used for types that have a toString
member function. The std::enable_if_t
construct leverages SFINAE to enable or disable each function overload based on the std::is_arithmetic_v<t></t>
trait.
By carefully crafting the SFINAE conditions, developers can create rich, type-aware function overloads that allow for more flexible and generic programming.
The above is the detailed content of What is SFINAE (Substitution Failure Is Not An Error)? How is it used in template metaprogramming?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
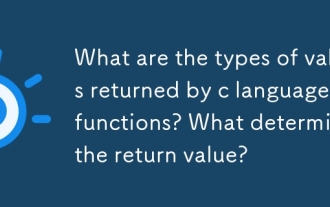
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
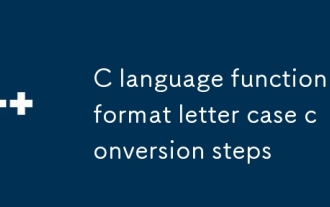
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
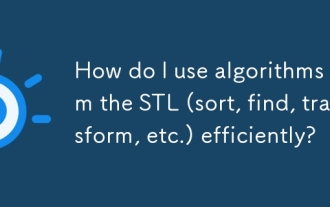
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
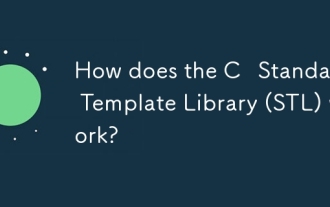
How does the C Standard Template Library (STL) work?
