Explain the purpose of enable_if in template metaprogramming.
Mar 25, 2025 pm 02:49 PMExplain the purpose of enable_if in template metaprogramming.
enable_if
is a utility in C template metaprogramming that allows you to enable or disable specific function overloads or template instantiations based on compile-time conditions. It's part of the <type_traits></type_traits>
header and is used to conditionally remove functions from the overload resolution set. This is particularly useful in generic programming where you want to provide different implementations of a function based on traits or conditions of the template arguments.
The general syntax of enable_if
is std::enable_if<condition t>::type</condition>
, where condition
is a boolean expression that is evaluated at compile-time. If the condition is true, std::enable_if<condition t>::type</condition>
will be defined as T
; otherwise, it will not be defined, effectively removing the function from consideration during overload resolution.
The purpose of enable_if
is to allow the programmer to select different implementations of a function based on compile-time conditions, which can lead to cleaner and more expressive code. It's especially useful for implementing SFINAE (Substitution Failure Is Not An Error) techniques, which are fundamental in advanced C template metaprogramming.
How does enable_if help in selecting specific function overloads?
enable_if
helps in selecting specific function overloads through the SFINAE principle. When the compiler tries to instantiate a template, it will attempt to resolve the function overloads. If an enable_if
condition within a function signature evaluates to false, the substitution of the template parameters into the function signature will fail, and that function will be removed from the overload resolution set.
This allows you to define multiple overloads of a function with different enable_if
conditions, and the compiler will pick the function whose enable_if
condition evaluates to true. This is particularly useful when you want to provide different implementations of a function based on the properties of the template arguments.
For instance, if you have a generic function that you want to behave differently for types that support a specific operation (like addition), you can use enable_if
to enable the function only for types that satisfy this condition.
What are the common use cases for enable_if in C template programming?
-
Overload Selection Based on Type Traits: One of the most common uses of
enable_if
is to select function overloads based on type traits. For instance, you might want to provide a specialized implementation of a function for arithmetic types, and a different one for other types. -
Conditional Template Specialization:
enable_if
can be used to conditionally enable or disable template specializations based on the properties of the template arguments. -
Function Enabling/Disabling Based on Compile-Time Conditions: You can use
enable_if
to enable or disable a function based on any compile-time condition, not just type traits. This can be useful in metaprogramming scenarios where you need to control the availability of a function based on complex conditions. -
Implementing Concepts-Like Behavior in C 03/C 11: Before the introduction of Concepts in C 20,
enable_if
was often used to simulate concept-like behavior, where you define interfaces that a type must satisfy to be used with certain functions or templates. -
Preventing Unwanted Implicit Conversions:
enable_if
can be used to prevent unwanted implicit conversions by disabling functions that would otherwise be candidates for overload resolution.
Can you provide an example where enable_if improves code readability and functionality?
Here's an example that demonstrates how enable_if
can be used to improve both readability and functionality by providing different implementations of a function for different types:
#include <iostream> #include <type_traits> template<typename T> typename std::enable_if<std::is_arithmetic<T>::value, T>::type add(T a, T b) { return a b; } template<typename T> typename std::enable_if<!std::is_arithmetic<T>::value, T>::type add(T a, T b) { // Assuming T has an operator defined return a b; } int main() { int a = 5, b = 3; std::cout << "Arithmetic addition: " << add(a, b) << std::endl; std::string s1 = "Hello, ", s2 = "World!"; std::cout << "String concatenation: " << add(s1, s2) << std::endl; return 0; }
In this example, the add
function is overloaded to provide different implementations based on whether the template argument T
is an arithmetic type or not. For arithmetic types, it performs arithmetic addition, and for other types (like strings), it uses the
operator to perform concatenation.
Using enable_if
here improves readability and functionality by clearly separating the two cases and ensuring that the correct operation is performed based on the type of the arguments. This approach also keeps the interface clean, as the user of the add
function does not need to know about the implementation details; the correct version is automatically selected by the compiler.
The above is the detailed content of Explain the purpose of enable_if in template metaprogramming.. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
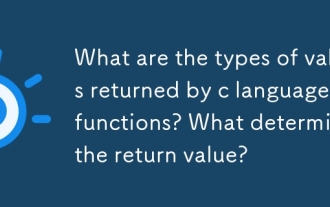
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
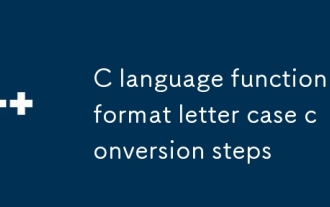
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
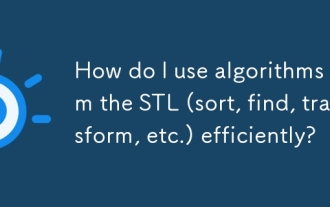
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
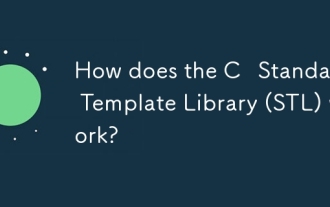
How does the C Standard Template Library (STL) work?
