PHP Authentication & Authorization: Secure implementation.
How can I implement robust authentication in PHP to prevent unauthorized access?
Implementing robust authentication in PHP involves several steps to ensure that only authorized users can access your application. Here's a detailed approach to achieving this:
- Use HTTPS: Always serve your application over HTTPS to encrypt data between the client and server. This prevents man-in-the-middle attacks that could compromise user credentials.
-
Password Hashing: Use PHP's
password_hash()
function to securely hash passwords before storing them in your database. When a user attempts to log in, usepassword_verify()
to check the provided password against the stored hash.$password = 'userpassword'; $hash = password_hash($password, PASSWORD_BCRYPT); // When verifying: if (password_verify($password, $hash)) { // Password is correct } else { // Password is incorrect }
Copy after login Session Management: Use PHP sessions to manage user states after authentication. Ensure you regenerate the session ID after successful authentication to prevent session fixation attacks.
session_start(); if (isset($_POST['username']) && isset($_POST['password'])) { // Authentication logic here if (/*user authenticated*/) { session_regenerate_id(true); $_SESSION['logged_in'] = true; $_SESSION['user_id'] = $user_id; } }
Copy after login- Implement Two-Factor Authentication (2FA): Add an extra layer of security by implementing 2FA. You can use tools like Google Authenticator or Authy to generate time-based one-time passwords (TOTP).
- Rate Limiting: Implement rate limiting on login attempts to prevent brute-force attacks. You can use a library like
symfony/rate-limiter
to manage this effectively. - Login Throttling: After a few failed login attempts, implement a delay or temporary account lockout to further mitigate brute-force attacks.
By following these practices, you can build a robust authentication system in PHP that safeguards against unauthorized access.
What are the best practices for managing user authorization securely in PHP applications?
Managing user authorization securely in PHP applications requires a structured approach to ensure that users have access only to the resources they are entitled to. Here are some best practices:
Role-Based Access Control (RBAC): Implement RBAC to assign permissions to roles rather than directly to users. This simplifies management and makes it easier to modify permissions as roles change.
class Role { private $permissions; public function __construct($permissions) { $this->permissions = $permissions; } public function hasPermission($permission) { return in_array($permission, $this->permissions); } } // Usage $adminRole = new Role(['create_user', 'delete_user', 'view_user']); if ($adminRole->hasPermission('create_user')) { // User can create users }
Copy after login- Least Privilege Principle: Ensure that users have the minimum level of access necessary to perform their job functions. Regularly audit and adjust permissions to maintain this principle.
- Attribute-Based Access Control (ABAC): For more granular control, use ABAC, which grants access based on user attributes, resource attributes, and environmental conditions. This can be more complex but offers fine-tuned access control.
- Session and Token Management: Use secure session management as discussed earlier. For APIs, implement token-based authentication with OAuth or JSON Web Tokens (JWT) to manage authorization securely.
- Logging and Monitoring: Log all authorization attempts and monitor these logs to detect and respond to suspicious activities promptly.
- Secure Storage of Permissions: Store user permissions securely in the database and ensure that these permissions are not tampered with. Use integrity checks where necessary.
By implementing these best practices, you can ensure that user authorization in your PHP application is managed securely and efficiently.
Can you recommend tools or libraries that enhance security for PHP authentication and authorization?
Several tools and libraries can enhance security for PHP authentication and authorization. Here are some recommendations:
-
Password Hashing:
-
PHP's
password_hash
andpassword_verify
: Built-in functions for secure password hashing and verification.
-
PHP's
-
Authentication Libraries:
- Delight\Auth: A comprehensive authentication library for PHP that supports secure password hashing, session management, and email-based password reset functionality.
- Firebase Authentication: For PHP applications that need to support multiple authentication methods, including social logins and 2FA.
-
Authorization Libraries:
- Symfony Security Component: Offers robust tools for managing authentication and authorization, including RBAC and ABAC support.
- Laravel Authorization: Provides a simple and powerful way to manage authorization using gates and policies.
-
Two-Factor Authentication:
- PHPGangsta/GoogleAuthenticator: A library for implementing Google Authenticator-based two-factor authentication.
- RobThree/TwoFactorAuth: Another option for implementing TOTP-based 2FA in PHP.
-
Session and Token Management:
- Firebase JWT: A library for working with JSON Web Tokens (JWT) in PHP, which can be used for API authentication and authorization.
-
OAuth 2.0 Client: Use libraries like
league/oauth2-client
to implement OAuth 2.0 for secure API access.
-
Security Auditing and Monitoring:
- OWASP PHP Security Project: Provides guidelines and tools for securing PHP applications.
- PHPStan: A PHP static analysis tool that can help detect security issues in your code.
By integrating these tools and libraries into your PHP application, you can significantly enhance the security of your authentication and authorization mechanisms.
The above is the detailed content of PHP Authentication & Authorization: Secure implementation.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


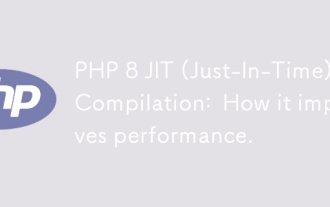
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
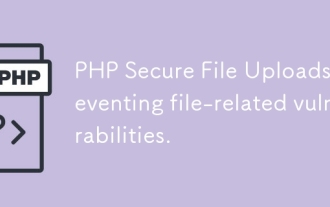
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
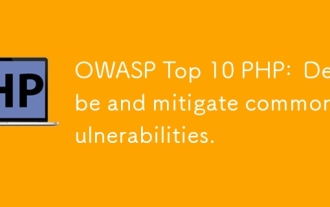
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
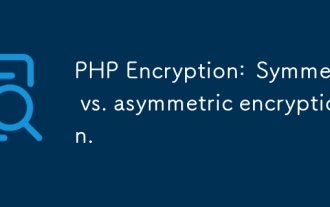
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
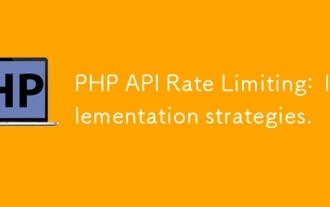
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
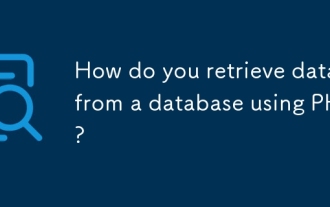
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
