


How can you use contexts in Go to manage goroutine cancellation and deadlines?
Mar 25, 2025 pm 03:44 PMHow can you use contexts in Go to manage goroutine cancellation and deadlines?
In Go, contexts are a powerful tool for managing goroutine lifecycle, especially for cancellation and deadline enforcement. The context
package in Go provides a way to pass request-scoped data, cancellation signals, and deadlines across API boundaries and between processes.
To use contexts for managing goroutine cancellation and deadlines, you start by creating a context. The context.Background()
or context.TODO()
functions are used to create a root context, which is then used to derive more specific contexts. For instance, to cancel a goroutine, you use context.WithCancel(parent)
to create a context that can be canceled. When you want to cancel all goroutines sharing this context, you call the cancel function returned by context.WithCancel
.
For handling deadlines, you use context.WithDeadline(parent, deadline)
or context.WithTimeout(parent, timeout)
to create a context that will automatically cancel after the specified deadline or timeout.
Here's a simple example of how you might use a context to manage a goroutine's lifecycle:
ctx, cancel := context.WithCancel(context.Background()) defer cancel() go func() { for { select { case <-ctx.Done(): fmt.Println("goroutine canceled") return default: // do something time.Sleep(1 * time.Second) } } }() // To cancel the goroutine cancel()
What are the benefits of using contexts for goroutine management in Go?
Using contexts for goroutine management in Go offers several benefits:
- Centralized Cancellation: Contexts allow you to centralize the cancellation of multiple goroutines. When you cancel a context, all goroutines watching that context will be notified and can gracefully shut down.
- Deadline Enforcement: Contexts enable you to enforce deadlines across operations. This is particularly useful in scenarios where operations should not run indefinitely, such as in HTTP servers or when dealing with external services.
- Request Scoping: Contexts help in passing request-scoped data and cancellation signals across different layers of your application, which is crucial in distributed systems and microservices.
- Simplified Code: By using contexts, you can simplify your code, especially when dealing with complex operations involving multiple goroutines. This leads to more maintainable and less error-prone code.
- Efficient Resource Management: Contexts help in managing resources efficiently by allowing you to clean up resources (like closing connections or freeing up memory) when operations are canceled or timed out.
How do you set and handle deadlines using contexts in Go?
Setting and handling deadlines using contexts in Go involves using the context.WithDeadline
or context.WithTimeout
functions. Here's how you can do it:
- Setting a Deadline: To set a deadline, you use
context.WithDeadline
. This function takes a parent context and a time value for the deadline. - Setting a Timeout: Alternatively, if you want to set a timeout instead of an absolute deadline, you use
context.WithTimeout
. This function takes a parent context and a duration.
Here's an example of setting a deadline and handling it:
ctx, cancel := context.WithDeadline(context.Background(), time.Now().Add(5*time.Second)) defer cancel() go func() { for { select { case <-ctx.Done(): fmt.Println("Deadline exceeded:", ctx.Err()) return default: // do something time.Sleep(1 * time.Second) } } }() // Wait for the goroutine to finish or the deadline to be reached time.Sleep(10 * time.Second)
In this example, the goroutine will run until the deadline is reached or it is canceled manually.
How can contexts help in propagating cancellation signals across multiple goroutines in Go?
Contexts in Go are particularly useful for propagating cancellation signals across multiple goroutines. When you create a context with context.WithCancel
, context.WithDeadline
, or context.WithTimeout
, you can share this context with multiple goroutines. When the context is canceled (either manually or due to a deadline being reached), all goroutines that are watching the context will be notified via the ctx.Done()
channel.
Here's how it works:
- Create a Context: Start by creating a context that can be canceled or has a deadline.
- Share the Context: Pass this context to multiple goroutines. Each goroutine should listen to
ctx.Done()
to know when it should stop running. - Cancel the Context: When you want to cancel all operations, call the cancel function associated with the context. All goroutines watching this context will receive the cancellation signal.
Here’s an example demonstrating this:
ctx, cancel := context.WithCancel(context.Background()) defer cancel() go func() { for { select { case <-ctx.Done(): fmt.Println("Goroutine 1 stopped") return default: fmt.Println("Goroutine 1 working") time.Sleep(1 * time.Second) } } }() go func() { for { select { case <-ctx.Done(): fmt.Println("Goroutine 2 stopped") return default: fmt.Println("Goroutine 2 working") time.Sleep(1 * time.Second) } } }() // Cancel after 3 seconds time.Sleep(3 * time.Second) cancel() // Wait for goroutines to finish time.Sleep(2 * time.Second)
In this example, both goroutines will stop after 3 seconds when the context is canceled. This demonstrates how contexts help in propagating cancellation signals effectively across multiple goroutines.
The above is the detailed content of How can you use contexts in Go to manage goroutine cancellation and deadlines?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
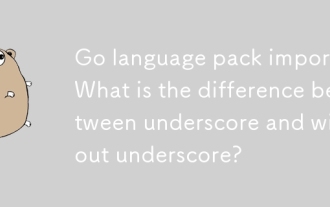
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
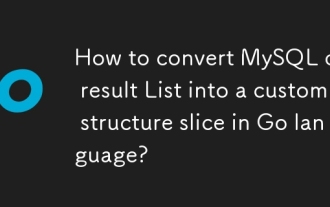
How to convert MySQL query result List into a custom structure slice in Go language?
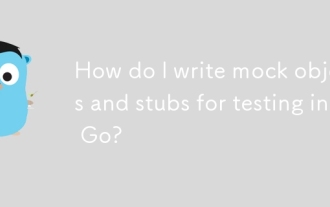
How do I write mock objects and stubs for testing in Go?
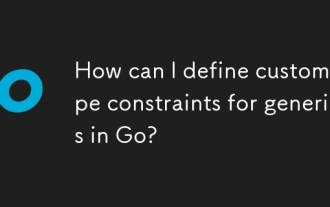
How can I define custom type constraints for generics in Go?
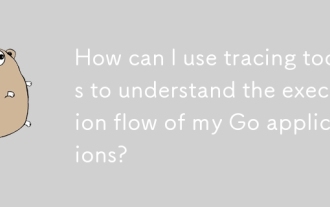
How can I use tracing tools to understand the execution flow of my Go applications?
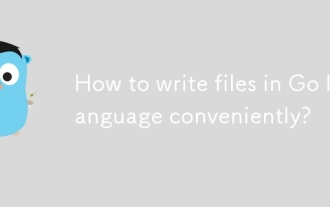
How to write files in Go language conveniently?
