


How do you write unit tests in Python using the unittest framework?
How do you write unit tests in Python using the unittest framework?
Writing unit tests in Python using the unittest
framework involves several steps. Below is a detailed guide to creating and running unit tests:
-
Import the unittest Module: The first step is to import the
unittest
module, which provides the framework for writing and running tests.import unittest
Copy after login Define a Test Class: Your tests will be grouped into classes that inherit from
unittest.TestCase
. This class will contain methods that define individual tests.class TestExample(unittest.TestCase):
Copy after loginWrite Test Methods: Inside the
TestExample
class, you can write methods that start with the wordtest
. These methods will run as individual tests.def test_example(self): self.assertEqual(1 1, 2)
Copy after loginSet Up and Tear Down: If your tests require any setup or cleanup, you can use
setUp
andtearDown
methods.setUp
runs before each test method, andtearDown
runs after.def setUp(self): # Code here will run before every test pass def tearDown(self): # Code here will run after every test pass
Copy after loginRun the Tests: To run the tests, you can either run the script directly if it contains the tests, or use a test runner. The simplest way is to add the following code at the end of your script:
if __name__ == '__main__': unittest.main()
Copy after login
When you run the script, unittest
will automatically discover and execute all methods starting with test
within classes that inherit from unittest.TestCase
.
What are the best practices for structuring unit tests with Python's unittest?
Adhering to best practices when structuring unit tests in Python's unittest
framework helps ensure tests are maintainable, readable, and effective. Here are key practices to follow:
- Test Naming Conventions: Use clear, descriptive names for your test classes and methods. For example,
TestCalculator
for a class andtest_addition
for a method. This helps quickly understand what each test is intended to verify. Arrange-Act-Assert Pattern: Structure your test methods using the Arrange-Act-Assert pattern:
- Arrange: Set up the conditions for the test.
- Act: Perform the action you want to test.
Assert: Verify the result.
def test_addition(self): # Arrange calc = Calculator() # Act result = calc.add(2, 3) # Assert self.assertEqual(result, 5)
Copy after login
- Isolate Tests: Ensure that each test is independent. Use
setUp
andtearDown
methods to manage test fixtures, ensuring each test starts with a clean slate. - Use setUp and tearDown Wisely: Use
setUp
to initialize objects andtearDown
to clean up resources if necessary. Avoid using them for actions that can be done inline with tests unless you find significant code duplication. - Group Related Tests: Group similar tests into the same test class to keep related functionality together, making your test suite more organized and easier to understand.
Use Descriptive Error Messages: When using assertions like
assertEqual
, you can add a custom message to clarify what went wrong, which is particularly useful when debugging failing tests.self.assertEqual(result, 5, "The addition of 2 and 3 should be 5")
Copy after login
How can you use assertions effectively in Python unittest to validate test results?
Assertions are crucial in unittest
to check if the output of your code meets the expected results. Here's how to use them effectively:
Choose the Right Assertion Method:
unittest
provides several assertion methods, each designed for specific comparisons:assertEqual(a, b)
: Checks ifa == b
.assertNotEqual(a, b)
: Checks ifa != b
.assertTrue(x)
: Checks ifx
is true.assertFalse(x)
: Checks ifx
is false.assertIs(a, b)
: Checks ifa
isb
(object identity).assertIsNot(a, b)
: Checks ifa
is notb
.assertIn(a, b)
: Checks ifa
is inb
.assertNotIn(a, b)
: Checks ifa
is not inb
.
Choose the assertion that best fits the test condition.
Use Custom Messages: For complex tests, it's helpful to provide a custom message to explain why the assertion failed.
self.assertEqual(result, 5, "Expected 5 but got {}".format(result))
Copy after loginTest for Edge Cases: Use assertions to validate not only the typical case but also edge cases and error conditions. For example, test for boundary conditions, invalid inputs, and expected exceptions.
def test_division_by_zero(self): with self.assertRaises(ZeroDivisionError): Calculator().divide(10, 0)
Copy after login- Avoid Over-Assertion: Don’t overdo assertions in a single test method. If you find yourself asserting multiple, unrelated things, it might be a sign that you should split the test into multiple methods.
Use Context Managers for Expected Exceptions: If you're expecting a specific exception, use the
assertRaises
context manager.with self.assertRaises(ValueError): Calculator().sqrt(-1)
Copy after login
What are common pitfalls to avoid when writing unit tests in Python using the unittest framework?
When writing unit tests with unittest
, it's helpful to be aware of common pitfalls to avoid in order to maintain high-quality tests:
- Testing Too Much in One Test: Avoid overloading a single test method with multiple assertions that test different functionalities. It's better to write separate tests for each piece of functionality.
- Not Testing Edge Cases: Neglecting to test for edge cases, such as empty inputs, maximum and minimum values, or error conditions, can leave your code vulnerable. Always think about the boundaries and unexpected inputs.
-
Overusing setUp and tearDown: While
setUp
andtearDown
are useful, overusing them can lead to test dependencies and slower tests. Use them only when necessary to set up test fixtures or clean up resources. - Ignoring Test Isolation: Each test should be independent. Sharing state between tests can lead to unpredictable results and make it hard to diagnose failures.
- Writing Tests After Code: Writing tests after the code can lead to tests that simply confirm the code works as-is rather than ensuring it behaves correctly under all conditions. Prefer writing tests before the code (Test-Driven Development, TDD).
- Not Updating Tests with Code Changes: As your code evolves, your tests need to evolve too. Failing to update tests to reflect changes in your code can lead to false negatives or false positives.
- Neglecting to Use Mocks and Stubs: For tests that depend on external resources or complex objects, not using mocks or stubs can make tests slow and brittle. Utilize mocking libraries to isolate dependencies.
- Writing Too Few Tests: Under-testing can leave critical parts of your code untested. Aim for a high coverage, especially for complex logic and edge cases.
By avoiding these pitfalls, you can ensure that your unit tests are robust, maintainable, and effectively validate the functionality of your code.
The above is the detailed content of How do you write unit tests in Python using the unittest framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


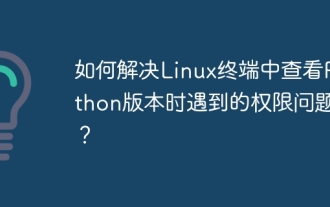
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
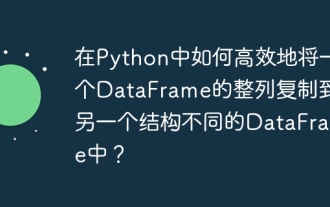
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
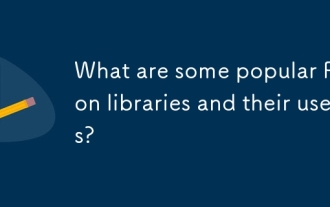
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
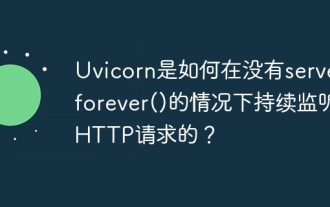
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
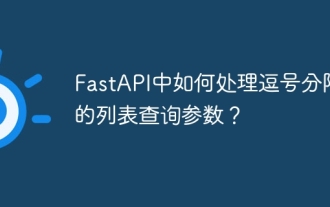
Fastapi ...
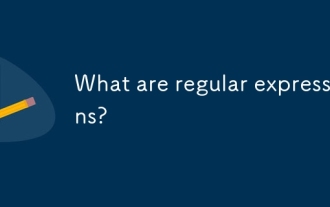
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
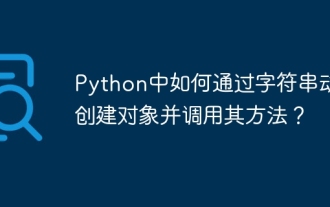
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
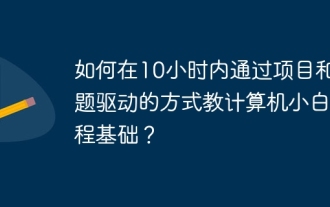
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
