


Explain the use of functools.lru_cache. How does it work, and when is it beneficial?
Explain the use of functools.lru_cache. How does it work, and when is it beneficial?
functools.lru_cache
is a decorator in Python's standard library that implements memoization, a technique where the results of expensive function calls are stored and reused when the same inputs occur again. This decorator implements a least-recently-used (LRU) cache, meaning that when the cache reaches its maximum size, the least recently used items are discarded to make room for new ones.
How it Works:
-
Function Call: When a function decorated with
lru_cache
is called, the decorator first checks if the arguments have been seen before. - Cache Hit: If the arguments are found in the cache (a cache hit), the cached result is returned instead of recalculating the result.
- Cache Miss: If the arguments are not found (a cache miss), the function is executed, the result is stored in the cache, and then returned.
- Cache Management: The LRU algorithm manages the cache size by discarding the least recently used items when the cache exceeds its maximum size.
When it is Beneficial:
- Expensive Computations: When a function performs expensive computations or operations like recursive algorithms (e.g., Fibonacci sequence) or database queries.
- Recurring Calls: When a function is called repeatedly with the same arguments.
- Performance Critical Sections: When performance improvements are crucial, such as in web applications where response times are important.
What specific performance improvements can be expected from using functools.lru_cache in Python?
Using functools.lru_cache
can lead to significant performance improvements, specifically:
- Reduced Computation Time: By storing results of previous calls, subsequent calls with the same arguments can return instantly without recomputing the result.
-
Memory Efficiency: Although
lru_cache
uses additional memory to store cached results, this can be offset by reduced CPU time, especially for recursive functions or those involving complex computations. - Database Load Reduction: For functions that perform database queries, caching results can dramatically reduce the load on the database and improve response times.
Example:
Consider a recursive Fibonacci function. Without caching, calculating fibonacci(100)
would require millions of redundant calculations. With lru_cache
, each unique call is calculated only once, and subsequent calls reuse these results.
from functools import lru_cache @lru_cache(maxsize=None) def fibonacci(n): if n < 2: return n return fibonacci(n-1) fibonacci(n-2) # First call: actual computation print(fibonacci(100)) # Quick due to caching # Subsequent calls: instant due to cached results print(fibonacci(100)) # Much faster
How does functools.lru_cache handle function arguments and what are its limitations?
Handling Function Arguments:
functools.lru_cache
uses the arguments passed to the function as keys in its cache dictionary. It hashes these arguments to create a unique key, which allows it to store and retrieve results efficiently. This means:
- Immutable Arguments: Only immutable types (like integers, strings, and tuples) can be used as keys. Mutable types (like lists and dictionaries) cannot be used reliably because their hash value may change.
- Keyword Arguments: Keyword arguments are handled by converting them to a tuple of their sorted keys and values, ensuring that the order of keyword arguments does not affect caching.
Limitations:
-
Cache Size: The decorator has a
maxsize
parameter, which limits the number of cached results. Settingmaxsize=None
can lead to unlimited cache growth, potentially causing memory issues. -
Function Overhead: There is a small overhead in checking the cache and managing the LRU queue, which can make
lru_cache
less efficient for very cheap operations. - Mutable Arguments: As mentioned, using mutable arguments can lead to unexpected behavior since their hash values may change after caching.
- No Automatic Invalidation: Cached results are not automatically invalidated. If underlying data changes, the cache must be manually cleared or updated.
In what types of applications or scenarios would functools.lru_cache be most advantageous?
functools.lru_cache
is particularly advantageous in the following scenarios:
- Recursive Algorithms: For functions like recursive Fibonacci calculations, dynamic programming problems, or tree traversals where subproblems are solved repeatedly.
- Web Applications: To cache results of expensive database queries or API calls to reduce server load and improve response times.
- Scientific Computing: For functions that involve complex calculations but are called repeatedly with the same arguments, such as solving differential equations or matrix operations.
- Machine Learning Pipelines: Caching results of preprocessing steps or model predictions when the input data does not change frequently.
- Game Development: For caching results of expensive computations related to game physics, AI decision-making, or procedural generation.
- Financial Applications: For caching results of financial models, risk calculations, or simulation outcomes that are frequently called with the same parameters.
In summary, functools.lru_cache
is most beneficial in scenarios where function calls are expensive, recurring, and the results do not change often. By caching these results, applications can achieve significant performance gains and efficiency improvements.
The above is the detailed content of Explain the use of functools.lru_cache. How does it work, and when is it beneficial?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


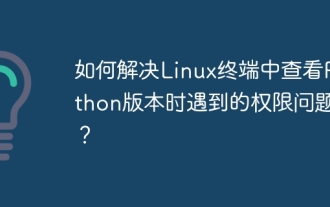
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
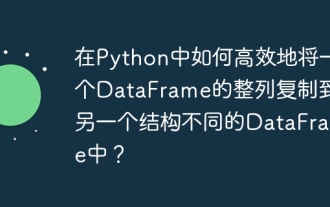
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
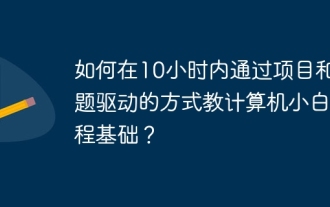
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
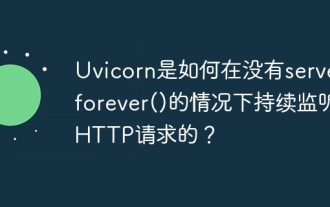
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
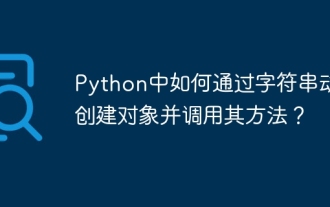
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
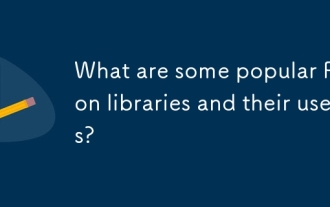
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
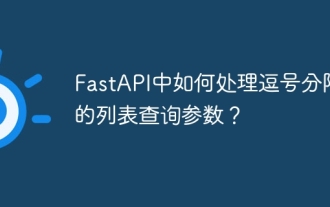
Fastapi ...
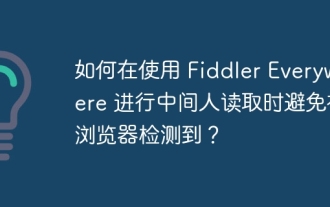
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
