


Explain the concept of "duck typing" in Python. What are its advantages and disadvantages?
Explain the concept of "duck typing" in Python. What are its advantages and disadvantages?
Duck typing is a concept in Python that allows objects to be treated as if they were of a certain type, based on their behavior rather than their actual type. The term comes from the saying, "If it walks like a duck and quacks like a duck, then it must be a duck." In Python, this means that you can call methods on an object without checking its type, as long as the object has those methods.
Advantages of Duck Typing:
- Flexibility: Duck typing allows for more flexible code. You can write functions that work with a variety of objects, as long as they have the required methods or attributes.
- Less Boilerplate Code: Since you don't need to check the type of an object before using it, you can write more concise code. This reduces the amount of boilerplate code needed for type checking.
- Easier to Refactor: Duck typing makes it easier to refactor code. You can change the type of an object without having to change the code that uses it, as long as the new object behaves similarly.
Disadvantages of Duck Typing:
- Runtime Errors: Because type checking is not done at compile time, errors may only be discovered at runtime. This can lead to unexpected behavior if an object does not have the expected methods or attributes.
- Difficulty in Debugging: Without explicit type checking, it can be harder to debug code. It may take longer to identify the source of an error if it's caused by an object not behaving as expected.
- Less Explicit Code: Some developers find duck typing less explicit and harder to understand, especially for those coming from statically typed languages. This can make the code less readable and maintainable for some teams.
How does duck typing affect code maintainability in Python projects?
Duck typing can have both positive and negative effects on code maintainability in Python projects.
Positive Effects:
- Easier Refactoring: As mentioned earlier, duck typing makes it easier to refactor code. You can change the type of an object without having to update all the places where it's used, as long as the new object behaves similarly. This can make maintenance easier and less time-consuming.
- More Flexible Code: Duck typing allows for more flexible code, which can be easier to maintain. You can add new types of objects to your code without having to change existing code, as long as the new objects behave as expected.
Negative Effects:
- Runtime Errors: The lack of compile-time type checking can lead to runtime errors, which can be harder to find and fix. This can make maintenance more difficult, especially in large projects.
- Less Readable Code: Some developers find duck typing less explicit and harder to understand. This can make the code less readable and maintainable, especially for new team members or those coming from statically typed languages.
- Difficulty in Debugging: Without explicit type checking, it can be harder to debug code. This can make maintenance more challenging, as it may take longer to identify and fix issues.
Can you provide examples of when duck typing in Python might lead to runtime errors?
Here are a few examples of when duck typing in Python might lead to runtime errors:
-
Missing Method:
class Duck: def quack(self): print("Quack!") class Dog: def bark(self): print("Woof!") def make_sound(animal): animal.quack() duck = Duck() dog = Dog() make_sound(duck) # This works fine make_sound(dog) # This will raise an AttributeError because Dog does not have a 'quack' method
Copy after loginIn this example, the
make_sound
function assumes that any object passed to it has aquack
method. When aDog
object is passed, it raises anAttributeError
becauseDog
does not have aquack
method. Incorrect Method Signature:
class Duck: def quack(self): print("Quack!") class Parrot: def quack(self, volume): print("Quack!" * volume) def make_sound(animal): animal.quack() duck = Duck() parrot = Parrot() make_sound(duck) # This works fine make_sound(parrot) # This will raise a TypeError because Parrot's 'quack' method requires an argument
Copy after loginIn this example, the
make_sound
function assumes that thequack
method takes no arguments. When aParrot
object is passed, it raises aTypeError
becauseParrot
'squack
method requires an argument.Unexpected Behavior:
class Duck: def quack(self): print("Quack!") class SilentDuck: def quack(self): pass def make_sound(animal): animal.quack() duck = Duck() silent_duck = SilentDuck() make_sound(duck) # This works fine make_sound(silent_duck) # This will not raise an error, but the behavior is unexpected
Copy after loginIn this example, the
make_sound
function assumes that thequack
method will produce some output. When aSilentDuck
object is passed, it does not raise an error, but the behavior is unexpected because nothing is printed.
What are the best practices for using duck typing effectively in Python development?
To use duck typing effectively in Python development, consider the following best practices:
Use Type Hints:
While duck typing allows for flexibility, using type hints can help catch potential errors early and make the code more readable. Type hints can be used to indicate the expected types of function parameters and return values.from typing import Any def make_sound(animal: Any) -> None: animal.quack()
Copy after loginImplement Defensive Programming:
Use defensive programming techniques to check for the existence of methods or attributes before using them. This can help prevent runtime errors.def make_sound(animal: Any) -> None: if hasattr(animal, 'quack') and callable(getattr(animal, 'quack')): animal.quack() else: print("This animal does not quack.")
Copy after login- Write Comprehensive Tests:
Write thorough unit tests to ensure that your code works correctly with different types of objects. This can help catch any unexpected behavior early in the development process. Document Assumptions:
Clearly document any assumptions about the behavior of objects in your code. This can help other developers understand how to use your code and what to expect.def make_sound(animal: Any) -> None: """ Make the animal quack. This function assumes that the animal has a 'quack' method. Args: animal (Any): An object that has a 'quack' method. """ animal.quack()
Copy after loginUse Abstract Base Classes (ABCs):
When appropriate, use abstract base classes to define a common interface for objects. This can help ensure that objects have the required methods and make your code more maintainable.from abc import ABC, abstractmethod class Quackable(ABC): @abstractmethod def quack(self): pass class Duck(Quackable): def quack(self): print("Quack!") def make_sound(animal: Quackable) -> None: animal.quack()
Copy after login
By following these best practices, you can leverage the flexibility of duck typing while minimizing its potential drawbacks.
The above is the detailed content of Explain the concept of "duck typing" in Python. What are its advantages and disadvantages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










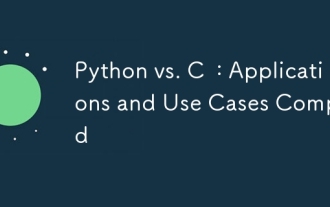
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
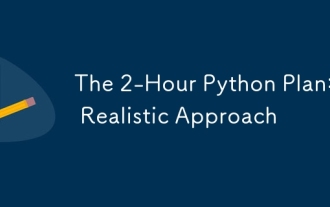
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
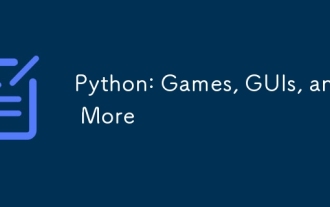
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
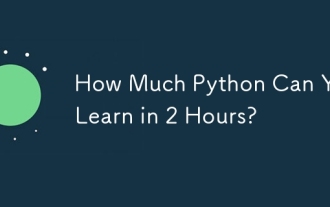
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
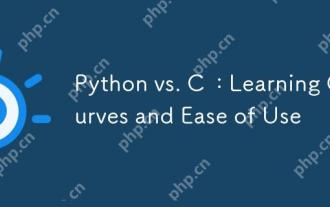
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
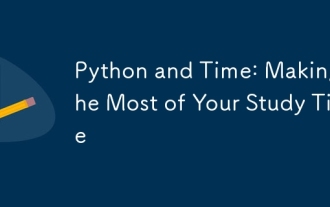
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
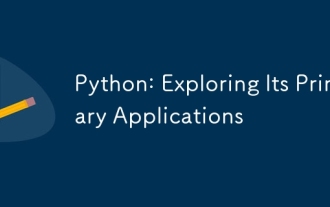
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
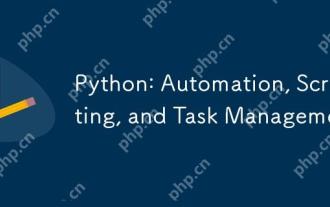
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
