


Explain the difference between value receivers and pointer receivers on Go methods. When would you use each?
Explain the difference between value receivers and pointer receivers on Go methods. When would you use each?
In Go, methods can be associated with a type, and the method receiver can be defined as either a value or a pointer. The difference between value receivers and pointer receivers lies in how they interact with the underlying data and how changes to the data are managed.
Value Receivers:
A value receiver is specified by using the type itself (e.g., func (t T) Method()
). When a method with a value receiver is called, the method operates on a copy of the value. Any changes made within the method do not affect the original value outside the method. Value receivers are suitable when the method does not need to modify the state of the receiver, or when the receiver is a small, cheap-to-copy type.
Pointer Receivers:
A pointer receiver is specified by using a pointer to the type (e.g., func (t *T) Method()
). When a method with a pointer receiver is called, it operates directly on the value that the pointer points to. Any changes made within the method affect the original value. Pointer receivers are necessary when the method needs to modify the state of the receiver, or when the receiver is a large, expensive-to-copy type.
When to Use Each:
-
Value Receivers: Use when:
- The method does not need to modify the receiver's state.
- The receiver type is small and copying it is not expensive (e.g., built-in types like
int
,float64
, etc.). - You want to ensure that the method does not modify the original value.
-
Pointer Receivers: Use when:
- The method needs to modify the receiver's state.
- The receiver type is large and copying it would be expensive (e.g., structs with many fields).
- You need to ensure that changes made within the method affect the original value.
What are the performance implications of using value receivers versus pointer receivers in Go?
The performance implications of using value receivers versus pointer receivers in Go depend on the size of the receiver and the nature of the method calls.
Value Receivers:
- Copying Overhead: For small types, the overhead of copying the value is negligible. However, for larger types, copying can be expensive in terms of memory and CPU time.
- Memory Allocation: Each method call with a value receiver may involve creating a new copy of the value, which can lead to increased memory allocation and garbage collection overhead.
- Thread Safety: Since value receivers operate on a copy, they are inherently thread-safe as changes made within the method do not affect the original value.
Pointer Receivers:
- No Copying Overhead: Pointer receivers do not incur the cost of copying the value, as they operate directly on the original value.
- Less Memory Allocation: Since no new copies are made, there is less memory allocation and garbage collection overhead.
- Thread Safety: Pointer receivers are not inherently thread-safe, as changes made within the method directly affect the original value. Care must be taken to ensure thread safety when using pointer receivers.
In summary, value receivers may lead to higher memory usage and copying overhead but can be faster for small types, whereas pointer receivers are more efficient for large types and methods that need to modify the original value.
How does the choice between value and pointer receivers affect the behavior of method calls in Go?
The choice between value and pointer receivers in Go significantly affects the behavior of method calls in several ways:
Modifiability:
- Value Receivers: Changes made to the receiver within the method do not affect the original value outside the method. The method operates on a copy of the original value.
- Pointer Receivers: Changes made to the receiver within the method directly affect the original value. The method operates on the value that the pointer points to.
Receiver Type Compatibility:
- Value Receivers: A method defined with a value receiver can be called on both values and pointers of the receiver type. Go automatically dereferences the pointer to match the method signature.
- Pointer Receivers: A method defined with a pointer receiver can be called on both pointers and values of the receiver type. Go automatically takes the address of the value to match the method signature.
Method Dispatch:
- Value Receivers: The method call involves creating a copy of the value, which may be slightly slower for large types but can be faster for small types due to the efficiency of value copying.
- Pointer Receivers: The method call involves passing the address of the value, which is generally faster and more memory-efficient, especially for large types.
Semantics and Intent:
- Value Receivers: Indicate that the method does not modify the original value, which can improve code readability and maintainability.
- Pointer Receivers: Indicate that the method may modify the original value, which is important for conveying the method's intent and potential side effects.
In what scenarios would using a pointer receiver be more beneficial than a value receiver in Go programming?
Using a pointer receiver in Go programming would be more beneficial than a value receiver in the following scenarios:
Modifying the Receiver's State:
When a method needs to modify the state of the receiver, a pointer receiver is necessary. This is common in scenarios where you need to update properties of an object, such as setting a new value for a field in a struct.
type User struct { Name string Age int } func (u *User) SetAge(age int) { u.Age = age }
Large Receiver Types:
For large structs or types that are expensive to copy, using a pointer receiver is more efficient. Copying large structures can lead to increased memory usage and performance overhead, which can be avoided by using pointer receivers.
type LargeStruct struct { Data [1000]int64 } func (ls *LargeStruct) ProcessData() { // Process the data directly without copying the large struct }
Memory Efficiency:
When memory efficiency is a concern, pointer receivers can help reduce memory allocation and garbage collection overhead. This is particularly important in high-performance applications or when dealing with a large number of objects.
Thread Safety with Shared State:
In scenarios where multiple goroutines need to access and potentially modify a shared state, using pointer receivers with appropriate synchronization mechanisms (e.g., mutexes) can help manage the shared state effectively.
type Counter struct { mu sync.Mutex count int } func (c *Counter) Increment() { c.mu.Lock() defer c.mu.Unlock() c.count }
Interface Satisfaction:
When implementing interfaces, especially when the interface methods require modifying the receiver, pointer receivers ensure that the implementation can satisfy the interface contract.
type Logger interface { Log(message string) } type FileLogger struct { file *os.File } func (l *FileLogger) Log(message string) { // Write to the file directly l.file.WriteString(message "\n") }
In summary, pointer receivers are more beneficial in Go programming when the method needs to modify the receiver's state, the receiver type is large, memory efficiency is crucial, shared state needs to be managed, or when implementing interfaces that require state modification.
The above is the detailed content of Explain the difference between value receivers and pointer receivers on Go methods. When would you use each?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


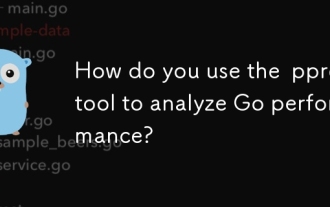
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
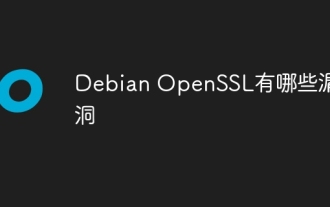
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
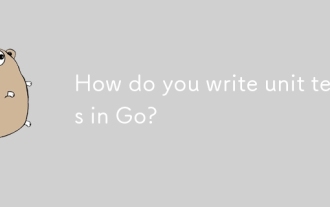
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
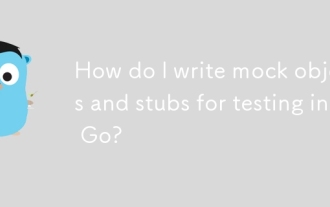
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
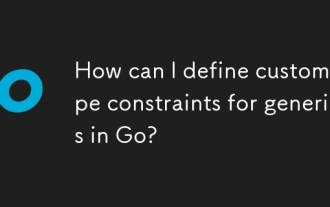
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
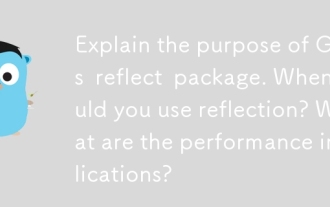
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
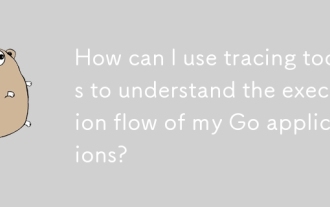
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
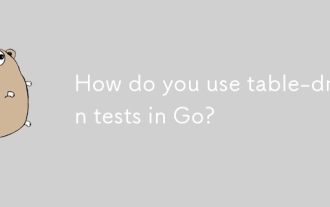
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
