How are strings represented in Go? Are they mutable or immutable?
How are strings represented in Go? Are they mutable or immutable?
In Go, strings are represented as a sequence of bytes ([]byte
), but they are not just simple byte slices. They are actually a structure that includes a pointer to the underlying bytes and a length, making them more akin to a read-only view into a byte slice. The string type in Go is defined as:
type stringStruct struct { str unsafe.Pointer len int }
Strings in Go are immutable, which means once a string is created, it cannot be modified. Any operation that seems to modify a string actually creates a new string. This immutability has several implications for how strings are used and managed in Go programs.
What operations can be performed on strings in Go?
Go provides a variety of operations that can be performed on strings, leveraging its standard library and built-in methods:
-
Concatenation: Strings can be concatenated using the
strings.Join
function for more efficient concatenation of multiple strings. -
Comparison: Strings can be compared using the standard comparison operators (
,
!=
,, <code>, <code>>
,>=
). These operations are case-sensitive. -
Indexing and Slicing: Individual characters in a string can be accessed using indexing (
str[index]
). Slicing is also supported (str[start:end]
), which creates a new string. -
Length: The
len()
function returns the length of the string in bytes. -
Conversion: Strings can be converted to and from other types. For example,
strconv
package functions can convert strings to integers and vice versa. -
Searching and Manipulating: The
strings
package provides functions likeContains
,HasPrefix
,HasSuffix
,Index
,Replace
,Split
,Trim
, and others to search, manipulate, and analyze strings. -
Rune Iteration: Strings can be iterated over as runes (Unicode code points) using
for range
loop, which is useful for processing text containing Unicode characters.
How does Go handle string concatenation and what are the performance implications?
Go handles string concatenation primarily through the
operator and the strings.Join
function. The method of concatenation can have significant performance implications:
-
Using the
-
Using
strings.Join
: Thestrings.Join
function is more efficient when concatenating a slice of strings, as it allocates memory only once for the final result. This approach is particularly useful when dealing with a known number of strings. -
Using
bytes.Buffer
orstrings.Builder
: For dynamic string building, especially in loops, usingbytes.Buffer
orstrings.Builder
is recommended. These types allow appending to a buffer without the overhead of creating new strings each time, offering better performance.
The performance implications of string concatenation are mainly related to memory allocation and copying. Using inefficient methods can lead to higher memory usage and slower performance, especially with large numbers of concatenations or in performance-critical sections of code.
What are the best practices for working with strings in Go to optimize memory usage?
To optimize memory usage when working with strings in Go, consider the following best practices:
-
Minimize String Creation: Since strings are immutable, try to minimize the creation of new strings. If possible, work with byte slices (
[]byte
) or usestrings.Builder
for building strings dynamically. -
Use
strings.Builder
for Dynamic String Building: When you need to build a string incrementally, especially in loops, usestrings.Builder
instead of concatenating with -
Prefer
strings.Join
for Multiple String Concatenation: When you need to concatenate multiple strings, usestrings.Join
instead of multiple -
Use Byte Slices for Mutable Data: If you need to modify data, consider using a
[]byte
instead of a string. You can convert a string to a byte slice using[]byte(str)
and convert back withstring(byteSlice)
when necessary. - Avoid Unnecessary Conversions: Be mindful of unnecessary conversions between strings and other types, as each conversion may involve memory allocation.
-
Use Efficient String Functions: When manipulating strings, use efficient functions from the
strings
package. For instance, usestrings.Trim
instead of manually checking and removing characters. - Consider Interning: For frequently used strings, consider interning them using a map to store and reuse string pointers, which can reduce memory usage by avoiding duplicate copies of the same string.
By following these practices, you can optimize memory usage and improve the performance of your Go programs when working with strings.
The above is the detailed content of How are strings represented in Go? Are they mutable or immutable?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


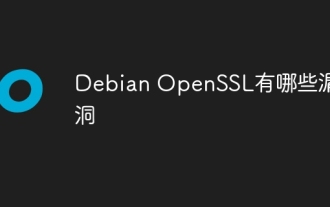
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
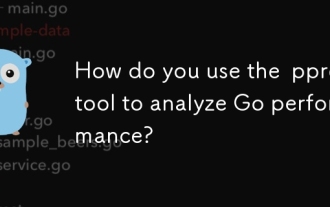
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
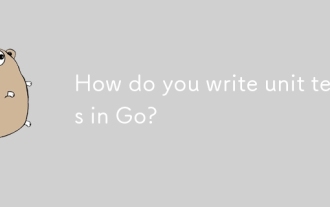
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
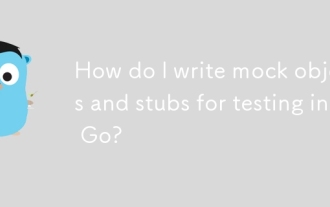
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
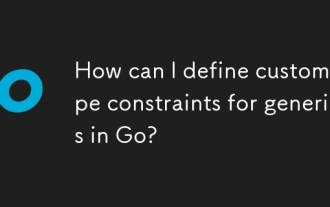
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
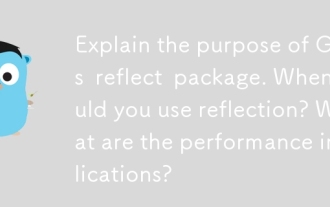
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
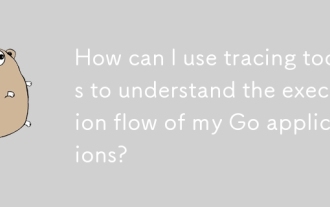
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
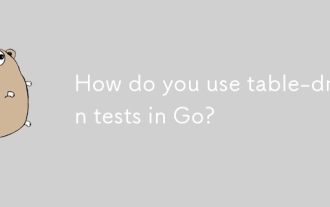
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
