


Explain how Go's channels work. What are buffered channels and unbuffered channels?
Mar 26, 2025 pm 01:33 PMExplain how Go's channels work. What are buffered channels and unbuffered channels?
Go's channels are a powerful feature for managing concurrency and communication between goroutines. A channel is a typed conduit through which you can send and receive values with the channel operator . Channels can be thought of as pipes that allow goroutines to communicate safely and efficiently.
Unbuffered Channels:
Unbuffered channels have no capacity to hold values. When you send a value to an unbuffered channel, the sending goroutine will block until another goroutine receives the value. Similarly, a goroutine trying to receive from an unbuffered channel will block until a value is sent. This synchronization ensures that the send and receive operations happen simultaneously, making unbuffered channels useful for ensuring that operations occur in a specific order.
Here's an example of an unbuffered channel:
ch := make(chan int) go func() { ch <- 42 // This will block until the value is received }() value := <-ch // This will unblock the sender fmt.Println(value) // Prints 42
Buffered Channels:
Buffered channels, on the other hand, have a capacity to hold a certain number of values. You specify this capacity when you create the channel. Sending a value to a buffered channel will not block if the channel is not full. Similarly, receiving from a buffered channel will not block if the channel is not empty. However, if the channel is full, the sender will block until space becomes available, and if the channel is empty, the receiver will block until a value is sent.
Here's an example of a buffered channel:
ch := make(chan int, 1) // Buffered channel with capacity 1 ch <- 42 // This will not block because the channel has space value := <-ch // This will not block because the channel has a value fmt.Println(value) // Prints 42
What are the main differences in usage between buffered and unbuffered channels in Go?
The main differences in usage between buffered and unbuffered channels in Go are centered around their behavior in terms of blocking and synchronization:
Blocking Behavior:
- Unbuffered Channels: Sending and receiving operations on unbuffered channels are synchronous. The sender blocks until the receiver is ready, and vice versa. This ensures that the send and receive operations happen at the same time.
- Buffered Channels: Sending to a buffered channel does not block if the channel is not full, and receiving does not block if the channel is not empty. This allows for more flexibility in the timing of operations.
Synchronization:
- Unbuffered Channels: They are often used for synchronization between goroutines, ensuring that certain operations happen in a specific order. For example, you might use an unbuffered channel to signal that a task is complete.
- Buffered Channels: They are useful when you want to decouple the sender and receiver, allowing the sender to continue working without waiting for the receiver. This can be beneficial for improving throughput in certain scenarios.
Use Cases:
- Unbuffered Channels: Ideal for scenarios where you need strict synchronization, such as passing work between stages in a pipeline or signaling the completion of a task.
- Buffered Channels: Suitable for scenarios where you want to handle bursts of data or when you need to smooth out the flow of data between goroutines, such as in a producer-consumer pattern.
How can I implement concurrency using Go's channels effectively?
To implement concurrency effectively using Go's channels, consider the following strategies:
Use Channels for Communication:
Channels are the primary means of communication between goroutines. Use them to pass data and signals between different parts of your program. For example, you can use channels to send tasks to worker goroutines and receive results back.tasks := make(chan int) results := make(chan int) go worker(tasks, results) tasks <- 1 tasks <- 2 close(tasks) result1, result2 := <-results, <-results fmt.Println(result1, result2)
Copy after loginImplement the Worker Pool Pattern:
A worker pool can help manage a fixed number of goroutines to handle a potentially large number of tasks. This can prevent overwhelming the system with too many goroutines.func workerPool(numWorkers int, tasks <-chan int, results chan<- int) { for i := 0; i < numWorkers; i { go func() { for task := range tasks { results <- processTask(task) } }() } }
Copy after loginUse Select for Multiple Channels:
Theselect
statement allows you to wait on multiple channel operations simultaneously. This is useful for handling multiple sources of data or implementing timeouts.select { case result := <-ch1: fmt.Println("Received from ch1:", result) case result := <-ch2: fmt.Println("Received from ch2:", result) case <-time.After(1 * time.Second): fmt.Println("Timeout") }
Copy after loginClose Channels to Signal Completion:
Closing a channel signals to receivers that no more values will be sent. Use therange
keyword to iterate over a channel until it is closed.ch := make(chan int) go func() { for i := 0; i < 5; i { ch <- i } close(ch) }() for v := range ch { fmt.Println(v) }
Copy after login- Avoid Deadlocks:
Be mindful of potential deadlocks, especially with unbuffered channels. Ensure that there is always a receiver ready for a sender, and vice versa.
What common pitfalls should I avoid when working with channels in Go?
When working with channels in Go, there are several common pitfalls to avoid:
Deadlocks:
Deadlocks occur when goroutines are blocked indefinitely, waiting for each other. This can happen if you try to send to a channel without a corresponding receiver, or vice versa. Always ensure that there is a receiver for every sender.ch := make(chan int) ch <- 42 // This will deadlock because there is no receiver
Copy after loginLeaking Goroutines:
Goroutines that are waiting on a channel that will never be closed or receive a value can lead to goroutine leaks. Always ensure that channels are closed when no more values will be sent.ch := make(chan int) go func() { for v := range ch { fmt.Println(v) } }() // Remember to close the channel when done close(ch)
Copy after loginIgnoring Channel Errors:
When using channels, it's important to handle potential errors, such as sending to a closed channel or receiving from a closed channel. Use theok
idiom to check for these conditions.ch := make(chan int) close(ch) v, ok := <-ch if !ok { fmt.Println("Channel is closed") }
Copy after login- Overusing Channels:
While channels are powerful, overusing them can lead to complex and hard-to-debug code. Consider using other synchronization primitives like mutexes or wait groups when appropriate. Not Using Buffered Channels When Needed:
Unbuffered channels can lead to unnecessary blocking in some scenarios. Use buffered channels to improve performance when you need to decouple the sender and receiver.ch := make(chan int, 10) // Buffered channel with capacity 10
Copy after login-
Ignoring Channel Capacity:
When using buffered channels, be aware of their capacity. Sending to a full channel will block, and receiving from an empty channel will block. Monitor the channel's state to avoid unexpected blocking.
By being aware of these pitfalls and following best practices, you can use Go's channels effectively to manage concurrency and communication in your programs.
The above is the detailed content of Explain how Go's channels work. What are buffered channels and unbuffered channels?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
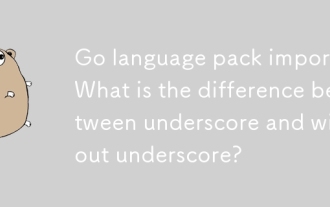
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
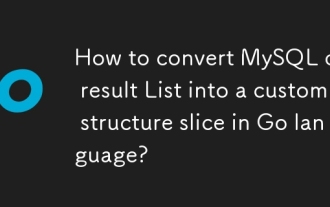
How to convert MySQL query result List into a custom structure slice in Go language?
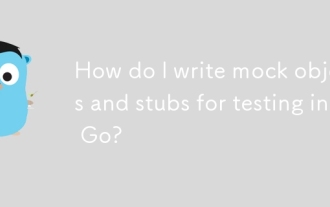
How do I write mock objects and stubs for testing in Go?
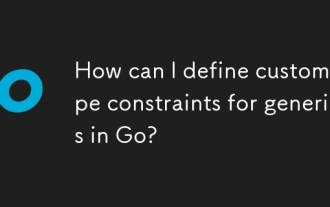
How can I define custom type constraints for generics in Go?
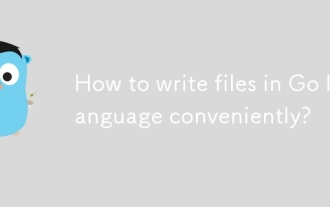
How to write files in Go language conveniently?
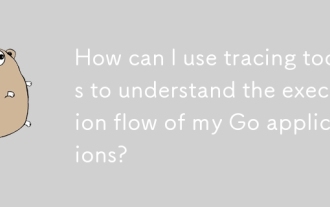
How can I use tracing tools to understand the execution flow of my Go applications?
