


Explain how to use concurrent.futures to manage thread pools and process pools.
Explain how to use concurrent.futures to manage thread pools and process pools.
The concurrent.futures
module in Python provides a high-level interface for asynchronously executing callables using threads or separate processes. It includes two main classes for managing pools: ThreadPoolExecutor
for managing a pool of threads, and ProcessPoolExecutor
for managing a pool of processes. Here's how to use them:
-
Import the module:
import concurrent.futures
Copy after login Create a ThreadPoolExecutor or ProcessPoolExecutor:
For threads:
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor: # Use the executor
Copy after loginFor processes:
with concurrent.futures.ProcessPoolExecutor(max_workers=5) as executor: # Use the executor
Copy after loginThe
max_workers
parameter specifies the maximum number of threads or processes to use.
Submit tasks to the executor:
You can submit tasks using thesubmit
method, which returns aFuture
object representing the execution of the task.future = executor.submit(task_function, arg1, arg2)
Copy after loginRetrieve results:
You can retrieve the result of a task using theresult
method of theFuture
object.result = future.result()
Copy after loginUsing
map
for multiple tasks:
Themap
method can be used to apply a function to an iterable of arguments.results = list(executor.map(task_function, iterable_of_args))
Copy after loginUsing
as_completed
for handling results as they finish:
Theas_completed
function can be used to process results as they become available.for future in concurrent.futures.as_completed(futures): result = future.result() # Process result
Copy after login
What are the key differences between using ThreadPoolExecutor and ProcessPoolExecutor in concurrent.futures?
The key differences between ThreadPoolExecutor
and ProcessPoolExecutor
in concurrent.futures
are:
Execution Context:
ThreadPoolExecutor
uses threads within the same process. All threads share the same memory space, which allows for easy sharing of data but can lead to issues like race conditions and deadlocks.ProcessPoolExecutor
uses separate processes. Each process has its own memory space, which prevents issues like race conditions but makes sharing data more complex.
Performance:
ThreadPoolExecutor
is generally faster to start and stop because creating threads is less resource-intensive than creating processes.ProcessPoolExecutor
can utilize multiple CPU cores more effectively, making it better suited for CPU-bound tasks. However, it has higher overhead due to inter-process communication.
Use Cases:
ThreadPoolExecutor
is ideal for I/O-bound tasks, such as network requests or file operations, where threads can be blocked without consuming CPU resources.ProcessPoolExecutor
is better for CPU-bound tasks, such as data processing or scientific computing, where parallel execution on multiple cores can significantly improve performance.
Global Interpreter Lock (GIL):
- In CPython, the GIL prevents multiple native threads from executing Python bytecodes at once. This means that
ThreadPoolExecutor
may not fully utilize multiple cores for CPU-bound tasks. ProcessPoolExecutor
bypasses the GIL because each process has its own Python interpreter.
- In CPython, the GIL prevents multiple native threads from executing Python bytecodes at once. This means that
How can I monitor and control the execution of tasks in a thread pool or process pool using concurrent.futures?
Monitoring and controlling the execution of tasks in a thread pool or process pool using concurrent.futures
can be achieved through several methods:
Using
Future
objects:You can check the status of a task using the
done()
,running()
, andcancelled()
methods of theFuture
object.future = executor.submit(task_function) if future.done(): result = future.result() elif future.running(): print("Task is running") elif future.cancelled(): print("Task was cancelled")
Copy after login
Cancelling tasks:
You can attempt to cancel a task using the
cancel()
method of theFuture
object.future = executor.submit(task_function) if future.cancel(): print("Task was successfully cancelled") else: print("Task could not be cancelled")
Copy after login
Using
as_completed
:The
as_completed
function allows you to process results as they become available, which can help in monitoring the progress of tasks.futures = [executor.submit(task_function, arg) for arg in args] for future in concurrent.futures.as_completed(futures): result = future.result() # Process result
Copy after login
Using
wait
:The
wait
function can be used to wait for a set of futures to complete, with options to wait for all to complete or just a subset.futures = [executor.submit(task_function, arg) for arg in args] done, not_done = concurrent.futures.wait(futures, timeout=None, return_when=concurrent.futures.ALL_COMPLETED)
Copy after login
Using
ThreadPoolExecutor
orProcessPoolExecutor
attributes:- You can access the number of active threads or processes using the
ThreadPoolExecutor._threads
orProcessPoolExecutor._processes
attributes, though these are not part of the public API and should be used cautiously.
- You can access the number of active threads or processes using the
Can you provide an example of how to handle exceptions in tasks managed by concurrent.futures?
Handling exceptions in tasks managed by concurrent.futures
can be done by catching exceptions when retrieving the result of a Future
object. Here's an example:
import concurrent.futures def task_function(x): if x == 0: raise ValueError("x cannot be zero") return 1 / x def main(): with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor: futures = [executor.submit(task_function, i) for i in range(5)] for future in concurrent.futures.as_completed(futures): try: result = future.result() print(f"Result: {result}") except ValueError as e: print(f"ValueError occurred: {e}") except ZeroDivisionError as e: print(f"ZeroDivisionError occurred: {e}") except Exception as e: print(f"An unexpected error occurred: {e}") if __name__ == "__main__": main()
In this example, we submit tasks to a ThreadPoolExecutor
and use as_completed
to process the results as they become available. We catch specific exceptions (ValueError
and ZeroDivisionError
) and a general Exception
to handle any unexpected errors. This approach allows you to handle exceptions gracefully and continue processing other tasks.
The above is the detailed content of Explain how to use concurrent.futures to manage thread pools and process pools.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
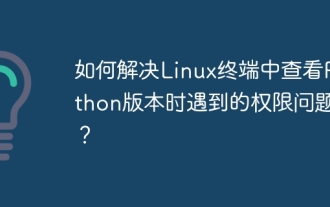
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
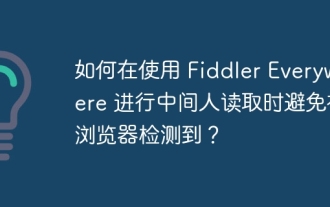
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
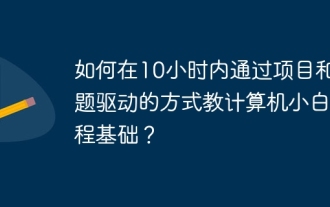
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
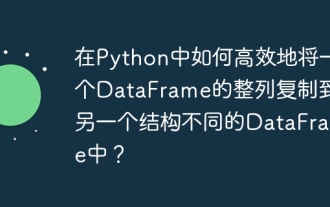
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
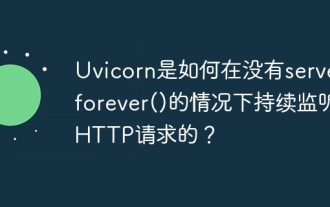
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
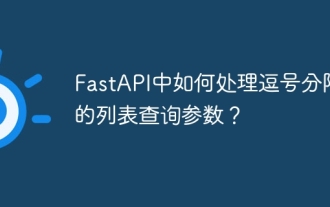
Fastapi ...
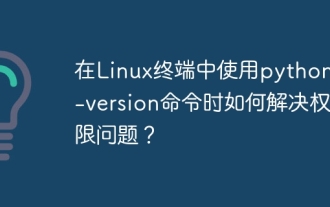
Using python in Linux terminal...
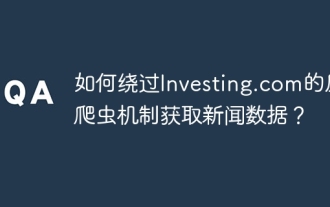
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
