


What are the benefits of using asynchronous queues (e.g., asyncio.Queue)?
What are the benefits of using asynchronous queues (e.g., asyncio.Queue)?
Asynchronous queues, such as those provided by asyncio.Queue
in Python, offer several significant benefits that can enhance the efficiency and performance of concurrent programming. Here are some key advantages:
- Improved Concurrency: Asynchronous queues allow multiple tasks to run concurrently without blocking each other. This is particularly useful in I/O-bound applications where tasks frequently wait for I/O operations to complete. By using an asynchronous queue, a task can yield control back to the event loop while waiting, allowing other tasks to proceed.
- Resource Efficiency: Asynchronous queues help in managing resources more efficiently. Since tasks do not block the execution of other tasks, fewer threads or processes are needed to handle concurrent operations, leading to lower memory usage and better overall system performance.
- Simplified Task Management: Asynchronous queues provide a straightforward way to manage task dependencies and communication between different parts of a program. Producers can add items to the queue, and consumers can retrieve them when ready, facilitating a clean separation of concerns.
- Scalability: By allowing more tasks to be processed within the same time frame, asynchronous queues contribute to the scalability of applications. This is especially beneficial in scenarios where the number of concurrent operations can grow significantly.
- Error Handling and Robustness: Asynchronous queues can be used to implement robust error handling mechanisms. For instance, if a consumer task fails, the queue can be designed to handle retries or redirect the task to another consumer, enhancing the overall reliability of the system.
What types of applications can benefit most from implementing asynchronous queues?
Several types of applications can significantly benefit from implementing asynchronous queues, particularly those that involve high levels of concurrency and I/O operations. Here are some examples:
- Web Servers and APIs: Web servers and APIs often handle multiple client requests simultaneously. Asynchronous queues can help manage these requests efficiently, ensuring that the server remains responsive even under heavy load.
- Real-time Data Processing: Applications that process real-time data, such as financial trading platforms or IoT data streams, can use asynchronous queues to handle incoming data without blocking other operations. This ensures that data is processed in a timely manner.
- Chat and Messaging Applications: In chat and messaging applications, asynchronous queues can be used to manage message delivery and processing. This allows the application to handle a large number of concurrent users and messages without performance degradation.
- Task Queues and Job Scheduling: Applications that involve task queues and job scheduling, such as background job processing systems, can benefit from asynchronous queues. They can manage and distribute tasks across multiple workers efficiently.
- Distributed Systems: In distributed systems, asynchronous queues can facilitate communication and coordination between different nodes or services. This is crucial for maintaining the overall performance and reliability of the system.
How does using asynchronous queues improve the performance and scalability of concurrent systems?
Using asynchronous queues can significantly improve the performance and scalability of concurrent systems in several ways:
- Non-blocking Operations: Asynchronous queues allow tasks to operate without blocking each other. When a task needs to wait for an I/O operation or another task to complete, it can yield control back to the event loop, allowing other tasks to proceed. This non-blocking nature ensures that the system remains responsive and efficient.
- Efficient Resource Utilization: By reducing the need for multiple threads or processes, asynchronous queues help in utilizing system resources more efficiently. This leads to lower memory usage and better CPU utilization, which is crucial for scaling applications to handle more concurrent operations.
- Load Balancing: Asynchronous queues can be used to implement load balancing mechanisms. For instance, tasks can be distributed across multiple consumers based on their current load, ensuring that no single consumer becomes a bottleneck.
- Scalability: The ability to handle more tasks within the same time frame directly contributes to the scalability of the system. As the number of concurrent operations grows, asynchronous queues help in maintaining performance levels without a proportional increase in resources.
- Improved Throughput: By allowing tasks to be processed concurrently, asynchronous queues can significantly increase the throughput of the system. This is particularly beneficial in scenarios where the system needs to handle a high volume of requests or data.
What are some best practices for managing and optimizing asynchronous queues in Python?
To effectively manage and optimize asynchronous queues in Python, consider the following best practices:
-
Proper Queue Sizing: Set an appropriate size for your queue to prevent it from growing indefinitely. This can be done using the
maxsize
parameter when initializing the queue. A well-sized queue helps in managing memory usage and preventing performance issues.queue = asyncio.Queue(maxsize=1000)
Copy after login - Task Prioritization: Implement task prioritization if your application requires it. You can use multiple queues with different priorities or implement a custom queue that supports priority-based operations.
Error Handling: Implement robust error handling mechanisms. Use try-except blocks to handle exceptions that may occur during task processing, and consider implementing retry logic for failed tasks.
async def process_task(task): try: # Process the task await some_operation(task) except Exception as e: # Handle the error, possibly retry print(f"Error processing task: {e}") await asyncio.sleep(1) # Wait before retrying await process_task(task) # Retry the task
Copy after loginMonitoring and Logging: Monitor the queue's performance and log important events. This can help in identifying bottlenecks and optimizing the system. Use logging libraries to track queue size, task processing times, and any errors.
import logging logging.basicConfig(level=logging.INFO) logger = logging.getLogger(__name__) async def monitor_queue(queue): while True: logger.info(f"Current queue size: {queue.qsize()}") await asyncio.sleep(60) # Log every minute
Copy after loginEfficient Task Distribution: Ensure that tasks are distributed efficiently among consumers. You can use multiple consumers to process tasks from the same queue, and consider implementing load balancing to distribute tasks evenly.
async def consumer(queue): while True: task = await queue.get() try: await process_task(task) finally: queue.task_done() async def main(): queue = asyncio.Queue() consumers = [asyncio.create_task(consumer(queue)) for _ in range(5)] # 5 consumers # Add tasks to the queue await queue.join() for c in consumers: c.cancel()
Copy after login- Avoiding Deadlocks: Be cautious of potential deadlocks, especially when using multiple queues. Ensure that tasks do not wait indefinitely for resources that are held by other tasks.
By following these best practices, you can effectively manage and optimize asynchronous queues in Python, leading to more efficient and scalable concurrent systems.
The above is the detailed content of What are the benefits of using asynchronous queues (e.g., asyncio.Queue)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
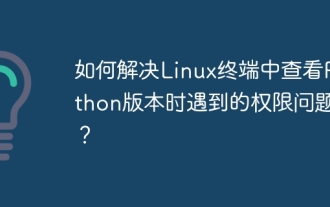
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
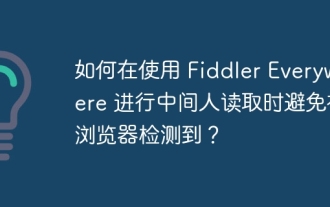
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
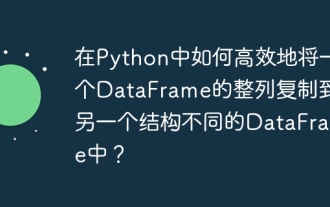
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
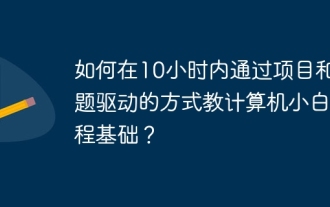
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
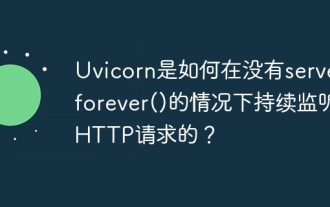
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
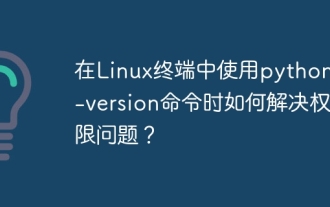
Using python in Linux terminal...
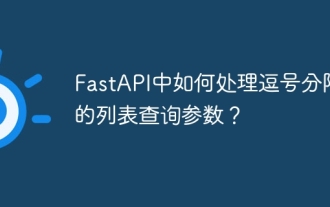
Fastapi ...
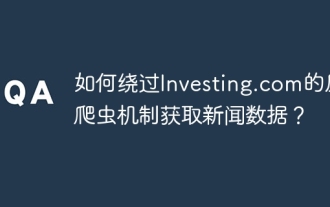
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
