


How can you prevent SQL injection vulnerabilities in Python?
How can you prevent SQL injection vulnerabilities in Python?
SQL injection vulnerabilities can pose a significant security risk to applications that interact with databases. In Python, you can prevent these vulnerabilities through several key strategies:
-
Use of Parameterized Queries: This is the most effective way to prevent SQL injection. Parameterized queries ensure that user inputs are treated as data, not executable code. For example, using the
execute
method with placeholders in the SQL statement ensures that input is escaped properly.import sqlite3 conn = sqlite3.connect('example.db') cursor = conn.cursor() user_input = "Robert'); DROP TABLE Students;--" cursor.execute("SELECT * FROM Users WHERE name = ?", (user_input,)) results = cursor.fetchall() conn.close()
Copy after login - Stored Procedures: Utilizing stored procedures on the database side can also help in preventing SQL injection. Stored procedures encapsulate the SQL logic and allow for the use of parameters, similar to parameterized queries.
- ORMs (Object-Relational Mappers): Using an ORM like SQLAlchemy or Django ORM can help abstract the SQL code and automatically protect against injection attacks by using parameterized queries internally.
- Input Validation and Sanitization: Validate and sanitize all user inputs before using them in database queries. While this alone is not sufficient, it adds an additional layer of security.
- Principle of Least Privilege: Ensure that the database user has only the permissions required to perform necessary operations. This reduces the damage that an injection attack could cause.
- Regular Updates and Patching: Keep your Python version, database, and any libraries up-to-date to protect against known vulnerabilities.
What are the best practices for using parameterized queries in Python to prevent SQL injection?
Using parameterized queries is a fundamental practice for preventing SQL injection attacks. Here are some best practices:
Always Use Parameters: Never concatenate user input directly into SQL statements. Use placeholders (
?
,%s
, etc.) instead of string formatting to insert data.import mysql.connector conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() user_input = "Robert'); DROP TABLE Students;--" query = "SELECT * FROM Users WHERE name = %s" cursor.execute(query, (user_input,)) results = cursor.fetchall() conn.close()
Copy after loginCopy after login- Use the Correct Placeholder: Different database libraries use different placeholders. For example,
sqlite3
uses?
, whilemysql.connector
uses%s
. Make sure to use the correct placeholder for your database library. - Avoid Complex Queries: While parameterized queries can handle complex queries, it's better to keep queries as simple as possible to reduce the risk of errors and make them easier to maintain.
Use ORM Libraries: If you're using an ORM like SQLAlchemy, it automatically uses parameterized queries, which simplifies the process and reduces the risk of SQL injection.
from sqlalchemy import create_engine, select from sqlalchemy.orm import sessionmaker from your_models import User engine = create_engine('sqlite:///example.db') Session = sessionmaker(bind=engine) session = Session() user_input = "Robert'); DROP TABLE Students;--" stmt = select(User).where(User.name == user_input) results = session.execute(stmt).scalars().all() session.close()
Copy after loginCopy after login- Error Handling: Implement proper error handling to manage and log any issues that arise from query execution, which can help in identifying potential security issues.
Can you recommend any Python libraries that help in securing database interactions against SQL injection?
Several Python libraries are designed to help secure database interactions and prevent SQL injection:
SQLAlchemy: SQLAlchemy is a popular ORM that provides a high-level interface for database operations. It automatically uses parameterized queries, which helps prevent SQL injection.
from sqlalchemy import create_engine, select from sqlalchemy.orm import sessionmaker from your_models import User engine = create_engine('sqlite:///example.db') Session = sessionmaker(bind=engine) session = Session() user_input = "Robert'); DROP TABLE Students;--" stmt = select(User).where(User.name == user_input) results = session.execute(stmt).scalars().all() session.close()
Copy after loginCopy after loginPsycopg2: This is a PostgreSQL adapter for Python that supports parameterized queries. It's widely used and well-maintained.
import psycopg2 conn = psycopg2.connect( dbname="yourdbname", user="yourusername", password="yourpassword", host="yourhost" ) cur = conn.cursor() user_input = "Robert'); DROP TABLE Students;--" cur.execute("SELECT * FROM users WHERE name = %s", (user_input,)) results = cur.fetchall() conn.close()
Copy after loginmysql-connector-python: This is the official Oracle-supported driver to connect MySQL with Python. It supports parameterized queries and is designed to prevent SQL injection.
import mysql.connector conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() user_input = "Robert'); DROP TABLE Students;--" query = "SELECT * FROM Users WHERE name = %s" cursor.execute(query, (user_input,)) results = cursor.fetchall() conn.close()
Copy after loginCopy after loginDjango ORM: If you're using the Django framework, its ORM automatically uses parameterized queries, providing a high level of protection against SQL injection.
from django.db.models import Q from your_app.models import User user_input = "Robert'); DROP TABLE Students;--" users = User.objects.filter(name=user_input)
Copy after login
How do you validate and sanitize user inputs in Python to mitigate SQL injection risks?
Validating and sanitizing user inputs is an essential step in mitigating SQL injection risks. Here are some strategies to achieve this in Python:
Input Validation: Validate user inputs to ensure they conform to expected formats. Use regular expressions or built-in validation methods to check the input.
import re def validate_username(username): if re.match(r'^[a-zA-Z0-9_]{3,20}$', username): return True return False user_input = "Robert'); DROP TABLE Students;--" if validate_username(user_input): print("Valid username") else: print("Invalid username")
Copy after loginSanitization: Sanitize inputs to remove or escape any potentially harmful characters. However, sanitization alone is not sufficient to prevent SQL injection; it should be used in conjunction with parameterized queries.
import html def sanitize_input(input_string): return html.escape(input_string) user_input = "Robert'); DROP TABLE Students;--" sanitized_input = sanitize_input(user_input) print(sanitized_input) # Output: Robert'); DROP TABLE Students;--
Copy after loginWhitelist Approach: Only allow specific, known-safe inputs. This can be particularly useful for dropdown menus or other controlled input fields.
def validate_selection(selection): allowed_selections = ['option1', 'option2', 'option3'] if selection in allowed_selections: return True return False user_input = "option1" if validate_selection(user_input): print("Valid selection") else: print("Invalid selection")
Copy after loginLength and Type Checking: Ensure that the input length and type match the expected values. This can help prevent buffer overflows and other types of attacks.
def validate_length_and_type(input_string, max_length, expected_type): if len(input_string) <= max_length and isinstance(input_string, expected_type): return True return False user_input = "Robert'); DROP TABLE Students;--" if validate_length_and_type(user_input, 50, str): print("Valid input") else: print("Invalid input")
Copy after loginUse of Libraries: Libraries like
bleach
can be used to sanitize HTML inputs, which can be useful if you're dealing with user-generated content.import bleach user_input = "<script>alert('XSS')</script>" sanitized_input = bleach.clean(user_input) print(sanitized_input) # Output: <script>alert('XSS')</script>
Copy after login
By combining these validation and sanitization techniques with the use of parameterized queries, you can significantly reduce the risk of SQL injection attacks in your Python applications.
The above is the detailed content of How can you prevent SQL injection vulnerabilities in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










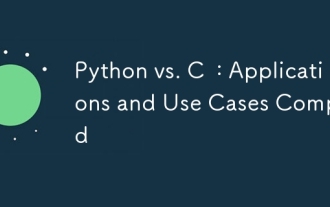
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
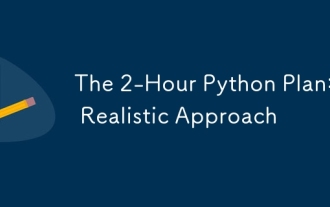
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
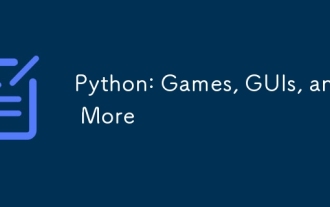
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
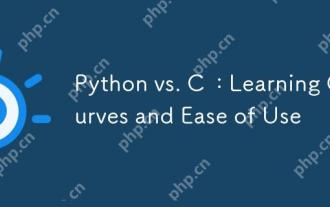
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
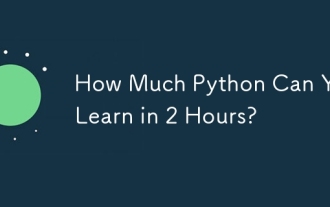
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
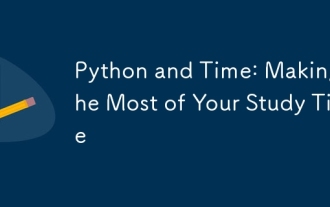
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
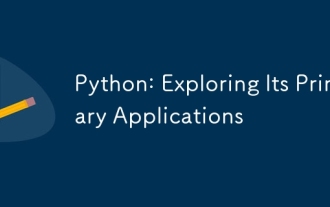
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
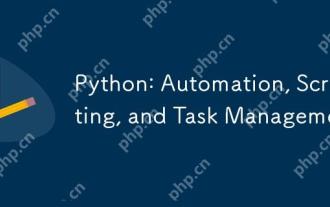
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
