


How can you use Pandas to clean, transform, and analyze data in Python?
How can you use Pandas to clean, transform, and analyze data in Python?
Pandas is a powerful Python library designed for data manipulation and analysis, which makes it an excellent tool for cleaning, transforming, and analyzing data. Here’s a comprehensive look at how you can use Pandas for these purposes:
Data Cleaning:
Pandas provides numerous functions to handle missing data, duplicates, and incorrect formats. You can use methods like dropna()
to remove missing values, fillna()
to replace missing values with a specified value or method, and drop_duplicates()
to remove duplicate rows. Additionally, you can use replace()
and str
accessor methods to clean up text data.
Data Transformation:
Transforming data with Pandas involves reshaping and restructuring your dataset to suit your analysis needs. You can use melt()
, pivot()
, and pivot_table()
for reshaping data, groupby()
for aggregation and transformation based on groups, and apply()
for custom transformations. Methods like map()
, applymap()
, and replace()
are useful for applying functions to series or dataframes.
Data Analysis:
Pandas excels in data analysis tasks. You can perform descriptive statistics using methods like describe()
, mean()
, median()
, and std()
. For more complex analyses, you can use groupby()
in combination with aggregation functions to derive insights from grouped data. You can also use rolling()
and expanding()
to analyze time-series data.
Overall, Pandas offers a rich set of tools that streamline the process of cleaning, transforming, and analyzing data, making it indispensable for data scientists and analysts working in Python.
What are the best practices for data cleaning using Pandas in Python?
Effective data cleaning is crucial for reliable analysis, and Pandas provides a variety of methods to achieve this. Here are some best practices for data cleaning using Pandas:
-
Handling Missing Data:
- Use
isna()
ornotna()
to identify missing values. - Decide whether to drop rows/columns with missing values using
dropna()
or fill them withfillna()
. Consider the context of your data to choose the appropriate strategy.
- Use
-
Removing Duplicates:
- Use
drop_duplicates()
to remove duplicate rows. Consider specifying a subset of columns if only certain columns are relevant for identifying duplicates.
- Use
-
Correcting Data Types:
- Ensure columns have the correct data type using methods like
astype()
orpd.to_numeric()
,pd.to_datetime()
for numeric and datetime data, respectively.
- Ensure columns have the correct data type using methods like
-
Standardizing and Cleaning Text Data:
- Use the
str
accessor to apply string methods likelower()
,upper()
,strip()
, andreplace()
to standardize text data.
- Use the
-
Outlier Detection and Handling:
- Use statistical methods like
describe()
,boxplot()
, andhist()
to identify outliers. You can then decide to either remove them or cap them using techniques likeclip()
.
- Use statistical methods like
-
Validation and Consistency Checks:
- Use
apply()
ormap()
to apply custom validation functions and ensure data consistency across your dataset.
- Use
By following these best practices, you can ensure your dataset is clean and ready for analysis.
How can Pandas be utilized to transform datasets efficiently in Python?
Pandas offers several efficient ways to transform datasets, making it easier to prepare data for analysis or further processing. Here are some key methods:
-
Reshaping Data:
-
melt()
is useful for converting a DataFrame from wide format to long format, making it easier to work with in certain analysis scenarios. -
pivot()
andpivot_table()
help convert long format data back to wide format or create summary statistics.
-
-
Aggregation and Grouping:
-
groupby()
is essential for grouping data and applying aggregate functions likesum()
,mean()
, or custom functions. - Use
agg()
to apply multiple aggregation functions at once.
-
-
Applying Functions:
-
apply()
andapplymap()
allow you to apply a function along an axis of the DataFrame or element-wise. -
map()
is useful for applying a function to a Series to replace values.
-
-
Combining DataFrames:
-
merge()
,join()
, andconcat()
allow you to combine different DataFrames based on a key or index.
-
-
Time Series Transformations:
- Use
resample()
for time-based resampling,rolling()
for rolling window calculations, andexpanding()
for cumulative calculations.
- Use
By utilizing these transformation methods, you can efficiently prepare your data for analysis or further processing, making your workflow more streamlined and effective.
What types of data analysis can be performed using Pandas in Python?
Pandas is versatile and can be used for a wide range of data analysis tasks. Here are some of the key types of analysis you can perform using Pandas:
-
Descriptive Statistics:
- Use
describe()
to get summary statistics like mean, median, min, max, and standard deviation for numerical columns. -
value_counts()
can help analyze the frequency of unique values in a column.
- Use
-
Time Series Analysis:
- Utilize
resample()
,rolling()
, andexpanding()
to analyze time series data and perform operations like calculating moving averages or resampling to different frequencies.
- Utilize
-
Grouped Analysis:
-
groupby()
allows you to perform operations on groups of data, such as calculating aggregated statistics for different categories.
-
-
Correlation and Covariance:
- Use
corr()
andcov()
to compute the correlation and covariance between columns, helping to understand relationships in the data.
- Use
-
Data Visualization:
- While Pandas itself does not create plots, it integrates seamlessly with libraries like Matplotlib and Seaborn. Methods like
plot()
,hist()
, andboxplot()
can be used to quickly visualize data.
- While Pandas itself does not create plots, it integrates seamlessly with libraries like Matplotlib and Seaborn. Methods like
-
Pivot Tables and Cross-Tabulation:
-
pivot_table()
andcrosstab()
are powerful tools for creating summary statistics and analyzing multi-dimensional data.
-
-
Custom Analysis:
- Use
apply()
to apply custom functions to your data, allowing for flexible and tailored analysis.
- Use
By leveraging these capabilities, Pandas can help you conduct thorough and varied data analysis, making it an essential tool in the data scientist’s toolkit.
The above is the detailed content of How can you use Pandas to clean, transform, and analyze data in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
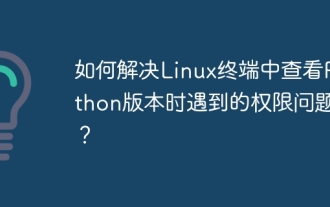
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
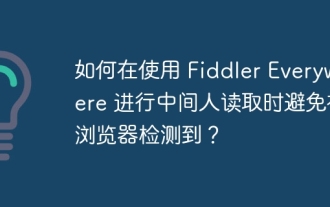
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
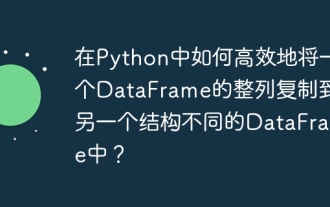
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
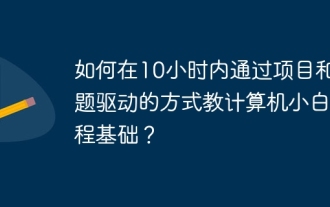
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
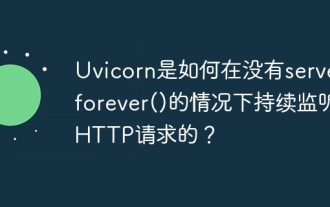
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
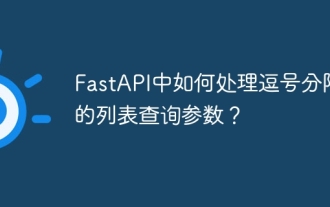
Fastapi ...
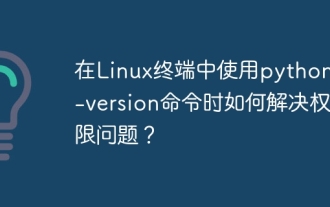
Using python in Linux terminal...
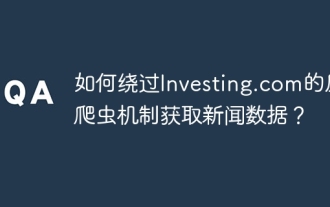
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
