How can you optimize database queries in Python?
How can you optimize database queries in Python?
Optimizing database queries in Python is crucial for enhancing the performance of your application. Here are several strategies that can be employed:
-
Use Efficient Query Structures: Construct your queries to fetch only the data that is needed. This can be achieved by specifying the columns you need rather than using
SELECT *
, which retrieves all columns and can be resource-intensive. -
Limit Data Retrieval: Use
LIMIT
or equivalent in your database system to restrict the number of rows returned. This is particularly useful when you need to paginate results or when you're dealing with large datasets. -
Avoid N 1 Query Problem: The N 1 query problem occurs when you fetch a list of objects, and then for each object in the list, you fetch additional data, resulting in many additional queries. To avoid this, use eager loading where possible. In SQLAlchemy, you can use
joinedload
orsubqueryload
to pre-load related objects. -
Use Appropriate Data Types: Ensure that you are using the most appropriate data types for your columns. For example, using
DATETIME
for date fields instead ofVARCHAR
can help improve query performance. - Optimize JOINs: Be careful with JOIN operations, as they can significantly slow down queries. Use INNER JOINs when possible, and consider using EXISTS instead of IN if you're checking for the existence of records.
- Batch Operations: If you need to insert or update multiple rows, consider using batch operations. Most database engines allow you to perform multiple operations in a single query, which is more efficient than running multiple individual queries.
- Caching: Implement caching mechanisms to store the results of frequently accessed queries. This can drastically reduce the load on your database.
-
Profile and Monitor: Use profiling tools to identify slow queries and monitor their performance over time. Tools like
cProfile
in Python can help identify bottlenecks in your code.
What are some common techniques for improving query performance in Python?
Improving query performance in Python can be approached from several angles:
- Indexing: Proper indexing can dramatically speed up query times. Indexes help the database find data quickly without having to scan every row in a table.
- Query Optimization: Analyze and rewrite queries to make them more efficient. Tools like EXPLAIN can help you understand how the database is executing your query and where bottlenecks might be.
- Connection Pooling: Implement connection pooling to manage database connections efficiently. This reduces the overhead of opening and closing database connections repeatedly.
-
Asynchronous Queries: Use asynchronous programming to perform database operations. Libraries like
asyncpg
for PostgreSQL can help manage database operations without blocking other operations in your application. - Database Sharding: For very large datasets, consider implementing database sharding to distribute data across multiple servers, reducing the load on any single database.
- Denormalization: In some cases, denormalization (intentionally duplicating data to speed up reads) can be beneficial, although it needs to be managed carefully to keep data consistent.
- Use of ORM Optimizations: If using an ORM like SQLAlchemy, take advantage of its optimizations features, such as lazy loading and eager loading, to manage how and when data is fetched from the database.
How can indexing help in optimizing database queries in Python?
Indexing is one of the most effective ways to optimize database queries in Python because it allows the database engine to quickly locate data without having to scan every row in a table. Here’s how indexing can help:
- Faster Data Retrieval: Indexes work like the index of a book, allowing the database to jump directly to the relevant data. This significantly reduces the time required to retrieve data, especially for large tables.
- Reduced I/O Operations: By limiting the amount of data that needs to be read from disk, indexing can reduce the I/O operations, which are typically a major performance bottleneck.
- Efficient JOIN Operations: Indexes can speed up JOIN operations by allowing the database to quickly find matching rows between tables.
- Support for Unique Constraints: Indexes can enforce uniqueness, ensuring data integrity, and can speed up queries that check for uniqueness.
- Full-Text Search: For databases that support it, full-text indexes can dramatically speed up text searches, making them more efficient and powerful.
When using Python to interact with databases, you can create indexes through your SQL queries or by using ORM features. For instance, in SQLAlchemy, you can define indexes when you're creating your model classes:
from sqlalchemy import Index class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) email = Column(String) __table_args__ = (Index('idx_name_email', 'name', 'email'),)
This example adds a composite index on the name
and email
fields, which can optimize queries involving these columns.
What tools or libraries in Python can assist with database query optimization?
Several tools and libraries in Python can assist with database query optimization:
- SQLAlchemy: A popular ORM that provides a high-level interface for database interactions. SQLAlchemy includes features like eager loading, which helps avoid the N 1 query problem, and can also be used to create indexes and manage database connections efficiently.
- Pandas: While primarily a data manipulation library, Pandas can be used to analyze and process data retrieved from databases. You can use it to optimize data retrieval by processing data in memory after fetching it from the database.
- psycopg2: A PostgreSQL adapter for Python that supports features like prepared statements, which can be used to optimize repeated queries.
- asyncpg: An asynchronous PostgreSQL driver for Python that can help in managing database operations without blocking other operations in your application, thus improving overall performance.
- Django ORM: If you're using Django, its ORM provides various optimizations like select_related and prefetch_related to optimize database queries.
- cProfile: A built-in Python profiling tool that can help identify bottlenecks in your code, including database operations.
- pgadmin: While not a Python library, pgadmin is a useful tool for PostgreSQL database administration and can be used to analyze and optimize queries.
- EXPLAIN: Not a Python tool per se, but a SQL command that can be executed through Python to analyze the execution plan of a query, helping you understand and optimize it.
By leveraging these tools and libraries, you can significantly enhance the performance of your database queries in Python.
The above is the detailed content of How can you optimize database queries in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










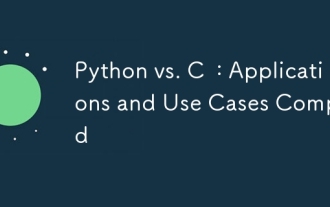
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
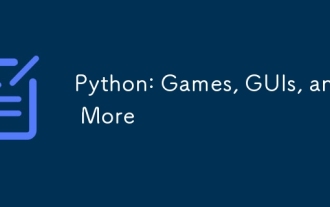
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
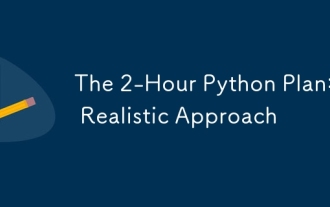
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
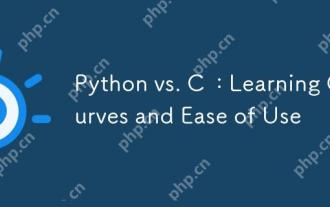
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
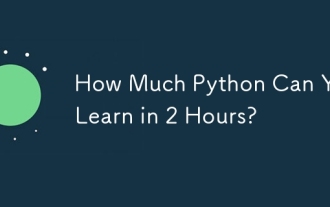
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
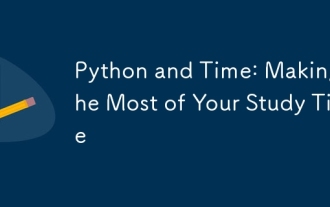
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
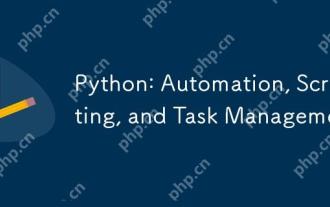
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
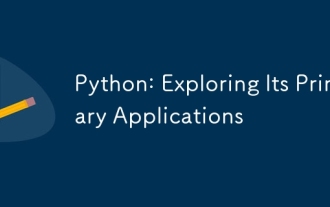
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
