


How can you use goroutine pools to limit the number of concurrent goroutines?
How can you use goroutine pools to limit the number of concurrent goroutines?
Goroutine pools are used to manage and limit the number of concurrent goroutines in a Go program. This is particularly useful when you want to control the level of concurrency to prevent overwhelming system resources or to ensure that the program behaves predictably under high load. Here's how you can use goroutine pools to achieve this:
- Creation of a Pool: First, you create a pool of goroutines. This pool typically consists of a fixed number of worker goroutines that are ready to execute tasks.
- Task Submission: When a task needs to be executed, it is submitted to the pool. The pool manages a queue of tasks waiting to be processed.
- Task Execution: The worker goroutines in the pool pick up tasks from the queue and execute them. Since the number of worker goroutines is fixed, the number of concurrently running goroutines is limited to the size of the pool.
- Completion and Reuse: Once a worker goroutine completes a task, it returns to the pool and is ready to pick up another task. This allows for efficient reuse of goroutines.
By using a goroutine pool, you ensure that no more than the specified number of goroutines are running at any given time, thus controlling the level of concurrency.
What are the benefits of using goroutine pools for managing concurrency in Go?
Using goroutine pools to manage concurrency in Go offers several benefits:
- Resource Management: Goroutine pools help in managing system resources more efficiently. By limiting the number of concurrent goroutines, you prevent the system from being overwhelmed, which can lead to better performance and stability.
- Predictable Behavior: With a fixed number of goroutines, the behavior of the program becomes more predictable. This is particularly important in production environments where consistent performance is crucial.
- Efficient Reuse: Goroutine pools allow for the efficient reuse of goroutines. Instead of creating and destroying goroutines for each task, the same goroutines are reused, reducing the overhead associated with goroutine creation and termination.
- Scalability: Goroutine pools can be easily scaled by adjusting the size of the pool. This allows you to fine-tune the level of concurrency based on the specific needs of your application.
- Simplified Error Handling: With a fixed number of goroutines, it becomes easier to manage and handle errors. You can implement centralized error handling mechanisms within the pool, making it easier to debug and maintain the application.
How do you implement a goroutine pool to control the number of simultaneous goroutines?
Implementing a goroutine pool in Go involves creating a structure to manage the pool and its workers. Here's a basic example of how you can implement a goroutine pool:
package main import ( "fmt" "sync" ) type Task func() type Pool struct { workers int taskQueue chan Task wg sync.WaitGroup } func NewPool(workers int) *Pool { p := Pool{ workers: workers, taskQueue: make(chan Task), } return p } func (p *Pool) Run() { for i := 0; i < p.workers; i { go func() { for task := range p.taskQueue { task() p.wg.Done() } }() } } func (p *Pool) Submit(task Task) { p.wg.Add(1) p.taskQueue <- task } func (p *Pool) Shutdown() { close(p.taskQueue) p.wg.Wait() } func main() { pool := NewPool(5) // Create a pool with 5 workers pool.Run() for i := 0; i < 10; i { task := func() { fmt.Printf("Task %d executed\n", i) } pool.Submit(task) } pool.Shutdown() }
In this example:
-
Pool Structure: The
Pool
struct contains the number of workers, a channel for the task queue, and async.WaitGroup
to manage the completion of tasks. - NewPool Function: This function initializes a new pool with the specified number of workers.
- Run Method: This method starts the worker goroutines. Each worker continuously pulls tasks from the task queue and executes them.
-
Submit Method: This method adds a new task to the task queue and increments the
WaitGroup
counter. -
Shutdown Method: This method closes the task queue and waits for all tasks to complete using the
WaitGroup
.
By using this implementation, you can control the number of simultaneous goroutines to the specified number of workers.
What are the potential drawbacks of using goroutine pools to manage concurrency?
While goroutine pools offer several benefits, there are also potential drawbacks to consider:
- Complexity: Implementing and managing a goroutine pool can add complexity to your code. You need to handle the creation of the pool, task submission, and proper shutdown, which can be error-prone.
- Overhead: There is some overhead associated with managing a goroutine pool, such as maintaining the task queue and coordinating the workers. This overhead might not be justified for simple applications with low concurrency needs.
- Fixed Concurrency: By using a fixed number of workers, you might not be able to take full advantage of the available system resources. If the system has more capacity, a fixed pool size might limit the overall performance.
- Potential Bottlenecks: If the task queue grows too large, it can become a bottleneck. Tasks might wait in the queue for a long time before being executed, leading to increased latency.
- Difficulty in Scaling: While you can adjust the size of the pool, doing so dynamically based on the current load can be challenging. This might require additional logic to monitor and adjust the pool size, adding further complexity.
In summary, while goroutine pools are a powerful tool for managing concurrency in Go, they come with trade-offs that need to be carefully considered based on the specific requirements of your application.
The above is the detailed content of How can you use goroutine pools to limit the number of concurrent goroutines?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










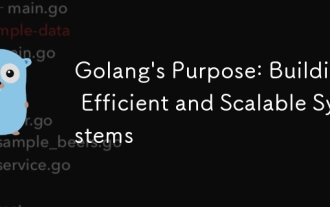
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
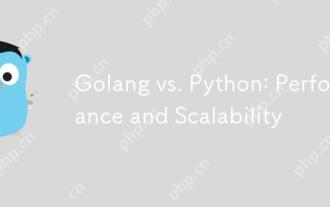
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
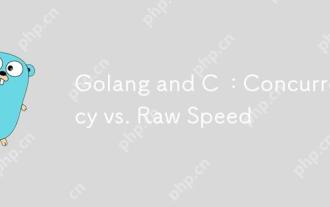
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
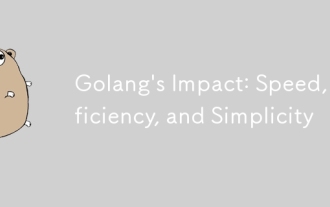
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
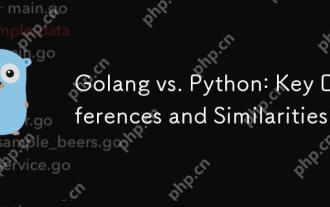
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
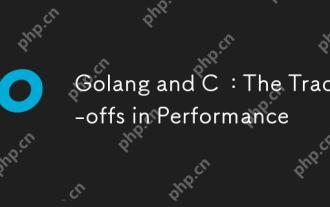
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
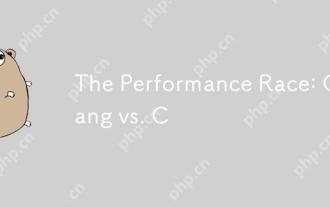
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
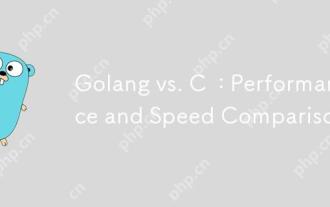
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
