


What is static analysis? How can you use tools like flake8 and pylint to improve code quality?
The article discusses static analysis in software development, focusing on tools like flake8 and pylint to enhance code quality. It explains how these tools detect various code issues and reduce debugging time.
What is static analysis? How can you use tools like flake8 and pylint to improve code quality?
Static analysis is a method of evaluating software code without executing it. It involves analyzing the code's structure, syntax, and style to identify potential errors, security vulnerabilities, and areas for improvement. By using static analysis tools, developers can catch issues early in the development process, which leads to better code quality and more reliable software.
Tools like flake8 and pylint are widely used in the Python programming community to enhance code quality. Flake8 is a combination of several tools: PyFlakes for syntax errors, pycodestyle for style checks, and McCabe for complexity checks. Pylint, on the other hand, is a more comprehensive tool that not only checks for errors and style but also evaluates the overall quality of the code through a scoring system.
To improve code quality using these tools, you can integrate them into your development workflow in several ways:
- Pre-commit Hooks: Configure flake8 and pylint to run automatically before committing code to a version control system. This ensures that the code adheres to the defined standards before it is merged into the main branch.
- Continuous Integration (CI) Pipelines: Integrate these tools into your CI/CD pipeline to automatically analyze the code every time a new commit is pushed. This helps maintain a high standard of code quality across the entire project.
- IDE Integration: Many integrated development environments (IDEs) support plugins for flake8 and pylint, allowing developers to receive real-time feedback on their code as they write it. This immediate feedback can help catch issues early and improve coding habits.
- Code Reviews: Use the reports generated by flake8 and pylint during code reviews to discuss and address code quality issues. This collaborative approach can lead to better adherence to coding standards and more consistent code across the team.
By leveraging these tools in your development process, you can significantly improve the quality of your code, leading to fewer bugs and more maintainable software.
What specific code issues can flake8 and pylint detect?
Flake8 and pylint are capable of detecting a wide range of code issues, each with its own focus and capabilities. Here are some specific issues they can identify:
Flake8:
- Syntax Errors: Flake8 can catch common syntax errors that would prevent the code from running, such as undefined variables, missing colons, and incorrect indentation.
- Style Violations: It checks for style issues based on PEP 8, the style guide for Python code. This includes improper spacing, line length, and naming conventions.
- Complexity: Flake8 uses the McCabe complexity checker to identify functions that are too complex and may need refactoring. It measures the cyclomatic complexity, which is the number of linearly independent paths through the source code.
- Unused Imports and Variables: Flake8 can identify unused imports and variables, helping to clean up the code and prevent unnecessary clutter.
Pylint:
- Syntax and Style: Similar to flake8, pylint checks for syntax errors and adherence to PEP 8 style guidelines. However, it goes beyond basic checks and can provide more detailed feedback on style issues.
- Code Duplication: Pylint can detect duplicated code blocks, which can be a sign of poor design and potential maintenance issues.
- Refactoring Opportunities: It suggests refactoring opportunities, such as splitting large functions or classes, to improve code readability and maintainability.
- Security Issues: Pylint can identify potential security vulnerabilities, such as SQL injection or insecure use of cryptographic functions.
- Code Smells: It detects code smells, which are structures in code that, while not necessarily bugs, may indicate deeper problems and should be refactored.
- Documentation: Pylint checks for the presence and quality of documentation, ensuring that functions and classes are properly documented.
By using these tools, developers can address a broad spectrum of issues, from simple style violations to complex security concerns, ultimately leading to more robust and maintainable code.
How do flake8 and pylint differ in their approach to code analysis?
Flake8 and pylint both serve the purpose of improving code quality, but they differ in their approach and the depth of their analysis:
Flake8:
- Modularity: Flake8 is a wrapper around three separate tools: PyFlakes, pycodestyle, and McCabe. This modular approach allows developers to focus on specific aspects of code quality, such as syntax, style, and complexity.
- Speed and Lightweight: Flake8 is known for being fast and lightweight, making it ideal for quick checks during development. It is designed to be less intrusive and focuses primarily on catching errors and enforcing style guidelines.
- Simple Output: The output of flake8 is straightforward and easy to understand, focusing on specific errors or violations. It reports issues with clear codes that correspond to different types of problems, making it easier to identify and fix them.
- Customizability: While flake8 can be customized to some extent, its configuration options are less extensive compared to pylint. It is designed to be used with default settings in many cases.
Pylint:
- Comprehensive Analysis: Pylint provides a more comprehensive analysis of the code. It goes beyond basic syntax and style checks to evaluate the overall quality and structure of the code. It includes checks for code duplication, refactoring opportunities, and even potential security issues.
- Scoring System: One of the unique features of pylint is its scoring system, which rates the code on a scale from 0 to 10 based on various criteria. This provides a quick overview of the code's overall quality and helps prioritize areas for improvement.
- Detailed Feedback: Pylint offers more detailed feedback and explanations for the issues it detects. It not only points out problems but also provides suggestions for improvement, making it a valuable tool for learning and improving coding practices.
- Extensive Configuration: Pylint allows for extensive customization. Developers can configure a wide range of settings to suit their project's specific needs, including setting custom rules and thresholds for different types of issues.
In summary, while flake8 is faster and more focused on catching specific errors and enforcing style, pylint provides a deeper and more comprehensive analysis of the code. The choice between the two often depends on the specific needs of the project and the desired level of detail in the analysis.
Can using static analysis tools like flake8 and pylint reduce debugging time?
Yes, using static analysis tools like flake8 and pylint can significantly reduce debugging time. Here's how they contribute to a more efficient debugging process:
- Early Detection of Issues: Static analysis tools catch many common issues before the code is executed. By identifying syntax errors, style violations, and potential bugs early in the development cycle, these tools help developers fix problems before they become part of the larger codebase. This early detection reduces the time spent debugging issues during later stages of development.
- Reduced Complexity: Tools like flake8 and pylint can identify complex code that may be prone to errors. By flagging functions with high cyclomatic complexity or suggesting refactoring opportunities, they encourage developers to write simpler, more maintainable code. Simpler code is easier to debug and maintain, which can lead to significant time savings.
- Consistency and Standards: These tools enforce coding standards and best practices, ensuring that the codebase remains consistent. A consistent codebase is easier to navigate and understand, making it easier to identify and fix bugs. When all team members follow the same coding standards, it reduces the likelihood of introducing errors due to inconsistent coding practices.
- Automation in CI/CD: Integrating flake8 and pylint into continuous integration pipelines automates the detection of issues with every commit. This automation means that bugs are caught and addressed as soon as they are introduced, preventing them from accumulating and becoming harder to fix over time. Automated checks save developers from the tedious task of manually reviewing code for common issues.
- Improved Code Quality: By regularly using static analysis tools, developers can improve the overall quality of their code. Higher quality code has fewer bugs and is easier to debug. As a result, the time spent on debugging is reduced because there are fewer issues to resolve.
- Focus on Critical Issues: With static analysis tools handling the detection of common errors and style issues, developers can focus their debugging efforts on more critical and complex problems. This targeted approach to debugging can lead to more efficient resolution of issues.
In conclusion, static analysis tools like flake8 and pylint play a crucial role in reducing debugging time by catching issues early, promoting code simplicity and consistency, automating quality checks, and allowing developers to focus on more complex problems. Integrating these tools into the development process can lead to more efficient debugging and, ultimately, more reliable software.
The above is the detailed content of What is static analysis? How can you use tools like flake8 and pylint to improve code quality?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
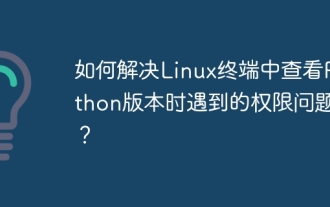
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
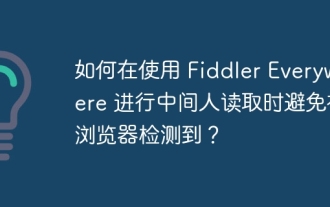
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
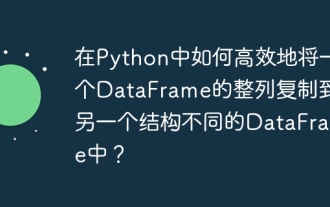
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
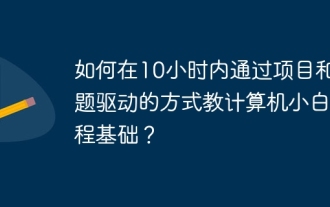
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
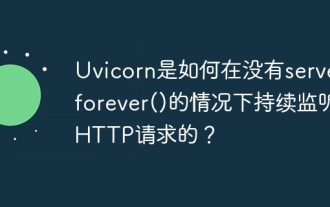
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
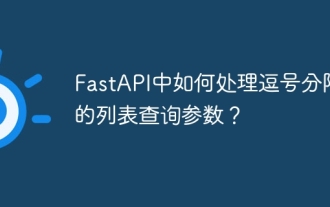
Fastapi ...
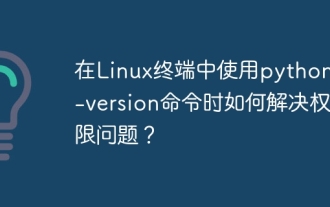
Using python in Linux terminal...
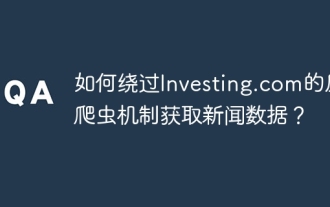
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
