


What is service discovery? How can you implement service discovery in Go (e.g., using Consul, etcd)?
Article discusses service discovery in microservices, focusing on implementation in Go using Consul and etcd. It highlights benefits and scalability improvements.Character count: 159
What is service discovery? How can you implement service discovery in Go (e.g., using Consul, etcd)?
Service discovery is a mechanism that allows services to find and communicate with each other dynamically in a distributed system. It is particularly useful in microservices architectures where services are developed, deployed, and scaled independently. Service discovery systems maintain a registry of available services and their locations, making it easier for services to connect without hardcoding endpoint details.
To implement service discovery in Go, you can use popular tools like Consul or etcd. Here's how you can do it with both:
Using Consul:
Consul is a service mesh solution that provides service discovery, configuration, and segmentation in one tool. To use Consul with Go, you'll need to use the consul/api
package. Here’s a basic example of how to register a service and discover it:
-
Install the Consul client:
go get github.com/hashicorp/consul/api
Copy after login Register a service:
package main import ( "fmt" "github.com/hashicorp/consul/api" ) func main() { config := api.DefaultConfig() client, err := api.NewClient(config) if err != nil { panic(err) } registration := new(api.AgentServiceRegistration) registration.Name = "my-service" registration.Port = 8080 registration.Address = "localhost" err = client.Agent().ServiceRegister(registration) if err != nil { panic(err) } fmt.Println("Service registered successfully") }
Copy after loginDiscover a service:
package main import ( "fmt" "github.com/hashicorp/consul/api" ) func main() { config := api.DefaultConfig() client, err := api.NewClient(config) if err != nil { panic(err) } services, _, err := client.Health().Service("my-service", "", true, &api.QueryOptions{}) if err != nil { panic(err) } for _, service := range services { fmt.Printf("Service: %s, Address: %s:%d\n", service.Service.Name, service.Service.Address, service.Service.Port) } }
Copy after login
Using etcd:
etcd is a distributed key-value store that can be used for service discovery. To use etcd with Go, you'll need the clientv3
package. Here’s a basic example of how to register a service and discover it:
Install the etcd client:
go get go.etcd.io/etcd/client/v3
Copy after loginRegister a service:
package main import ( "context" "fmt" "go.etcd.io/etcd/client/v3" ) func main() { cli, err := clientv3.New(clientv3.Config{ Endpoints: []string{"localhost:2379"}, }) if err != nil { panic(err) } defer cli.Close() _, err = cli.Put(context.Background(), "/services/my-service", "localhost:8080") if err != nil { panic(err) } fmt.Println("Service registered successfully") }
Copy after loginDiscover a service:
package main import ( "context" "fmt" "go.etcd.io/etcd/client/v3" ) func main() { cli, err := clientv3.New(clientv3.Config{ Endpoints: []string{"localhost:2379"}, }) if err != nil { panic(err) } defer cli.Close() resp, err := cli.Get(context.Background(), "/services/my-service") if err != nil { panic(err) } for _, kv := range resp.Kvs { fmt.Printf("Service: my-service, Address: %s\n", string(kv.Value)) } }
Copy after login
What are the benefits of using service discovery in a microservices architecture?
Using service discovery in a microservices architecture offers several benefits:
-
Dynamic Service Registration and Discovery:
Services can register themselves upon startup and deregister upon shutdown. Other services can discover these services dynamically, eliminating the need for hardcoded endpoint configurations. -
Scalability:
Service discovery allows for easy scaling of services. As new instances of a service are added or removed, the service registry is updated, and other services can find the new instances without manual intervention. -
Load Balancing:
Many service discovery tools provide built-in load balancing capabilities, distributing requests across multiple instances of a service to ensure optimal resource utilization and performance. -
Fault Tolerance:
Service discovery systems can detect when a service instance is down and remove it from the registry, ensuring that requests are not sent to unavailable services. -
Decoupling:
Services are decoupled from each other, allowing for independent development, deployment, and scaling. This promotes a more modular and flexible architecture. -
Simplified Configuration Management:
Service discovery reduces the need for complex configuration management, as services can automatically find and connect to each other.
How does service discovery improve the scalability of applications built with Go?
Service discovery significantly improves the scalability of applications built with Go in several ways:
-
Automatic Instance Management:
As new instances of a service are spun up or taken down, service discovery automatically updates the registry. This allows Go applications to scale horizontally without manual configuration changes. -
Load Balancing:
Service discovery tools often include load balancing features that distribute traffic across multiple instances of a service. This ensures that no single instance becomes a bottleneck, allowing Go applications to handle increased load efficiently. -
Efficient Resource Utilization:
By dynamically adjusting to the number of service instances, service discovery helps Go applications make better use of available resources. This is particularly important in cloud environments where resources can be dynamically allocated. -
Simplified Deployment:
With service discovery, deploying new versions of services or scaling existing ones becomes easier. Go applications can be updated and scaled without affecting the overall system, as other services will automatically discover the new instances. -
Resilience and Fault Tolerance:
Service discovery helps Go applications maintain high availability by quickly detecting and rerouting around failed instances. This resilience is crucial for scalable systems that need to handle failures gracefully.
What are the key differences between Consul and etcd when used for service discovery in Go?
When considering Consul and etcd for service discovery in Go, there are several key differences to keep in mind:
-
Architecture and Features:
- Consul: Consul is a more comprehensive service mesh solution that includes service discovery, health checking, key-value storage, and multi-datacenter support. It also provides built-in DNS and HTTP interfaces for service discovery.
- etcd: etcd is primarily a distributed key-value store designed for reliability and consistency. While it can be used for service discovery, it lacks the additional features like health checking and multi-datacenter support that Consul offers.
-
Ease of Use:
- Consul: Consul provides a more user-friendly interface for service discovery, with built-in support for service registration and discovery through its API. It also offers a web UI for easier management.
- etcd: Using etcd for service discovery requires more manual configuration, as you need to manage the key-value pairs yourself. However, it is straightforward to use if you are already familiar with key-value stores.
-
Performance:
- Consul: Consul is optimized for service discovery and can handle high volumes of service registrations and lookups efficiently. It also includes features like gossip protocol for faster service discovery.
- etcd: etcd is designed for consistency and reliability, which can sometimes result in higher latency compared to Consul. However, it is highly reliable and suitable for applications requiring strong consistency.
-
Integration and Ecosystem:
- Consul: Consul has a rich ecosystem with many integrations and tools, making it easier to integrate into existing systems. It is widely used in the service mesh space, particularly with tools like Envoy.
- etcd: etcd is commonly used in Kubernetes for configuration management and service discovery. It has a strong presence in the container orchestration space but may require additional tools for a complete service discovery solution.
-
Security:
- Consul: Consul provides robust security features, including ACLs (Access Control Lists) and encryption of data in transit. It is designed to be secure out of the box.
- etcd: etcd also supports encryption and authentication, but setting up these features requires more manual configuration compared to Consul.
In summary, Consul is a more feature-rich solution that is easier to use for service discovery, while etcd is a reliable key-value store that can be used for service discovery with additional configuration. The choice between them depends on the specific needs of your Go application and the existing ecosystem you are working within.
The above is the detailed content of What is service discovery? How can you implement service discovery in Go (e.g., using Consul, etcd)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










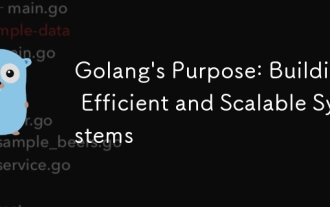
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
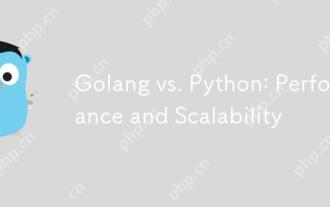
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
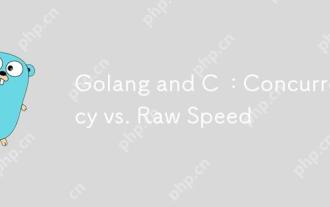
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
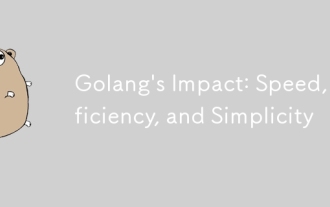
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
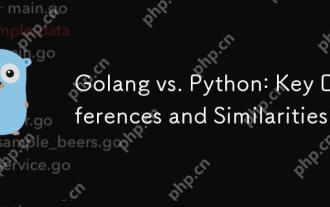
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
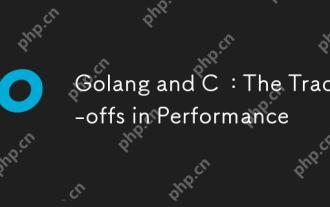
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
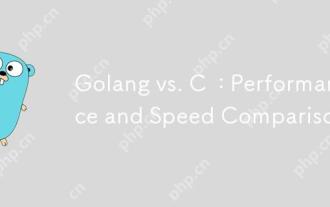
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
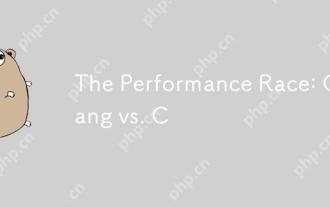
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
