


How can you use Go to build message queues (e.g., using Kafka, RabbitMQ)?
How can you use Go to build message queues (e.g., using Kafka, RabbitMQ)?
To build message queues using Go, you can leverage the language's efficiency and robust standard library to interface with systems like Kafka and RabbitMQ. Here's a breakdown of how you can use Go with these technologies:
-
Using Kafka:
-
Producer/Consumer Model: You can write a Kafka producer in Go to send messages to topics and a consumer to read from those topics. The
sarama
library is commonly used for this purpose, as it provides an easy-to-use interface for Kafka operations. -
Example Code: For a producer, you would initialize a
sarama.SyncProducer
, specify the topic, and then useSendMessage
to send the message. Similarly, a consumer would initialize asarama.ConsumerGroup
, join a group, and process messages from the assigned partitions. - Integration with Applications: Go applications can easily integrate Kafka for use cases such as log aggregation, event streaming, or as part of a microservices architecture.
-
Producer/Consumer Model: You can write a Kafka producer in Go to send messages to topics and a consumer to read from those topics. The
-
Using RabbitMQ:
-
AMQP Protocol: Go supports the AMQP protocol through libraries like
streadway/amqp
. You can establish a connection to a RabbitMQ server, declare queues, and publish/consume messages. -
Example Code: You'd use
amqp.Dial
to connect to the RabbitMQ server,amqp.Channel
to create a channel, and thenPublish
andConsume
methods to interact with the queue. - Use Cases: RabbitMQ can be used in Go applications for task distribution, workflow processing, or even as a simple message bus in smaller-scale applications.
-
AMQP Protocol: Go supports the AMQP protocol through libraries like
By using Go with Kafka or RabbitMQ, you can build scalable and efficient message queue systems that are crucial for modern distributed systems.
What are the performance benefits of using Go for implementing message queues like Kafka or RabbitMQ?
Go offers several performance benefits when used for implementing message queues such as Kafka or RabbitMQ:
- Concurrency: Go's lightweight goroutines and channels enable efficient concurrent handling of message operations. This is particularly beneficial for managing high volumes of messages in a scalable manner.
- Low Latency: Go compiles to native code, which results in fast execution times. This is crucial for real-time systems where messages need to be processed quickly.
- Memory Efficiency: Go's garbage collection and memory management help maintain low memory usage, even when dealing with a large number of messages.
- Robust Standard Library: Go's standard library includes networking and I/O packages that are highly optimized, reducing the overhead of interacting with external systems like Kafka or RabbitMQ.
- Scalability: Go's design allows applications to scale easily, which is important for handling varying loads of message processing.
These performance benefits make Go an excellent choice for implementing and integrating message queue systems.
Which libraries in Go are recommended for integrating with Kafka or RabbitMQ for message queue systems?
For integrating with Kafka and RabbitMQ in Go, the following libraries are recommended due to their popularity and feature set:
-
Kafka:
- sarama: This is the most widely used Go library for Kafka. It provides both synchronous and asynchronous producers, as well as consumer groups for handling high-throughput message processing.
- confluent-kafka-go: This is an official library from Confluent, the company behind Kafka. It's built on top of librdkafka and offers high performance and a robust feature set.
-
RabbitMQ:
- streadway/amqp: This library provides a complete implementation of the AMQP 0.9.1 protocol, making it suitable for interacting with RabbitMQ. It supports all essential operations like publishing and consuming messages.
- rabbitmq/amqp091-go: This is a maintained fork of the streadway/amqp library, with active development and improvements.
Both sets of libraries are well-documented and widely used in production environments, making them reliable choices for integrating Go with Kafka or RabbitMQ.
How can Go's concurrency features enhance the efficiency of message queues when using Kafka or RabbitMQ?
Go's concurrency features significantly enhance the efficiency of message queues when integrated with systems like Kafka or RabbitMQ:
- Goroutines: These are lightweight threads managed by the Go runtime. They allow you to handle multiple message operations concurrently without the overhead of traditional threads. For example, you can spawn multiple goroutines to process messages from different Kafka partitions or RabbitMQ queues simultaneously.
- Channels: Go's channels provide a safe way to communicate between goroutines. They can be used to pass messages between different parts of your application, ensuring that message processing remains efficient and synchronized. For instance, you can use channels to buffer messages from Kafka or RabbitMQ before processing them.
-
Select Statements: The
select
statement in Go allows you to wait on multiple channel operations. This is useful for managing multiple message streams or handling timeouts and error conditions gracefully in your message queue system. - Concurrency Patterns: Go supports various concurrency patterns like fan-out/fan-in, which can be applied to distribute the workload of processing messages across multiple goroutines and then aggregate the results. This is particularly useful for scaling the processing of high-volume message queues.
By leveraging these concurrency features, Go applications can efficiently handle the demands of message queue systems, ensuring high throughput and low latency in processing messages from Kafka or RabbitMQ.
The above is the detailed content of How can you use Go to build message queues (e.g., using Kafka, RabbitMQ)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










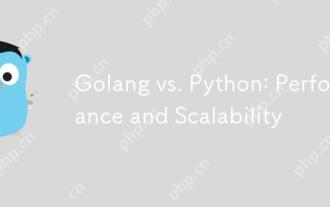
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
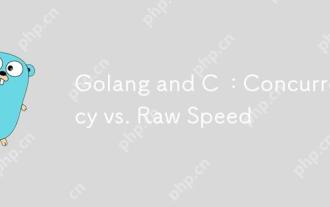
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
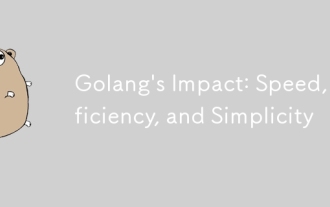
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
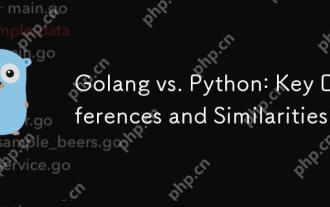
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
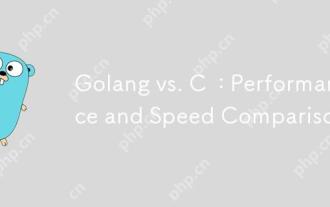
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
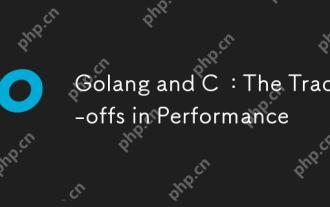
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
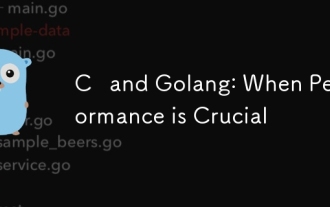
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
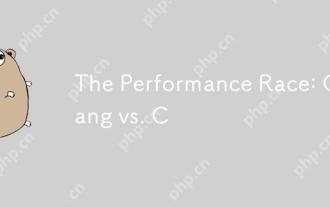
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
