


How can you use the Drag and Drop API to enable drag-and-drop functionality?
Mar 26, 2025 pm 09:04 PMThe article discusses using the Drag and Drop API in HTML5 for web applications, detailing how to make elements draggable, handle drag and drop events, and manage different data types during operations.
How can you use the Drag and Drop API to enable drag-and-drop functionality?
The Drag and Drop API in HTML5 provides a straightforward way to implement drag-and-drop functionality in web applications. To enable this feature, you need to follow these steps:
-
Make an element draggable: Set the
draggable
attribute totrue
on the element you want to make draggable. For example,<div draggable="true">Drag me!</div>
. -
Define drag events on the draggable element: You need to handle several events on the draggable element:
-
dragstart
: This event is fired when the user starts dragging the element. You can use it to set the data to be transferred during the drag operation usingevent.dataTransfer.setData()
. For example:element.addEventListener('dragstart', (e) => { e.dataTransfer.setData('text/plain', element.id); });
Copy after login drag
: This event is fired continuously as the user drags the element. It can be used for visual feedback, such as changing the appearance of the element being dragged.dragend
: This event is fired when the user releases the dragged element. It can be used to clean up any visual changes made during the drag operation.
-
Define drop events on the drop target: You also need to handle events on the element where you want to drop the dragged item:
dragenter
: This event is fired when the dragged element enters the drop target. You can use it to provide visual feedback, such as highlighting the drop target.dragover
: This event is fired continuously as the dragged element is over the drop target. By default, the browser prevents dropping, so you need to prevent the default action to allow dropping:dropTarget.addEventListener('dragover', (e) => { e.preventDefault(); });
Copy after logindragleave
: This event is fired when the dragged element leaves the drop target. You can use it to revert any visual changes made when the dragged element entered the drop target.drop
: This event is fired when the user drops the dragged element on the drop target. You can use it to handle the drop action, such as moving the dragged element to the drop target or processing the transferred data:dropTarget.addEventListener('drop', (e) => { e.preventDefault(); const data = e.dataTransfer.getData('text/plain'); // Handle the dropped data });
Copy after login
By following these steps and handling the appropriate events, you can implement drag-and-drop functionality using the Drag and Drop API.
What are the key events involved in implementing drag-and-drop with the Drag and Drop API?
The key events involved in implementing drag-and-drop functionality with the Drag and Drop API are:
- dragstart: Fired when the user starts dragging an element. This event is used to set the data to be transferred during the drag operation.
- drag: Fired continuously as the user drags the element. This event can be used to provide visual feedback during the drag operation.
- dragend: Fired when the user releases the dragged element. This event can be used to clean up any visual changes made during the drag operation.
- dragenter: Fired when the dragged element enters a valid drop target. This event can be used to provide visual feedback, such as highlighting the drop target.
- dragover: Fired continuously as the dragged element is over a valid drop target. By default, the browser prevents dropping, so you need to prevent the default action to allow dropping.
- dragleave: Fired when the dragged element leaves a valid drop target. This event can be used to revert any visual changes made when the dragged element entered the drop target.
- drop: Fired when the user drops the dragged element on a valid drop target. This event is used to handle the drop action, such as moving the dragged element to the drop target or processing the transferred data.
These events are essential for implementing a complete drag-and-drop functionality using the Drag and Drop API.
Can the Drag and Drop API be used to transfer data between different applications or windows?
The Drag and Drop API is primarily designed for transferring data within a single web application. However, it can be used to transfer data between different applications or windows under certain conditions:
- Within the same browser: You can use the Drag and Drop API to transfer data between different tabs or windows of the same browser. The data is transferred using the
dataTransfer
object, and the receiving application can access the data using thegetData
method. - Between different applications: The Drag and Drop API can be used to transfer data between different applications if the receiving application supports the same data format. For example, you can drag text from a web page to a text editor application. However, the receiving application must be able to handle the data format specified in the
setData
method. - Cross-origin restrictions: When transferring data between different origins (domains), you need to be aware of cross-origin restrictions. The Drag and Drop API follows the same-origin policy, which means that data can only be transferred between pages with the same origin unless the receiving page explicitly allows it using the
Access-Control-Allow-Origin
header.
In summary, while the Drag and Drop API is primarily designed for use within a single web application, it can be used to transfer data between different applications or windows under certain conditions, such as when the receiving application supports the same data format and cross-origin restrictions are handled appropriately.
How do you handle different types of data when using the Drag and Drop API for drag-and-drop operations?
Handling different types of data with the Drag and Drop API involves using the dataTransfer
object to set and retrieve data in various formats. Here's how you can handle different types of data:
Setting data: When initiating a drag operation, you can set multiple types of data using the
setData
method of thedataTransfer
object. For example:element.addEventListener('dragstart', (e) => { e.dataTransfer.setData('text/plain', 'Hello, World!'); e.dataTransfer.setData('text/html', '<p>Hello, World!</p>'); e.dataTransfer.setData('application/json', JSON.stringify({ message: 'Hello, World!' })); });
Copy after loginIn this example, we set three different types of data: plain text, HTML, and JSON.
Retrieving data: When handling the drop event, you can retrieve the data in the desired format using the
getData
method of thedataTransfer
object. For example:dropTarget.addEventListener('drop', (e) => { e.preventDefault(); const plainText = e.dataTransfer.getData('text/plain'); const html = e.dataTransfer.getData('text/html'); const json = e.dataTransfer.getData('application/json'); // Handle the retrieved data });
Copy after loginIn this example, we retrieve the data in three different formats: plain text, HTML, and JSON.
Handling multiple data types: You can check for the availability of different data types using the
types
property of thedataTransfer
object. For example:dropTarget.addEventListener('drop', (e) => { e.preventDefault(); const types = e.dataTransfer.types; if (types.includes('text/plain')) { const plainText = e.dataTransfer.getData('text/plain'); // Handle plain text data } if (types.includes('text/html')) { const html = e.dataTransfer.getData('text/html'); // Handle HTML data } if (types.includes('application/json')) { const json = e.dataTransfer.getData('application/json'); // Handle JSON data } });
Copy after loginIn this example, we check for the availability of different data types and handle each type accordingly.
By using the dataTransfer
object and its methods, you can handle different types of data during drag-and-drop operations with the Drag and Drop API.
The above is the detailed content of How can you use the Drag and Drop API to enable drag-and-drop functionality?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
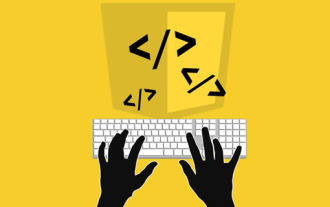
Difficulty in updating caching of official account web pages: How to avoid the old cache affecting the user experience after version update?
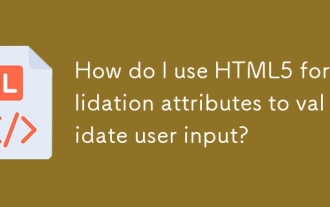
How do I use HTML5 form validation attributes to validate user input?
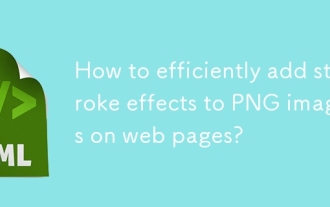
How to efficiently add stroke effects to PNG images on web pages?
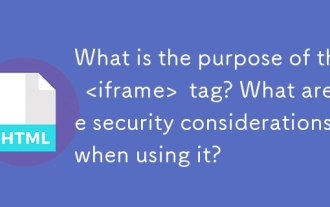
What is the purpose of the <iframe> tag? What are the security considerations when using it?
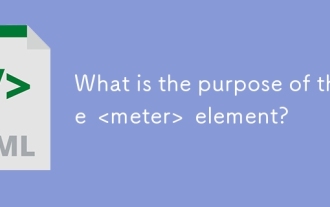
What is the purpose of the <meter> element?
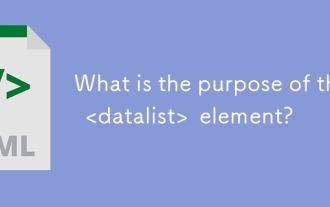
What is the purpose of the <datalist> element?
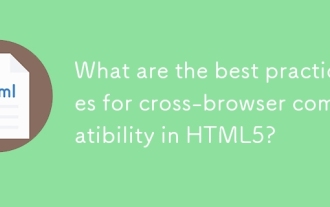
What are the best practices for cross-browser compatibility in HTML5?
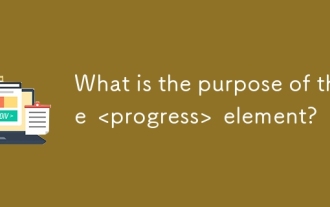
What is the purpose of the <progress> element?
