How can you implement a custom container in C ?
How can you implement a custom container in C ?
Implementing a custom container in C involves several steps and considerations to ensure it behaves like a standard container. Here's a step-by-step guide to creating a basic custom container:
-
Define the Container Class: Start by defining a class that will represent your container. This class should include member variables to store the data and member functions to manipulate the data.
template <typename T> class CustomContainer { private: std::vector<T> data; // Using std::vector as an example for simplicity public: // Constructor CustomContainer() = default; // Destructor ~CustomContainer() = default; // Member functions void push_back(const T& value) { data.push_back(value); } void pop_back() { if (!data.empty()) data.pop_back(); } T& back() { return data.back(); } const T& back() const { return data.back(); } bool empty() const { return data.empty(); } size_t size() const { return data.size(); } // Iterator support using iterator = typename std::vector<T>::iterator; using const_iterator = typename std::vector<T>::const_iterator; iterator begin() { return data.begin(); } iterator end() { return data.end(); } const_iterator begin() const { return data.begin(); } const_iterator end() const { return data.end(); } };
Copy after login - Implement Required Member Functions: Your container should support basic operations like insertion, deletion, and access. The example above uses
push_back
,pop_back
,back
,empty
, andsize
. - Support Iterators: To make your container compatible with standard algorithms, you need to provide iterator support. The example above uses
std::vector
's iterators for simplicity, but you can implement your own iterators if needed. - Implement Other Necessary Functions: Depending on your container's purpose, you might need to implement other functions like
front
,clear
,insert
,erase
, etc. - Test Your Container: Write test cases to ensure your container works as expected and is compatible with standard algorithms.
What are the key components needed to create a custom container in C ?
To create a custom container in C , you need to include several key components:
- Data Storage: This is the core of your container where the actual data is stored. It could be an array, a linked list, a tree, or any other data structure that suits your needs.
Member Functions: These are the functions that allow users to interact with the container. Common functions include:
push_back
,pop_back
,front
,back
,empty
,size
,clear
,insert
,erase
, etc.
Iterators: Iterators are crucial for making your container compatible with standard algorithms. You need to provide:
begin()
andend()
functions to return iterators to the start and end of the container.const
versions of these functions for read-only access.
- Constructors and Destructors: Proper initialization and cleanup are important for managing resources.
- Type Definitions: Define types like
value_type
,size_type
,iterator
,const_iterator
, etc., to make your container more compatible with standard algorithms and other containers. - Operator Overloading: Depending on your needs, you might want to overload operators like
[]
for direct access or=
for assignment. - Exception Handling: Implement proper exception handling to manage errors gracefully.
How do you ensure that your custom container in C is compatible with standard algorithms?
To ensure your custom container is compatible with standard algorithms, you need to follow these steps:
Implement Iterator Support: Standard algorithms rely heavily on iterators. Your container should provide
begin()
andend()
functions that return iterators to the start and end of the container. You should also provideconst
versions of these functions for read-only access.iterator begin() { return data.begin(); } iterator end() { return data.end(); } const_iterator begin() const { return data.begin(); } const_iterator end() const { return data.end(); }
Copy after loginDefine Necessary Type Aliases: Standard algorithms often use type aliases like
value_type
,size_type
,iterator
, andconst_iterator
. Define these in your container class.using value_type = T; using size_type = size_t; using iterator = typename std::vector<T>::iterator; using const_iterator = typename std::vector<T>::const_iterator;
Copy after login- Implement Required Member Functions: Some algorithms require specific member functions. For example,
std::sort
requiresbegin()
andend()
, whilestd::find
requiresbegin()
andend()
as well. Ensure your container provides these functions. - Ensure Iterator Category: Depending on the algorithms you want to support, your iterators should conform to certain categories (e.g., input iterator, output iterator, forward iterator, bidirectional iterator, random access iterator). For example,
std::sort
requires random access iterators. Test with Standard Algorithms: Write test cases using standard algorithms to ensure your container works correctly. For example:
CustomContainer<int> container; container.push_back(3); container.push_back(1); container.push_back(2); std::sort(container.begin(), container.end()); for (const auto& value : container) { std::cout << value << " "; } // Output should be: 1 2 3
Copy after login
What are the performance considerations when designing a custom container in C ?
When designing a custom container in C , performance considerations are crucial. Here are some key points to keep in mind:
- Memory Management: Efficient memory management is vital. Consider using techniques like memory pools or custom allocators to reduce overhead. For example, if your container frequently allocates and deallocates memory, using a custom allocator can improve performance.
- Cache Friendliness: Design your container to be cache-friendly. This means organizing data in a way that maximizes cache hits. For example, using contiguous memory (like an array) can improve performance due to better cache utilization.
-
Time Complexity: Analyze the time complexity of your container's operations. For example, if you're designing a vector-like container, ensure that
push_back
is amortized O(1) andat
is O(1). If you're designing a list-like container, ensure thatinsert
anderase
are O(1). -
Iterator Performance: The performance of iterators can significantly impact the performance of algorithms using your container. Ensure that your iterators are efficient and conform to the appropriate iterator category (e.g., random access iterators for better performance with algorithms like
std::sort
). - Thread Safety: If your container will be used in a multi-threaded environment, consider thread safety. This might involve using mutexes or other synchronization primitives to protect shared data.
- Copy and Move Semantics: Implement efficient copy and move constructors and assignment operators. This can significantly impact performance, especially for large containers.
- Exception Safety: Ensure your container provides strong exception safety guarantees. This means that if an operation throws an exception, the container should remain in a valid state.
- Benchmarking and Profiling: Use benchmarking and profiling tools to measure the performance of your container. This can help identify bottlenecks and areas for optimization.
By carefully considering these performance aspects, you can design a custom container that not only meets your functional requirements but also performs efficiently in real-world scenarios.
The above is the detailed content of How can you implement a custom container in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










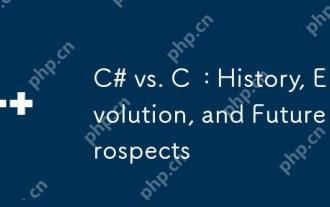
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
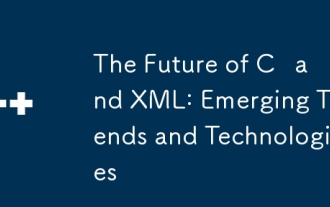
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
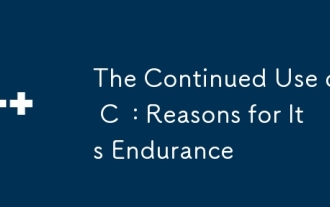
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
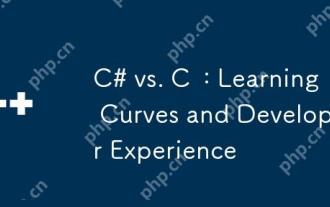
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
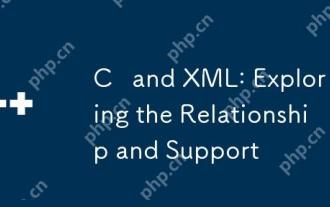
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
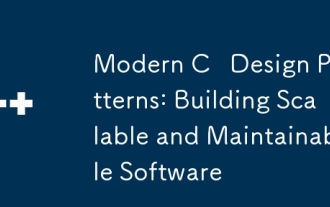
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
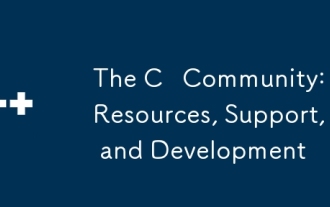
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
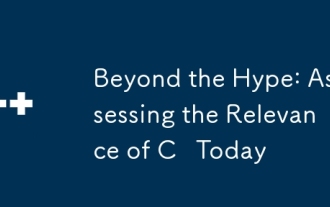
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
